Update README.md
Browse files
README.md
CHANGED
@@ -24,6 +24,9 @@ The model was trained on a random collection of **English** sentences from Wikip
|
|
24 |
# Model Usage
|
25 |
### Example 1) - Sentence Similarity
|
26 |
|
|
|
|
|
|
|
27 |
```python
|
28 |
from transformers import AutoTokenizer, AutoModel
|
29 |
import torch.nn as nn
|
@@ -69,8 +72,13 @@ cos_sim = sim(embeddings.unsqueeze(1),
|
|
69 |
print(f"Distance: {cos_sim[0,1].detach().item()}")
|
70 |
```
|
71 |
|
|
|
|
|
72 |
### Example 2) - Clustering
|
73 |
|
|
|
|
|
|
|
74 |
```python
|
75 |
from transformers import AutoTokenizer, AutoModel
|
76 |
import torch.nn as nn
|
@@ -146,9 +154,13 @@ umap_plot.points(umap_model, labels = np.array(classes),theme='fire')
|
|
146 |
|
147 |
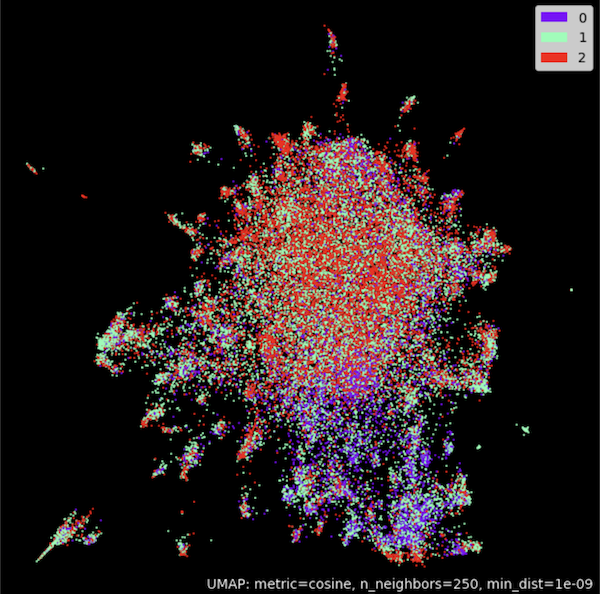
|
148 |
|
|
|
149 |
|
150 |
### Example 3) - Using [SentenceTransformers](https://www.sbert.net/)
|
151 |
|
|
|
|
|
|
|
152 |
```python
|
153 |
from sentence_transformers import SentenceTransformer, util
|
154 |
from sentence_transformers import models
|
@@ -182,6 +194,7 @@ for i in range(len(sentences1)):
|
|
182 |
print(f"Similarity {cosine_scores[i][i]:.2f}: {sentences1[i]} << vs. >> {sentences2[i]}")
|
183 |
```
|
184 |
|
|
|
185 |
|
186 |
# Benchmark
|
187 |
|
|
|
24 |
# Model Usage
|
25 |
### Example 1) - Sentence Similarity
|
26 |
|
27 |
+
<details>
|
28 |
+
<summary> Click to expand </summary>
|
29 |
+
|
30 |
```python
|
31 |
from transformers import AutoTokenizer, AutoModel
|
32 |
import torch.nn as nn
|
|
|
72 |
print(f"Distance: {cos_sim[0,1].detach().item()}")
|
73 |
```
|
74 |
|
75 |
+
</details>
|
76 |
+
|
77 |
### Example 2) - Clustering
|
78 |
|
79 |
+
<details>
|
80 |
+
<summary> Click to expand </summary>
|
81 |
+
|
82 |
```python
|
83 |
from transformers import AutoTokenizer, AutoModel
|
84 |
import torch.nn as nn
|
|
|
154 |
|
155 |
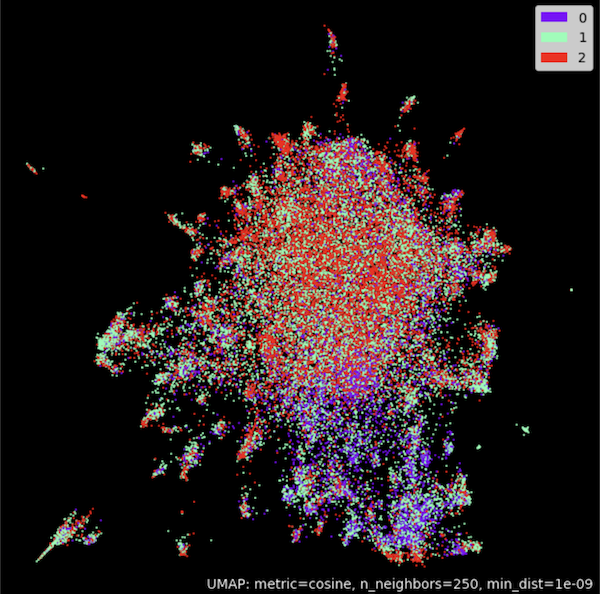
|
156 |
|
157 |
+
</details>
|
158 |
|
159 |
### Example 3) - Using [SentenceTransformers](https://www.sbert.net/)
|
160 |
|
161 |
+
<details>
|
162 |
+
<summary> Click to expand </summary>
|
163 |
+
|
164 |
```python
|
165 |
from sentence_transformers import SentenceTransformer, util
|
166 |
from sentence_transformers import models
|
|
|
194 |
print(f"Similarity {cosine_scores[i][i]:.2f}: {sentences1[i]} << vs. >> {sentences2[i]}")
|
195 |
```
|
196 |
|
197 |
+
</details>
|
198 |
|
199 |
# Benchmark
|
200 |
|