Spaces:
Sleeping
Sleeping
Upload app3.py
Browse files
app3.py
ADDED
@@ -0,0 +1,114 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import streamlit as st
|
2 |
+
import pandas as pd
|
3 |
+
import joblib
|
4 |
+
from preprocessText import preprocess
|
5 |
+
from apiSearch import get_metadata
|
6 |
+
import base64
|
7 |
+
import requests
|
8 |
+
# Load the model
|
9 |
+
model = joblib.load('85pct.pkl')
|
10 |
+
|
11 |
+
# Define the categories
|
12 |
+
categories = {
|
13 |
+
'Film & Animation': 1,
|
14 |
+
'Autos & Vehicles': 2,
|
15 |
+
'Music': 10,
|
16 |
+
'Pets & Animals': 15,
|
17 |
+
'Sports' : 17,
|
18 |
+
'Short Movies' : 18,
|
19 |
+
'Travel & Events' : 19,
|
20 |
+
'Gaming' : 20,
|
21 |
+
'Videoblogging' : 21,
|
22 |
+
'People & Blogs' : 22,
|
23 |
+
'Comedy' : 23,
|
24 |
+
'Entertainment' : 24,
|
25 |
+
'News & Politics' : 25,
|
26 |
+
'Howto & Style' : 26,
|
27 |
+
'Education' : 27,
|
28 |
+
'Science & Technology' : 28,
|
29 |
+
'Nonprofits & Activism' : 29
|
30 |
+
}
|
31 |
+
|
32 |
+
# Create the Streamlit web application
|
33 |
+
def main():
|
34 |
+
st.set_page_config(layout="wide")
|
35 |
+
st.title("YouTube Trend Prediction")
|
36 |
+
st.write("Enter the video details below:")
|
37 |
+
|
38 |
+
# Input fields
|
39 |
+
col1, col2, col3 = st.columns(3)
|
40 |
+
getTitle, getDuration, getCategory = "", 0.00, 1
|
41 |
+
getThumbnailUrl = ""
|
42 |
+
with col1:
|
43 |
+
url = st.text_input("URL")
|
44 |
+
if url:
|
45 |
+
metadata = get_metadata(url)
|
46 |
+
if not metadata.empty:
|
47 |
+
getTitle = metadata['title'].iloc[0]
|
48 |
+
getDuration = metadata['duration'].iloc[0]
|
49 |
+
category_id = metadata['category_id'].iloc[0]
|
50 |
+
getThumbnailUrl = metadata['thumbnail_link'].iloc[0]
|
51 |
+
getCategory = int(category_id)
|
52 |
+
if getThumbnailUrl is not None:
|
53 |
+
picture = get_picture_from_url(getThumbnailUrl)
|
54 |
+
if picture:
|
55 |
+
st.image(picture, caption='Uploaded Picture', use_column_width=True)
|
56 |
+
with col2:
|
57 |
+
title = st.text_input("Title", value=getTitle)
|
58 |
+
duration = st.number_input("Duration (in minutes)", min_value=0.0, value=getDuration)
|
59 |
+
category = st.selectbox("Category", list(categories.keys()), index=list(categories.values()).index(getCategory))
|
60 |
+
|
61 |
+
with col3:
|
62 |
+
picture = st.file_uploader("Upload Picture", type=["jpg", "jpeg", "png"])
|
63 |
+
if picture is not None:
|
64 |
+
st.picture(picture, use_column_width=True)
|
65 |
+
# Convert category to category ID
|
66 |
+
categoryId = categories[category]
|
67 |
+
|
68 |
+
# Predict button
|
69 |
+
if st.button("Predict"):
|
70 |
+
# Perform prediction
|
71 |
+
prediction = predict_trend(title, duration, categoryId)
|
72 |
+
if prediction[0] == 1:
|
73 |
+
st.success("This video is predicted to be a trend!")
|
74 |
+
st.markdown("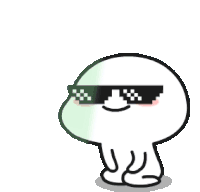")
|
75 |
+
else:
|
76 |
+
st.info("This video is predicted not to be a trend.")
|
77 |
+
st.markdown("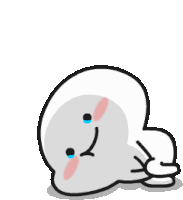")
|
78 |
+
|
79 |
+
|
80 |
+
|
81 |
+
|
82 |
+
|
83 |
+
def get_picture_from_url(url):
|
84 |
+
try:
|
85 |
+
response = requests.get(url)
|
86 |
+
image_data = response.content
|
87 |
+
return image_data
|
88 |
+
except:
|
89 |
+
return None
|
90 |
+
|
91 |
+
# Function to make predictions
|
92 |
+
def predict_trend(title, duration, category_id):
|
93 |
+
duration = str(duration)
|
94 |
+
category_id = str(category_id)
|
95 |
+
clean_new_title = preprocess(title)
|
96 |
+
# Join the preprocessed words back into a string
|
97 |
+
clean_new_title_str = ' '.join(clean_new_title)
|
98 |
+
# Prepare the input data
|
99 |
+
data = {
|
100 |
+
'cleanTitle': [clean_new_title_str],
|
101 |
+
'titleLength' : [len(title)],
|
102 |
+
'categoryId': [category_id],
|
103 |
+
'duration': [duration]
|
104 |
+
}
|
105 |
+
data = pd.DataFrame(data)
|
106 |
+
data['categoryId'] = data['categoryId'].astype('category')
|
107 |
+
data['duration'] = data['duration'].astype('float64')
|
108 |
+
# Make the prediction
|
109 |
+
print(model.predict_proba(data))
|
110 |
+
prediction = model.predict(data)
|
111 |
+
return prediction
|
112 |
+
|
113 |
+
if __name__ == "__main__":
|
114 |
+
main()
|