Spaces:
Runtime error
Runtime error
#!/usr/bin/env python | |
# coding: utf-8 | |
# In[113]: | |
import gradio as gr | |
import torch | |
from scipy.special import softmax | |
from transformers import BertForSequenceClassification, BertTokenizer, BertConfig | |
# In[114]: | |
tokenizer = BertTokenizer.from_pretrained("indobenchmark/indobert-base-p1") | |
config = BertConfig.from_pretrained('indobenchmark/indobert-base-p1') | |
model = BertForSequenceClassification.from_pretrained('indobenchmark/indobert-base-p1', config = config) | |
# In[115]: | |
def preprocess(text): | |
new_text = [] | |
for t in text.split(" "): | |
t = '@user' if t.startswith('@') and len(t) > 1 else t | |
t = 'http' if t.startswith('http') else t | |
new_text.append(t) | |
return " ".join(new_text) | |
# In[116]: | |
def sentiment_analysis(text): | |
text = preprocess(text) | |
encoded_input = tokenizer(text, return_tensors='pt') | |
output = model(**encoded_input) | |
scores_ = output[0][0].detach().numpy() | |
scores_ = softmax(scores_) | |
# Format output dict of scores | |
labels = ['Negative', 'Neutral', 'Positive'] | |
scores = {l:float(s) for (l,s) in zip(labels, scores_) } | |
return scores | |
# In[117]: | |
example_sentence_1 = "aduh mahasiswa sombong kasih kartu kuning belajar usahlah politik selesai kuliah nya politik telat dasar mahasiswa" | |
example_sentence_2 = "lokasi strategis jalan sumatra bandung nya nyaman sofa lantai paella nya enak pas dimakan minum bir dingin appetiser nya enak enak" | |
example_sentence_3 = "pakai kartu kredit bca tidak untung malah rugi besar" | |
example_sentence_4 = "makanan beragam , harga makanan di food stall akan ditambahkan 10 % lagi di kasir , suasana ramai dan perlu perhatian untuk mendapatkan parkir dan tempat duduk" | |
examples = [[example_sentence_1], [example_sentence_2], | |
[example_sentence_3], [example_sentence_4]] | |
# In[118]: | |
markdownn = ''' | |
## Sentiment Analysis IndoBERT by IndoNLU | |
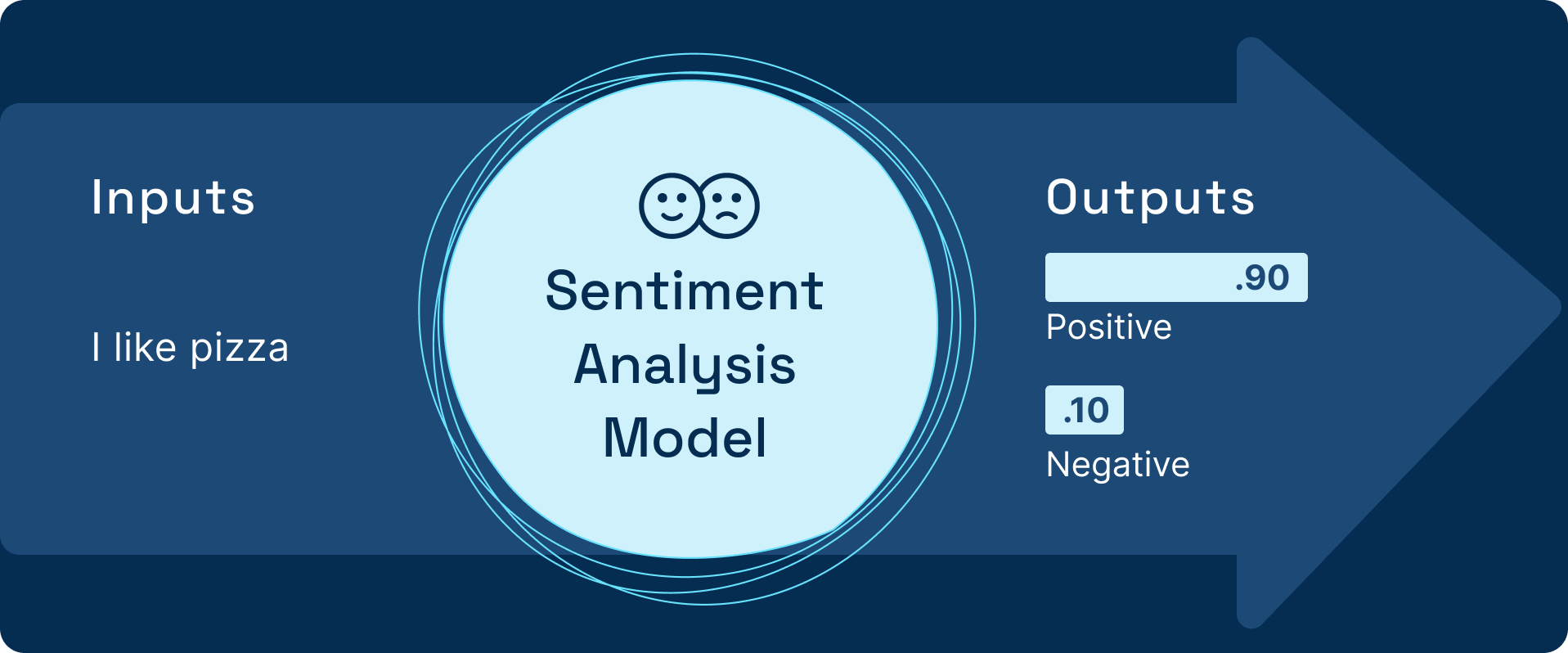 | |
### Apa itu Sentimen Analisis? | |
Sentimen analisis atau sentiment analysis adalah proses untuk mengidentifikasi, mengekstrak, | |
dan memproses sentimen atau opini dari suatu teks, seperti artikel, review, tweet, atau status media sosial lainnya. | |
Tujuannya adalah untuk menentukan apakah sentimen yang diekspresikan dalam teks tersebut adalah positif, negatif, atau netral. | |
Sentimen analisis sering digunakan dalam berbagai industri, seperti bisnis, pemasaran, media sosial, dan politik untuk memahami opini atau persepsi publik tentang produk, merek, acara, atau isu tertentu. | |
### Tujuan Penggunaan | |
Analisis sentimen adalah teknik yang dapat digunakan dalam berbagai aplikasi. Berikut adalah beberapa contoh kasus penggunaan untuk analisis sentimen. Pemantauan media sosial: Analisis sentimen dapat digunakan untuk memantau sentimen postingan media sosial tentang perusahaan atau merek. Teknik dapat membantu perusahaan memahami bagaimana merek mereka dirasakan oleh publik dan mengidentifikasi area untuk perbaikan. | |
Secara keseluruhan, analisis sentimen adalah teknik serbaguna yang dapat digunakan di berbagai bidang untuk mendapatkan wawasan dari data tekstual. | |
#### Dokumentasi Pipeline | |
berikut adalah dokumentasi Deep Learning indoBERT sentimen analisis: | |
```python | |
from deepsparse import Pipeline | |
tokenizer = BertTokenizer.from_pretrained("indobenchmark/indobert-base-p1") | |
config = BertConfig.from_pretrained('indobenchmark/indobert-base-p1') | |
model = BertForSequenceClassification.from_pretrained('indobenchmark/indobert-base-p1') | |
def preprocess(text): | |
new_text = [] | |
for t in text.split(" "): | |
t = '@user' if t.startswith('@') and len(t) > 1 else t | |
t = 'http' if t.startswith('http') else t | |
new_text.append(t) | |
return " ".join(new_text) | |
def sentiment_analysis(text): | |
text = preprocess(text) | |
encoded_input = tokenizer(text, return_tensors='pt') | |
output = model(**encoded_input) | |
scores_ = output[0][0].detach().numpy() | |
scores_ = softmax(scores_) | |
# Format output dict of scores | |
labels = ['Negative', 'Neutral', 'Positive'] | |
scores = {l:float(s) for (l,s) in zip(labels, scores_) } | |
return scores | |
``` | |
''' | |
# In[119]: | |
with gr.Blocks() as demo: | |
with gr.Row(): | |
with gr.Column(): | |
gr.Markdown(markdownn) | |
with gr.Column(): | |
gr.Markdown(""" | |
### Sentiment analysis demo | |
by [Created by @Vrooh933 Production](https://www.linkedin.com/in/m-afif-rizky-a-a96048182) | |
""") | |
text = gr.Text(label="Text") | |
btn = gr.Button("Submit") | |
sparse_answers = gr.Label(label="label") | |
gr.Examples([[example_sentence_1], [example_sentence_2], | |
[example_sentence_3], [example_sentence_4]], inputs = [text]) | |
btn.click( | |
sentiment_analysis, | |
inputs=[text], | |
outputs=[sparse_answers], | |
api_name="addition" | |
) | |
if __name__ == "__main__": | |
demo.launch() | |
# In[ ]: | |