Building a GraphQL-powered TODO list AI Agent in n8n (Complete tutorial)
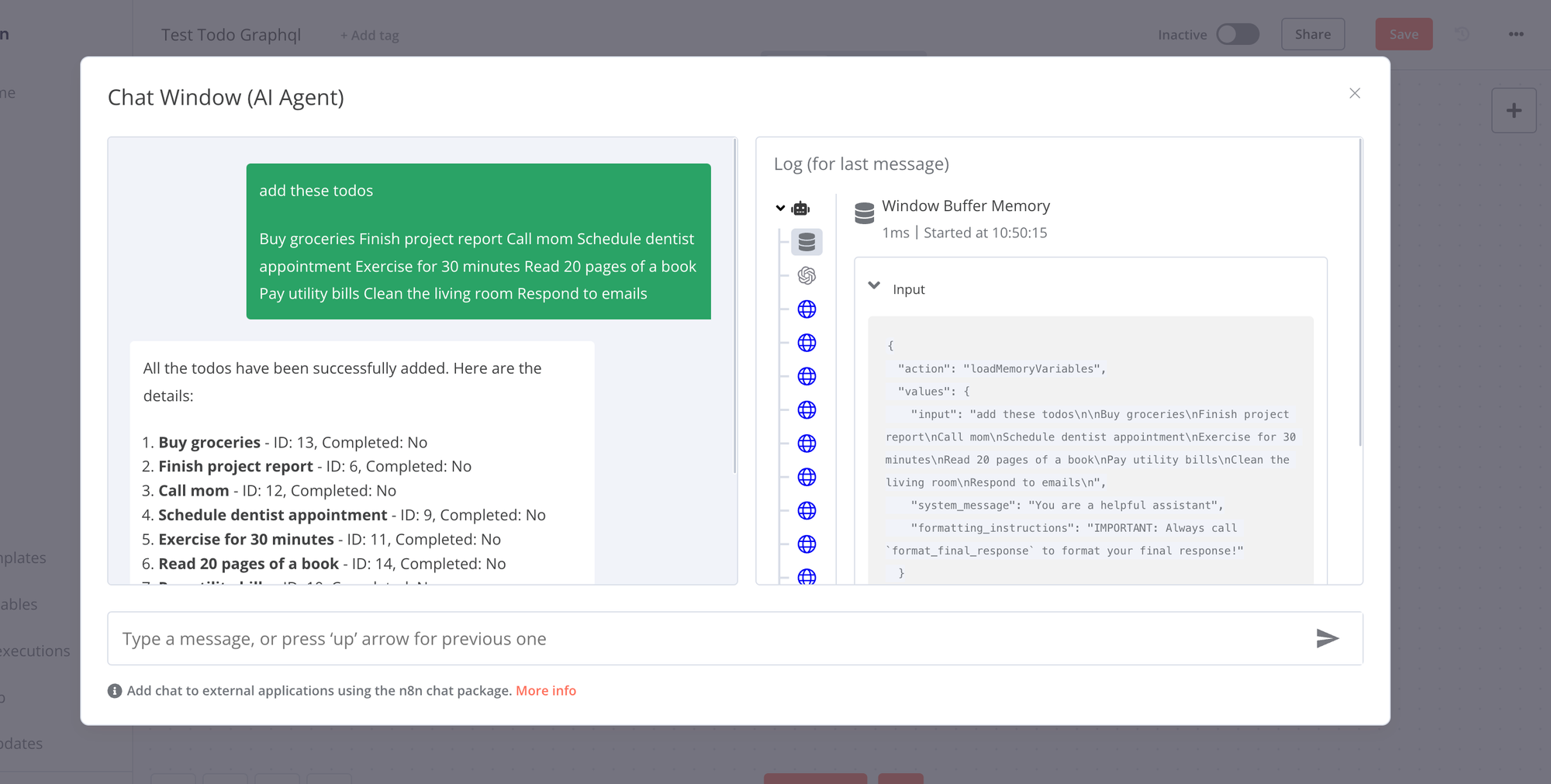
In this tutorial, we will build a GraphQL-powered todo list that you can chat with to create and analyse your todos.
We will use n8n, an open source self-hosted alternative similar to make.com, and OpenAI (gpt-4-0-mini).
But before we dive in:
Why GraphQL
If you've worked with n8n or make.com before, and used APIs in your workflow, you've usually had to add an API component to the workflow for each endpoint - for example, one for fetching todos, one for deleting, and so on.
But what if we could add the whole API in one go and expose it to an API agent that would automatically figure out the right parameters based on the user's request? Wouldn't that be great?
Of course, in a real scenario you'd need to add proper guardrails to prevent it from doing everything (at least it should be restricted to the user's data and allow rollback, etc.).
But let's see what an agent equipped with a GraphQL-powered API can do, using a todo list as an example.
#1 Build the NextJS todo list application
I built a simple Next.js todo list application using GraphQL. Here is the code that can initialise the application for you:
https://gist.github.com/deepmtch/b86990721e449e48ea3c0bd9bc4b727d
Run this script in your favourite environment (I use Docker, but you can also run it in a host environment).
The schema of the todos application is very simple:
type todo {
id: Int!
title: String!
completed: Boolean!
createdAt: String!
updatedAt: String!
}
type query {
todos: [Todo!]!
todo(id: Int!): Todo
}
type mutation {
createTodo(title: String!): Todo!
updateTodo(id: Int!, completed: Boolean!): Todo!
deleteTodo(id: Int!): Todo!
}