'+s1.split('
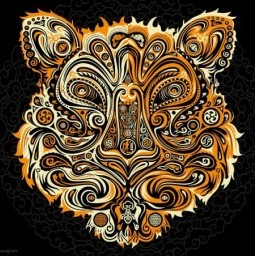
'
return s2
def fx_datatable(s:str):
a=exec(s)
return {k: v for k, v in locals().items() if isinstance(v,pd.DataFrame)}
def fx_dd(tk:str,s:str):
headers= {"Content-Type": "application/json"}
url="https://oapi.dingtalk.com/robot/send?access_token="+tk
data={'msgtype':'text','text':{'title': '吹牛逼',"content": s}, 'at': {'atMobiles': [], 'isAtAll': True}}
response=requests.post(url,json=data,headers=headers)
return response.text
def dd_ocr(tk,sl,dt):
headers= {"Content-Type": "application/json"}
url="https://oapi.dingtalk.com/topapi/ocr/structured/recognize?access_token="+tk
dc={"身份证":"idcard","增值税发票":"invoice","营业执照":"blicense","银行卡":"bank_card","车牌":"car_no","机动车发票":"car_invoice","驾驶证":"driving_license","行驶证":"vehicle_license","火车票":"train_ticket","定额发票":"quota_invoice","出租车发票":"taxi_ticket","机票行程单":"air_itinerary","审批表单":"approval_table","花名册":"roster"}
data={"image_url":sl,"type":dc[dt]}
response=requests.post(url,json=data,headers=headers)
return response.json()
demo=gr.Blocks()
with demo:
with gr.Tabs():
with gr.TabItem("测试1"):
with gr.Column():
text_input=gr.Textbox(placeholder='请输入测试字符串',label="请输入需要MD5加密的测试内容")
text_output=gr.Textbox(label="输出",visible=False)
text_input.change(fn=lambda visible: gr.update(visible=True), inputs=text_input, outputs=text_output)
bb_button=gr.Button("运行")
bb_button.click(fx, inputs=text_input, outputs=text_output,api_name='md5')
with gr.Column():
gr.Markdown("# TTS文本字符串转语音合成训练")
TTS_input=gr.Textbox(label="输入文本",default="你好吗?我很好。")
TTS_button=gr.Button("合成")
TTS_button.click(inference, inputs=TTS_input, outputs=gr.Audio(label="输出合成结果"),api_name='tts')
with gr.TabItem("M-Formatter"):
gr.Markdown("# PowerQuery M语言脚本格式化测试")
M_input=gr.Textbox(label="请填写需要格式化的M脚本",default="let a=1,b=2 in a+b",lines=18)
M_output=gr.Textbox(label="格式化结果",lines=50)
M_button=gr.Button("开始格式化>>")
M_button.click(fx_m, inputs=M_input, outputs=M_output,api_name='M')
with gr.TabItem("DAX-Formatter"):
gr.Markdown("# DAX表达式格式化测试")
with gr.Row():
DAX_input=gr.Textbox(label="请填写需要格式化的DAX表达式",default="扯淡=CALCULATE(VALUES('价格表'[单价]),FILTER('价格表','价格表'[产品]='销售表'[产品]))",lines=28)
DAX_button=gr.Button("格式化>>")
DAX_output=gr.HTML(label="DAX表达式格式化结果")
DAX_button.click(fx_dax, inputs=DAX_input, outputs=DAX_output,api_name='DAX')
with gr.TabItem("Python-Execute"):
gr.Markdown("# Python脚本测试")
d_input=gr.Textbox(label="请填写需要datatable库处理的脚本",lines=18)
d_output=gr.JSON(label="输出>")
d_button=gr.Button("开始编译>>")
d_button.click(fx_datatable, inputs=d_input, outputs=d_output,api_name='datatable')
with gr.TabItem("钉钉群消息推送"):
gr.Markdown("# 推送测试")
dd_input=[gr.Textbox(label="请填写机器人token"),gr.Textbox(label="请填写需要推送的信息",lines=10)]
dd_output=gr.Textbox(label="推送提示")
dd_button=gr.Button("提交")
dd_button.click(fx_dd, inputs=dd_input, outputs=dd_output,api_name='dingding_robot')
with gr.TabItem("钉钉ocr"):
gr.Markdown("# 网络图片OCR识别")
ocr_input=[gr.Textbox(label="请填写ocr_token"),gr.Textbox(label="请填写图片网址"),gr.Radio(["身份证","增值税发票","营业执照","银行卡","车牌","机动车发票","驾驶证","行驶证","火车票","定额发票","出租车发票","机票行程单","审批表单","花名册"],value="营业执照增值税发票",label="请选择识别类型:")]
ocr_button=gr.Button("开始识别>>")
ocr_output=gr.JSON(label="识别结果")
ocr_button.click(dd_ocr, inputs=ocr_input, outputs=ocr_output,api_name='dingding_ocr')
demo.launch()