import streamlit as st import pandas as pd import warnings import pandas as pd import plotly.express as px import plotly.graph_objects as go dataframe = pd.read_csv("life_expectancy.csv") dateframe2015 = dataframe[dataframe["Year"] == 2015] # page configs st.set_page_config( page_title="Global Life Expectancy", page_icon="π", ) # component for visualization def vis_component(hypothesis, figure, description): # no hypothesis we only show plot and description if hypothesis == "": st.plotly_chart(figure, use_container_width=True) st.write(description) # otherwise show the entire component else: st.header(hypothesis) st.plotly_chart(figure, use_container_width=True) st.write(description) # Page 1: Introduction def page_introduction(): st.title("Global Life Expectancy π") st.write("Team Name: Toasty Tangerines π") st.write("William Dawley") st.write("Ella Schutz") # introduction goes here st.write( "We employed a Kaggle dataset to develop a website presenting insights into global life expectancy factors. This dataset aids in predicting and identifying worldwide trends, facilitating efforts to mitigate diseases and enhance both the duration and quality of life." ) st.header("Our Data") st.markdown( "[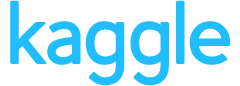](https://www.kaggle.com/datasets/amirhosseinmirzaie/countries-life-expectancy)" ) st.write("Head") head_rows = st.slider("number of rows", min_value=1, max_value=10, value=5, key="head") st.write(dataframe.head(head_rows)) st.write("Tail") tail_rows = st.slider("number of rows", min_value=1, max_value=10, value=5, key="tail") st.write(dataframe.tail(tail_rows)) st.header("Summary Statistics") st.write(dataframe.describe(include="all")) st.header("Data Cleaning and Preparation") st.write( "To ensure the accuracy of our findings, we took a careful approach to analyzing the data. We noticed that some columns had missing data, so we decided to remove the null values. This helped us avoid any potential inaccuracies caused by incomplete information. We then chose to focus our analysis on the columns where we had the most confidence. By doing so, we were able to construct graphs that were based on reliable data. " ) # Page 2: Average Life Expectancy by Country Status def page_avg_life_expectancy(): dataframe = pd.read_csv("life_expectancy.csv") dateframe2015 = dataframe[dataframe["Year"] == 2015] dataframe.dropna(inplace=True) dataframe.reset_index(drop=True, inplace=True) fig = px.bar( dateframe2015.groupby('Status')['Life expectancy'].mean().reset_index(), x='Status', y='Life expectancy', labels={'Life expectancy': 'Average Life Expectancy'}, title='Average Life Expectancy by Country Status') vis_component( "Hypothesis #1: How does developed countries and developing countries affect the average life expectancy?", fig, "Developed countries have a higher average life expectancy than developing countries. Developed countries average life expectancy is around 80 years while developing countries average life expectancy is around 70 years. This is about a ten-year difference between developed and developing countries." ) # Page 3: Top 5 Average Life Expectancy by Country def countries_life_exp(): page_top_countries() page_bottom_countries() def page_top_countries(): countries = ['Slovenia', 'Denmark', 'Cyprus', 'Chile', 'Japan'] life_expectancy = [] for country in countries: avg_life_expectancy = dateframe2015[dateframe2015['Country'] == country]['Life expectancy'].mean() life_expectancy.append(avg_life_expectancy) fig = go.Figure(data=go.Bar(x=countries, y=life_expectancy)) fig.update_layout(xaxis_title='Countries', yaxis_title='Average Life Expectancy', title='Top 5 Average Life Expectancy by Country') vis_component( "Hypothesis #2: What countries have the highest and lowest life expectancies?", fig, """The bar graph displays the countries with the highest and lowest average life expectancies. Among the countries with the highest average life expectancy, ranging between 80 to 90, are Slovenia, Denmark, Cyprus, Chile, and Japan. These countries potentially benefit from superior healthcare systems and improved living conditions. """) # Page 4: Bottom 5 Average Life Expectancy by Country def page_bottom_countries(): countries2 = [ 'Lesotho', "CΓ΄te d'Ivoire", 'Chad', 'Central African Republic', 'Angola' ] life_expectancy = [] for country in countries2: avg_life_expectancy = dateframe2015[dateframe2015['Country'] == country]['Life expectancy'].mean() life_expectancy.append(avg_life_expectancy) fig = go.Figure(data=go.Bar(x=countries2, y=life_expectancy)) fig.update_layout(xaxis_title='Countries', yaxis_title='Average Life Expectancy', title='Bottom 5 Average Life Expectancy by Country') vis_component( "", fig, """On the other hand, the graph also highlights the countries with the lowest average life expectancy, ranging between 50 to 55. These countries include Lesotho, Cote d'Ivoire, Chad, Central African Republic, and Angola. These countries may face challenges in healthcare accessibility and quality, as well as socioeconomic factors that impact overall well-being. The graph provides valuable insights into global disparities in life expectancy, emphasizing the importance of addressing healthcare and socio-economic inequalities to improve overall population health.""" ) # Page 5: Average Life Expectancy Over Time def page_life_expectancy_over_time(): avg_life_expectancy = dataframe.groupby( 'Year')['Life expectancy'].mean().reset_index() fig = go.Figure(data=go.Scatter(x=avg_life_expectancy['Year'], y=avg_life_expectancy['Life expectancy'], mode='lines+markers')) fig.update_layout(xaxis_title='Year', yaxis_title='Life Expectancy', title='Average Life Expectancy Over Time') vis_component( "Hypothesis #3: How Does the Global Life Expectancy Change Over Time", fig, """The graph depicts the average life expectancy from 2000 to 2015, demonstrating a consistent and overall upward trend. In most years, the average life expectancy shows a gradual increase, indicating positive advancements in healthcare, living conditions, and societal well-being. However, a notable exception occurs between 2001 and 2003 when the average life expectancy experiences a temporary decline of approximately two years. Despite this brief deviation, the graph reflects a generally linear progression, suggesting ongoing improvements in global health outcomes. The sustained upward trajectory signifies the collective efforts in healthcare advancements, disease prevention, and social development that contribute to prolonged life expectancy worldwide.""" ) def vari_comp(hypothesis, plots, descriptions): if hypothesis == "": for i in range(len(plots)): st.plotly_chart(plots[i], use_container_width=True) st.write(descriptions[i]) # otherwise show the entire component else: st.header(hypothesis) for i in range(len(plots)): st.plotly_chart(plots[i], use_container_width=True) st.write(descriptions[i]) # Page 6: Average Variables Over Time def page_variables_over_time(): avg_life_expectancy = dataframe.groupby( 'Year')['Life expectancy'].mean().reset_index() # Selecting the desired variables for the y-axis y_variables = ['Measles', 'Polio', 'Diphtheria'] plots = [] descriptions = [ """This graph presents the average incidence rate of reported measles cases per 1000 individuals worldwide from 2000 to 2015. It demonstrates a predominantly positive trend, wherein the number of measles cases generally rises over time. This observation underscores the concerning global pattern of increasing measles infections as the years progress.""", """This graph illustrates the average percentage of one-year-olds who received polio immunization worldwide from 2000 to 2015. The data reveals an initial positive trend, indicating a rising rate of polio immunization among one-year-olds from 2000 to approximately 2003. However, starting from 2004, a negative trend emerges, leading to a gradual decline in the immunization percentage until 2015. This observation highlights the need for further investigation and efforts to understand and address the factors contributing to the decreasing polio immunization rates during this period.""", """This graph presents the average percentage of one-year-olds who received diphtheria immunization worldwide from 2000 to 2015. The data reveals a discernible negative trend in the immunization percentage over the specified period. Interestingly, the observed trend closely mirrors the pattern observed in the previous graph depicting the average polio immunization over time. These findings underscore the importance of closely examining the factors contributing to the declining trend in diphtheria immunization rates, which parallel the trends observed in polio immunization. Further research and targeted interventions are warranted to address and mitigate these concerning trends in childhood immunization.""" ] # Looping over each variable and creating a separate plot for each for variable in y_variables: # Create a separate figure for each variable fig = go.Figure(data=go.Scatter(x=avg_life_expectancy['Year'], y=dataframe[variable], mode='lines+markers')) # Customizing the graph for each variable fig.update_layout(xaxis_title='Year', yaxis_title=variable, title=f'Average {variable} Over Time') plots.append(fig) # Displaying the graph for each variable vari_comp("Hypothesis 4#: How Do Diseases Change Over Time", plots, descriptions) def page_variables_over_time3(): avg_life_expectancy = dataframe.groupby( 'Year')['Life expectancy'].mean().reset_index() # Selecting the desired variables for the y-axis y_variables = ['BMI', 'thinness 1-19 years'] plots = [] descriptions = [ """This graph shows the average body mass index (BMI) from 2000 to 2015, revealing a consistent negative trend over time. This highlights the importance of addressing factors related to body weight and overall health. Understanding the underlying influences on weight management, such as lifestyle choices and socio-environmental factors, is crucial. By promoting balanced nutrition, physical activity, and supportive environments, we can improve health outcomes and reduce the risks of obesity-related diseases. Continued research and monitoring are essential to evaluate interventions and develop evidence-based strategies for population-wide health promotion and disease prevention.""", """This graph illustrates the average prevalence of thinness in 19-year-olds from 2000 to 2015. A positive trend is observed from 2000 to 2013, followed by a significant drop in prevalence between 2013 and 2014, and then a resumption of the upward trend. These patterns highlight the importance of addressing factors contributing to thinness in young adults, understanding underlying determinants, and conducting further research to investigate the anomaly. The findings underscore the need for comprehensive interventions promoting healthy body composition and emphasizing balanced nutrition and lifestyle behaviors for optimal health outcomes among this age group.""" ] # Looping over each variable and creating a separate plot for each for variable in y_variables: # Create a separate figure for each variable fig = go.Figure(data=go.Scatter(x=avg_life_expectancy['Year'], y=dataframe[variable], mode='lines+markers')) # Customizing the graph for each variable fig.update_layout(xaxis_title='Year', yaxis_title=variable, title=f'Average {variable} Over Time') # Displaying the graph for each variable plots.append(fig) vari_comp( "Hypothesis #5: Is there a correlation between the average BMI and the average prevalence of thinness over time?", plots, descriptions) def page_variables_over_time2(): avg_life_expectancy = dataframe.groupby( 'Year')['Life expectancy'].mean().reset_index() # Selecting the desired variables for the y-axis y_variables = ['infant deaths', 'under-five deaths'] plots = [] descriptions = [ """This graph reveals a consistent linear increase in the average number of infant deaths per year from 2000 to 2011, followed by a subsequent period of stabilization or decline from 2011 to 2015. Understanding and addressing factors affecting infant mortality rates, including healthcare access, socioeconomic conditions, and effective interventions, is crucial. The observed trends may indicate improvements in healthcare systems and successful interventions aimed at reducing infant mortality. Ongoing efforts to enhance maternal and child healthcare, implement evidence-based interventions, and address societal factors can contribute to healthier outcomes for infants and the prevention of avoidable infant deaths.""", """This graph shows the average number of deaths among children under five years old from 2000 to 2015. It exhibits a positive trend with a linear increase between 2000 and 2012, followed by a stabilization or decline in the number of deaths. These findings highlight the importance of addressing factors related to child mortality, including healthcare access, nutrition, and disease prevention. Further research is needed to understand the factors driving these trends and implement targeted interventions to improve child well-being and survival globally.""" ] # Looping over each variable and creating a separate plot for each for variable in y_variables: # Create a separate figure for each variable fig = go.Figure(data=go.Scatter(x=avg_life_expectancy['Year'], y=dataframe[variable], mode='lines+markers')) # Customizing the graph for each variable fig.update_layout(xaxis_title='Year', yaxis_title=variable, title=f'Average {variable} Over Time') plots.append(fig) # Displaying the graph for each variable vari_comp( "Hypothesis #6: How does the deaths of young children change over time? ", plots, descriptions) def conclusion(): st.title("Thank you for going through our page!") st.write("") st.markdown( """
""", unsafe_allow_html=True) # Main app def main(): pages = { "Introduction": page_introduction, "Average Life Expectancy by Status": page_avg_life_expectancy, "Average Life Expectancy by Country": countries_life_exp, "Average Life Expectancy Over Time": page_life_expectancy_over_time, "Mortality Over Time": page_variables_over_time2, "Diseases Over Time": page_variables_over_time, "Average Health Over Time": page_variables_over_time3, "Conclusion": conclusion } st.sidebar.title("Navigation") page = st.sidebar.selectbox("Select a page", tuple(pages.keys())) # Execute the selected page function pages[page]() if __name__ == "__main__": main()