Search is not available for this dataset
id
stringlengths 1
8
| text
stringlengths 72
9.81M
| addition_count
int64 0
10k
| commit_subject
stringlengths 0
3.7k
| deletion_count
int64 0
8.43k
| file_extension
stringlengths 0
32
| lang
stringlengths 1
94
| license
stringclasses 10
values | repo_name
stringlengths 9
59
|
---|---|---|---|---|---|---|---|---|
800 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
});
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Allow constructors with more than 1 parameter to be mocked
<DFF> @@ -491,6 +491,14 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
});
});
+ t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
+ awsMock.setSDK('aws-sdk');
+ awsMock.mock('CloudFront.Signer', 'getSignedUrl');
+ var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
+ st.ok(signer);
+ st.end();
+ });
+
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
| 8 | Allow constructors with more than 1 parameter to be mocked | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
801 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
});
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Allow constructors with more than 1 parameter to be mocked
<DFF> @@ -491,6 +491,14 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
});
});
+ t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
+ awsMock.setSDK('aws-sdk');
+ awsMock.mock('CloudFront.Signer', 'getSignedUrl');
+ var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
+ st.ok(signer);
+ st.end();
+ });
+
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
| 8 | Allow constructors with more than 1 parameter to be mocked | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
802 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import AWSMock from 'aws-sdk-mock';
import AWS from 'aws-sdk';
import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
### Configuring promises
If your environment lacks a global Promise contstructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #204 from r1b/master
fix: typo in README.md
<DFF> @@ -270,7 +270,7 @@ const sqs = new AWS.SQS();
### Configuring promises
-If your environment lacks a global Promise contstructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
+If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
| 1 | Merge pull request #204 from r1b/master | 1 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
803 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import AWSMock from 'aws-sdk-mock';
import AWS from 'aws-sdk';
import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
### Configuring promises
If your environment lacks a global Promise contstructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #204 from r1b/master
fix: typo in README.md
<DFF> @@ -270,7 +270,7 @@ const sqs = new AWS.SQS();
### Configuring promises
-If your environment lacks a global Promise contstructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
+If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
| 1 | Merge pull request #204 from r1b/master | 1 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
804 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
console.log(e);
}
});
t.end();
});
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Fix chained calls usage with mocked methods
<DFF> @@ -493,6 +493,14 @@ test('AWS.mock function should mock AWS service and method on the service', func
console.log(e);
}
});
+
+ t.test('Mocked service should allow chained calls after listening to events', function (st) {
+ awsMock.mock('S3', 'getObject');
+ const s3 = new AWS.S3();
+ const req = s3.getObject({Bucket: 'b', notKey: 'k'});
+ st.equals(req.on('httpHeaders', ()=>{}), req);
+ st.end();
+ });
t.end();
});
| 8 | Fix chained calls usage with mocked methods | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
805 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
console.log(e);
}
});
t.end();
});
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Fix chained calls usage with mocked methods
<DFF> @@ -493,6 +493,14 @@ test('AWS.mock function should mock AWS service and method on the service', func
console.log(e);
}
});
+
+ t.test('Mocked service should allow chained calls after listening to events', function (st) {
+ awsMock.mock('S3', 'getObject');
+ const s3 = new AWS.S3();
+ const req = s3.getObject({Bucket: 'b', notKey: 'k'});
+ st.equals(req.on('httpHeaders', ()=>{}), req);
+ st.end();
+ });
t.end();
});
| 8 | Fix chained calls usage with mocked methods | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
806 | <NME> index.js
<BEF> 'use strict';
/**
* Helpers to mock the AWS SDK Services using sinon.js under the hood
* Export two functions:
* - mock
* - restore
*
* Mocking is done in two steps:
* - mock of the constructor for the service on AWS
* - mock of the method on the service
**/
const sinon = require('sinon');
const traverse = require('traverse');
let _AWS = require('aws-sdk');
const Readable = require('stream').Readable;
const AWS = {};
const services = {};
/**
* Sets the aws-sdk to be mocked.
*/
AWS.setSDK = function(path) {
_AWS = require(path);
};
AWS.setSDKInstance = function(sdk) {
_AWS = sdk;
};
/**
* Stubs the service and registers the method that needs to be mocked.
*/
AWS.mock = function(service, method, replace) {
// If the service does not exist yet, we need to create and stub it.
if (!services[service]) {
services[service] = {};
/**
* Save the real constructor so we can invoke it later on.
* Uses traverse for easy access to nested services (dot-separated)
*/
services[service].Constructor = traverse(_AWS).get(service.split('.'));
services[service].methodMocks = {};
services[service].invoked = false;
mockService(service);
}
// Register the method to be mocked out.
if (!services[service].methodMocks[method]) {
services[service].methodMocks[method] = { replace: replace };
// If the constructor was already invoked, we need to mock the method here.
if (services[service].invoked) {
services[service].clients.forEach(client => {
mockServiceMethod(service, client, method, replace);
})
}
}
return services[service].methodMocks[method];
};
/**
* Stubs the service and registers the method that needs to be re-mocked.
*/
AWS.remock = function(service, method, replace) {
if (services[service].methodMocks[method]) {
restoreMethod(service, method);
services[service].methodMocks[method] = {
replace: replace
};
}
if (services[service].invoked) {
services[service].clients.forEach(client => {
mockServiceMethod(service, client, method, replace);
})
}
return services[service].methodMocks[method];
}
/**
* Stub the constructor for the service on AWS.
* E.g. calls of new AWS.SNS() are replaced.
*/
function mockService(service) {
const nestedServices = service.split('.');
const method = nestedServices.pop();
const object = traverse(_AWS).get(nestedServices);
const serviceStub = sinon.stub(object, method).callsFake(function(...args) {
services[service].invoked = true;
/**
* Create an instance of the service by calling the real constructor
* we stored before. E.g. const client = new AWS.SNS()
* This is necessary in order to mock methods on the service.
*/
const client = new services[service].Constructor(...args);
services[service].clients = services[service].clients || [];
services[service].clients.push(client);
// Once this has been triggered we can mock out all the registered methods.
for (const key in services[service].methodMocks) {
mockServiceMethod(service, client, key, services[service].methodMocks[key].replace);
};
return client;
});
services[service].stub = serviceStub;
};
/**
* Wraps a sinon stub or jest mock function as a fully functional replacement function
*/
function wrapTestStubReplaceFn(replace) {
if (typeof replace !== 'function' || !(replace._isMockFunction || replace.isSinonProxy)) {
return replace;
}
return (params, cb) => {
// If only one argument is provided, it is the callback
if (!cb) {
cb = params;
params = {};
}
// Spy on the users callback so we can later on determine if it has been called in their replace
const cbSpy = sinon.spy(cb);
try {
// Call the users replace, check how many parameters it expects to determine if we should pass in callback only, or also parameters
const result = replace.length === 1 ? replace(cbSpy) : replace(params, cbSpy);
// If the users replace already called the callback, there's no more need for us do it.
if (cbSpy.called) {
return;
}
if (typeof result.then === 'function') {
result.then(val => cb(undefined, val), err => cb(err));
} else {
cb(undefined, result);
}
} catch (err) {
cb(err);
}
};
}
/**
* Stubs the method on a service.
*
* All AWS service methods take two argument:
* - params: an object.
* - callback: of the form 'function(err, data) {}'.
*/
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
userCallback = args[(args.length || 1) - 1];
} else {
userArgs = args;
}
const havePromises = typeof AWS.Promise === 'function';
let promise, resolve, reject, storedResult;
const tryResolveFromStored = function() {
if (storedResult && promise) {
if (typeof storedResult.then === 'function') {
storedResult.then(resolve, reject)
} else if (storedResult.reject) {
reject(storedResult.reject);
} else {
resolve(storedResult.resolve);
}
}
};
const callback = function(err, data) {
if (!storedResult) {
if (err) {
storedResult = {reject: err};
} else {
storedResult = {resolve: data};
}
}
if (userCallback) {
userCallback(err, data);
}
tryResolveFromStored();
};
const request = {
promise: havePromises ? function() {
if (!promise) {
promise = new AWS.Promise(function (resolve_, reject_) {
resolve = resolve_;
reject = reject_;
});
}
tryResolveFromStored();
return promise;
} : undefined,
createReadStream: function() {
if (storedResult instanceof Readable) {
return storedResult;
}
if (replace instanceof Readable) {
return replace;
} else {
const stream = new Readable();
stream._read = function(size) {
if (typeof replace === 'string' || Buffer.isBuffer(replace)) {
this.push(replace);
}
this.push(null);
};
return stream;
}
},
on: function(eventName, callback) {
return this;
},
send: function(callback) {
callback(storedResult.reject, storedResult.resolve);
}
};
// different locations for the paramValidation property
const config = (client.config || client.options || _AWS.config);
if (config.paramValidation) {
try {
// different strategies to find method, depending on wether the service is nested/unnested
const inputRules =
((client.api && client.api.operations[method]) || client[method] || {}).input;
if (inputRules) {
const params = userArgs[(userArgs.length || 1) - 1];
new _AWS.ParamValidator((client.config || _AWS.config).paramValidation).validate(inputRules, params);
}
} catch (e) {
callback(e, null);
return request;
}
}
// If the value of 'replace' is a function we call it with the arguments.
if (typeof replace === 'function') {
const result = replace.apply(replace, userArgs.concat([callback]));
if (storedResult === undefined && result != null &&
(typeof result.then === 'function' || result instanceof Readable)) {
storedResult = result
}
}
// Else we call the callback with the value of 'replace'.
else {
callback(null, replace);
}
return request;
});
}
/**
* Restores the mocks for just one method on a service, the entire service, or all mocks.
*
* When no parameters are passed, everything will be reset.
* When only the service is passed, that specific service will be reset.
* When a service and method are passed, only that method will be reset.
*/
AWS.restore = function(service, method) {
if (!service) {
restoreAllServices();
} else {
if (method) {
restoreMethod(service, method);
} else {
restoreService(service);
}
};
};
/**
* Restores all mocked service and their corresponding methods.
*/
function restoreAllServices() {
for (const service in services) {
restoreService(service);
}
}
/**
* Restores a single mocked service and its corresponding methods.
*/
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
if (services[service].stub)
services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
}
}
/**
* Restores all mocked methods on a service.
*/
function restoreAllMethods(service) {
for (const method in services[service].methodMocks) {
restoreMethod(service, method);
}
}
/**
* Restores a single mocked method on a service.
*/
function restoreMethod(service, method) {
if (services[service] && services[service].methodMocks[method]) {
if (services[service].methodMocks[method].stub) {
// restore this method on all clients
services[service].clients.forEach(client => {
if (client[method] && typeof client[method].restore === 'function') {
client[method].restore();
}
})
}
delete services[service].methodMocks[method];
} else {
console.log('Method ' + service + ' was never instantiated yet you try to restore it.');
}
}
(function() {
const setPromisesDependency = _AWS.config.setPromisesDependency;
/* istanbul ignore next */
/* only to support for older versions of aws-sdk */
if (typeof setPromisesDependency === 'function') {
AWS.Promise = global.Promise;
_AWS.config.setPromisesDependency = function(p) {
AWS.Promise = p;
return setPromisesDependency(p);
};
}
})();
module.exports = AWS;
<MSG> made restoreService more robust
<DFF> @@ -157,7 +157,8 @@ function restoreAllServices() {
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
- services[service].stub.restore();
+ if( services[service].stub)
+ services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
| 2 | made restoreService more robust | 1 | .js | js | apache-2.0 | dwyl/aws-sdk-mock |
807 | <NME> index.js
<BEF> 'use strict';
/**
* Helpers to mock the AWS SDK Services using sinon.js under the hood
* Export two functions:
* - mock
* - restore
*
* Mocking is done in two steps:
* - mock of the constructor for the service on AWS
* - mock of the method on the service
**/
const sinon = require('sinon');
const traverse = require('traverse');
let _AWS = require('aws-sdk');
const Readable = require('stream').Readable;
const AWS = {};
const services = {};
/**
* Sets the aws-sdk to be mocked.
*/
AWS.setSDK = function(path) {
_AWS = require(path);
};
AWS.setSDKInstance = function(sdk) {
_AWS = sdk;
};
/**
* Stubs the service and registers the method that needs to be mocked.
*/
AWS.mock = function(service, method, replace) {
// If the service does not exist yet, we need to create and stub it.
if (!services[service]) {
services[service] = {};
/**
* Save the real constructor so we can invoke it later on.
* Uses traverse for easy access to nested services (dot-separated)
*/
services[service].Constructor = traverse(_AWS).get(service.split('.'));
services[service].methodMocks = {};
services[service].invoked = false;
mockService(service);
}
// Register the method to be mocked out.
if (!services[service].methodMocks[method]) {
services[service].methodMocks[method] = { replace: replace };
// If the constructor was already invoked, we need to mock the method here.
if (services[service].invoked) {
services[service].clients.forEach(client => {
mockServiceMethod(service, client, method, replace);
})
}
}
return services[service].methodMocks[method];
};
/**
* Stubs the service and registers the method that needs to be re-mocked.
*/
AWS.remock = function(service, method, replace) {
if (services[service].methodMocks[method]) {
restoreMethod(service, method);
services[service].methodMocks[method] = {
replace: replace
};
}
if (services[service].invoked) {
services[service].clients.forEach(client => {
mockServiceMethod(service, client, method, replace);
})
}
return services[service].methodMocks[method];
}
/**
* Stub the constructor for the service on AWS.
* E.g. calls of new AWS.SNS() are replaced.
*/
function mockService(service) {
const nestedServices = service.split('.');
const method = nestedServices.pop();
const object = traverse(_AWS).get(nestedServices);
const serviceStub = sinon.stub(object, method).callsFake(function(...args) {
services[service].invoked = true;
/**
* Create an instance of the service by calling the real constructor
* we stored before. E.g. const client = new AWS.SNS()
* This is necessary in order to mock methods on the service.
*/
const client = new services[service].Constructor(...args);
services[service].clients = services[service].clients || [];
services[service].clients.push(client);
// Once this has been triggered we can mock out all the registered methods.
for (const key in services[service].methodMocks) {
mockServiceMethod(service, client, key, services[service].methodMocks[key].replace);
};
return client;
});
services[service].stub = serviceStub;
};
/**
* Wraps a sinon stub or jest mock function as a fully functional replacement function
*/
function wrapTestStubReplaceFn(replace) {
if (typeof replace !== 'function' || !(replace._isMockFunction || replace.isSinonProxy)) {
return replace;
}
return (params, cb) => {
// If only one argument is provided, it is the callback
if (!cb) {
cb = params;
params = {};
}
// Spy on the users callback so we can later on determine if it has been called in their replace
const cbSpy = sinon.spy(cb);
try {
// Call the users replace, check how many parameters it expects to determine if we should pass in callback only, or also parameters
const result = replace.length === 1 ? replace(cbSpy) : replace(params, cbSpy);
// If the users replace already called the callback, there's no more need for us do it.
if (cbSpy.called) {
return;
}
if (typeof result.then === 'function') {
result.then(val => cb(undefined, val), err => cb(err));
} else {
cb(undefined, result);
}
} catch (err) {
cb(err);
}
};
}
/**
* Stubs the method on a service.
*
* All AWS service methods take two argument:
* - params: an object.
* - callback: of the form 'function(err, data) {}'.
*/
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
userCallback = args[(args.length || 1) - 1];
} else {
userArgs = args;
}
const havePromises = typeof AWS.Promise === 'function';
let promise, resolve, reject, storedResult;
const tryResolveFromStored = function() {
if (storedResult && promise) {
if (typeof storedResult.then === 'function') {
storedResult.then(resolve, reject)
} else if (storedResult.reject) {
reject(storedResult.reject);
} else {
resolve(storedResult.resolve);
}
}
};
const callback = function(err, data) {
if (!storedResult) {
if (err) {
storedResult = {reject: err};
} else {
storedResult = {resolve: data};
}
}
if (userCallback) {
userCallback(err, data);
}
tryResolveFromStored();
};
const request = {
promise: havePromises ? function() {
if (!promise) {
promise = new AWS.Promise(function (resolve_, reject_) {
resolve = resolve_;
reject = reject_;
});
}
tryResolveFromStored();
return promise;
} : undefined,
createReadStream: function() {
if (storedResult instanceof Readable) {
return storedResult;
}
if (replace instanceof Readable) {
return replace;
} else {
const stream = new Readable();
stream._read = function(size) {
if (typeof replace === 'string' || Buffer.isBuffer(replace)) {
this.push(replace);
}
this.push(null);
};
return stream;
}
},
on: function(eventName, callback) {
return this;
},
send: function(callback) {
callback(storedResult.reject, storedResult.resolve);
}
};
// different locations for the paramValidation property
const config = (client.config || client.options || _AWS.config);
if (config.paramValidation) {
try {
// different strategies to find method, depending on wether the service is nested/unnested
const inputRules =
((client.api && client.api.operations[method]) || client[method] || {}).input;
if (inputRules) {
const params = userArgs[(userArgs.length || 1) - 1];
new _AWS.ParamValidator((client.config || _AWS.config).paramValidation).validate(inputRules, params);
}
} catch (e) {
callback(e, null);
return request;
}
}
// If the value of 'replace' is a function we call it with the arguments.
if (typeof replace === 'function') {
const result = replace.apply(replace, userArgs.concat([callback]));
if (storedResult === undefined && result != null &&
(typeof result.then === 'function' || result instanceof Readable)) {
storedResult = result
}
}
// Else we call the callback with the value of 'replace'.
else {
callback(null, replace);
}
return request;
});
}
/**
* Restores the mocks for just one method on a service, the entire service, or all mocks.
*
* When no parameters are passed, everything will be reset.
* When only the service is passed, that specific service will be reset.
* When a service and method are passed, only that method will be reset.
*/
AWS.restore = function(service, method) {
if (!service) {
restoreAllServices();
} else {
if (method) {
restoreMethod(service, method);
} else {
restoreService(service);
}
};
};
/**
* Restores all mocked service and their corresponding methods.
*/
function restoreAllServices() {
for (const service in services) {
restoreService(service);
}
}
/**
* Restores a single mocked service and its corresponding methods.
*/
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
if (services[service].stub)
services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
}
}
/**
* Restores all mocked methods on a service.
*/
function restoreAllMethods(service) {
for (const method in services[service].methodMocks) {
restoreMethod(service, method);
}
}
/**
* Restores a single mocked method on a service.
*/
function restoreMethod(service, method) {
if (services[service] && services[service].methodMocks[method]) {
if (services[service].methodMocks[method].stub) {
// restore this method on all clients
services[service].clients.forEach(client => {
if (client[method] && typeof client[method].restore === 'function') {
client[method].restore();
}
})
}
delete services[service].methodMocks[method];
} else {
console.log('Method ' + service + ' was never instantiated yet you try to restore it.');
}
}
(function() {
const setPromisesDependency = _AWS.config.setPromisesDependency;
/* istanbul ignore next */
/* only to support for older versions of aws-sdk */
if (typeof setPromisesDependency === 'function') {
AWS.Promise = global.Promise;
_AWS.config.setPromisesDependency = function(p) {
AWS.Promise = p;
return setPromisesDependency(p);
};
}
})();
module.exports = AWS;
<MSG> made restoreService more robust
<DFF> @@ -157,7 +157,8 @@ function restoreAllServices() {
function restoreService(service) {
if (services[service]) {
restoreAllMethods(service);
- services[service].stub.restore();
+ if( services[service].stub)
+ services[service].stub.restore();
delete services[service];
} else {
console.log('Service ' + service + ' was never instantiated yet you try to restore it.');
| 2 | made restoreService more robust | 1 | .js | js | apache-2.0 | dwyl/aws-sdk-mock |
808 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.end();
});
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Merge pull request #253 from thomaux/jest
Allow passing in a sinon stub or jest mock as the replacement function
<DFF> @@ -7,6 +7,8 @@ const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
+const jest = require('jest-mock');
+const sinon = require('sinon');
AWS.config.paramValidation = false;
@@ -583,6 +585,76 @@ test('AWS.mock function should mock AWS service and method on the service', func
st.end();
});
+ t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
+ const sinonStub = sinon.stub();
+ sinonStub.returns('message');
+ awsMock.mock('DynamoDB', 'getItem', sinonStub);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(sinonStub.called, true);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
+ const sinonStub = sinon.stub();
+ sinonStub.returns('message');
+ awsMock.mock('DynamoDB', 'getItem', sinonStub);
+ const db = new AWS.DynamoDB();
+ db.getItem({}).promise().then(function(data){
+ st.equal(data, 'message');
+ st.equal(sinonStub.called, true);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
+ const jestMock = jest.fn().mockReturnValue('message');
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
+ const jestMock = jest.fn().mockResolvedValue('message');
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
+ const jestMock = jest.fn().mockImplementation(() => {
+ throw new Error('something went wrong')
+ });
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err){
+ st.equal(err.message, 'something went wrong');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
+ const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err){
+ st.equal(err.message, 'something went wrong');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
t.end();
});
| 72 | Merge pull request #253 from thomaux/jest | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
809 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.end();
});
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Merge pull request #253 from thomaux/jest
Allow passing in a sinon stub or jest mock as the replacement function
<DFF> @@ -7,6 +7,8 @@ const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
+const jest = require('jest-mock');
+const sinon = require('sinon');
AWS.config.paramValidation = false;
@@ -583,6 +585,76 @@ test('AWS.mock function should mock AWS service and method on the service', func
st.end();
});
+ t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
+ const sinonStub = sinon.stub();
+ sinonStub.returns('message');
+ awsMock.mock('DynamoDB', 'getItem', sinonStub);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(sinonStub.called, true);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
+ const sinonStub = sinon.stub();
+ sinonStub.returns('message');
+ awsMock.mock('DynamoDB', 'getItem', sinonStub);
+ const db = new AWS.DynamoDB();
+ db.getItem({}).promise().then(function(data){
+ st.equal(data, 'message');
+ st.equal(sinonStub.called, true);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
+ const jestMock = jest.fn().mockReturnValue('message');
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
+ const jestMock = jest.fn().mockResolvedValue('message');
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err, data){
+ st.equal(data, 'message');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
+ const jestMock = jest.fn().mockImplementation(() => {
+ throw new Error('something went wrong')
+ });
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err){
+ st.equal(err.message, 'something went wrong');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
+ t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
+ const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
+ awsMock.mock('DynamoDB', 'getItem', jestMock);
+ const db = new AWS.DynamoDB();
+ db.getItem({}, function(err){
+ st.equal(err.message, 'something went wrong');
+ st.equal(jestMock.mock.calls.length, 1);
+ st.end();
+ });
+ });
+
t.end();
});
| 72 | Merge pull request #253 from thomaux/jest | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
810 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equals(returnedValue, 'body');
st.end();
});
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> indent req.send function body
<DFF> @@ -508,8 +508,8 @@ test('AWS.mock function should mock AWS service and method on the service', func
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
- returnedValue = data.Body;
- });
+ returnedValue = data.Body;
+ });
st.equals(returnedValue, 'body');
st.end();
});
| 2 | indent req.send function body | 2 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
811 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equals(returnedValue, 'body');
st.end();
});
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> indent req.send function body
<DFF> @@ -508,8 +508,8 @@ test('AWS.mock function should mock AWS service and method on the service', func
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
- returnedValue = data.Body;
- });
+ returnedValue = data.Body;
+ });
st.equals(returnedValue, 'body');
st.end();
});
| 2 | indent req.send function body | 2 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
812 | <NME> workflow.yml
<BEF> name: Node CI
on: [push, pull_request]
jobs:
test:
runs-on: ${{ matrix.os }}
strategy:
matrix:
node-version: [12.x, 14.x, 16.x]
os: [ubuntu-latest, windows-latest, macos-latest]
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: ${{ matrix.node-version }}
- run: npm i
- run: npm run lint
- run: npm test
<MSG> add nodejs18 to ci settings
Because nodejs18 has been released.
<DFF> @@ -7,7 +7,7 @@ jobs:
runs-on: ${{ matrix.os }}
strategy:
matrix:
- node-version: [12.x, 14.x, 16.x]
+ node-version: [12.x, 14.x, 16.x, 18.x]
os: [ubuntu-latest, windows-latest, macos-latest]
steps:
- uses: actions/checkout@v2
| 1 | add nodejs18 to ci settings | 1 | .yml | github/workflows/workflow | apache-2.0 | dwyl/aws-sdk-mock |
813 | <NME> workflow.yml
<BEF> name: Node CI
on: [push, pull_request]
jobs:
test:
runs-on: ${{ matrix.os }}
strategy:
matrix:
node-version: [12.x, 14.x, 16.x]
os: [ubuntu-latest, windows-latest, macos-latest]
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: ${{ matrix.node-version }}
- run: npm i
- run: npm run lint
- run: npm test
<MSG> add nodejs18 to ci settings
Because nodejs18 has been released.
<DFF> @@ -7,7 +7,7 @@ jobs:
runs-on: ${{ matrix.os }}
strategy:
matrix:
- node-version: [12.x, 14.x, 16.x]
+ node-version: [12.x, 14.x, 16.x, 18.x]
os: [ubuntu-latest, windows-latest, macos-latest]
steps:
- uses: actions/checkout@v2
| 1 | add nodejs18 to ci settings | 1 | .yml | github/workflows/workflow | apache-2.0 | dwyl/aws-sdk-mock |
814 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import * as AWSMock from "aws-sdk-mock";
import * as AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> update readme with correct import examples
<DFF> @@ -74,8 +74,8 @@ AWS.restore('S3');
#### Using TypeScript
```typescript
-import * as AWSMock from "aws-sdk-mock";
-import * as AWS from "aws-sdk";
+import AWSMock from "aws-sdk-mock";
+import AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
| 2 | update readme with correct import examples | 2 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
815 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import * as AWSMock from "aws-sdk-mock";
import * as AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> update readme with correct import examples
<DFF> @@ -74,8 +74,8 @@ AWS.restore('S3');
#### Using TypeScript
```typescript
-import * as AWSMock from "aws-sdk-mock";
-import * as AWS from "aws-sdk";
+import AWSMock from "aws-sdk-mock";
+import AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
| 2 | update readme with correct import examples | 2 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
816 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
awsMock.mock('SNS', 'publish', 'message')
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Merge pull request #49 from mikkelfish/master
feat: setSDKInstance to allow mocking of specific aws objects
<DFF> @@ -444,7 +444,36 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
awsMock.mock('SNS', 'publish', 'message')
});
awsMock.setSDK('aws-sdk');
+ awsMock.restore()
+
+ st.end();
+ });
+ t.end();
+});
+
+test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
+ t.test('Specific Modules can be set for mocking', function(st) {
+ var aws2 = require('aws-sdk')
+ awsMock.setSDKInstance(aws2);
+ awsMock.mock('SNS', 'publish', 'message2');
+ var sns = new AWS.SNS();
+ sns.publish({}, function(err, data){
+ st.equals(data, 'message2');
+ awsMock.restore('SNS');
+ st.end();
+ })
+ });
+
+ t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
+ var bad = {}
+ awsMock.setSDKInstance(bad);
+ st.throws(function() {
+ awsMock.mock('SNS', 'publish', 'message')
+ });
+ awsMock.setSDKInstance(AWS);
+ awsMock.restore()
st.end();
});
t.end();
});
+
| 29 | Merge pull request #49 from mikkelfish/master | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
817 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
awsMock.mock('SNS', 'publish', 'message')
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Merge pull request #49 from mikkelfish/master
feat: setSDKInstance to allow mocking of specific aws objects
<DFF> @@ -444,7 +444,36 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
awsMock.mock('SNS', 'publish', 'message')
});
awsMock.setSDK('aws-sdk');
+ awsMock.restore()
+
+ st.end();
+ });
+ t.end();
+});
+
+test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
+ t.test('Specific Modules can be set for mocking', function(st) {
+ var aws2 = require('aws-sdk')
+ awsMock.setSDKInstance(aws2);
+ awsMock.mock('SNS', 'publish', 'message2');
+ var sns = new AWS.SNS();
+ sns.publish({}, function(err, data){
+ st.equals(data, 'message2');
+ awsMock.restore('SNS');
+ st.end();
+ })
+ });
+
+ t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
+ var bad = {}
+ awsMock.setSDKInstance(bad);
+ st.throws(function() {
+ awsMock.mock('SNS', 'publish', 'message')
+ });
+ awsMock.setSDKInstance(AWS);
+ awsMock.restore()
st.end();
});
t.end();
});
+
| 29 | Merge pull request #49 from mikkelfish/master | 0 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
818 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equals(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equals(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equals(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equals(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equals(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equals(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equals(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equals(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equals(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equals(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equals(promise.constructor.name, 'P');
promise.then(function(data) {
st.equals(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equals(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equals(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equals(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns1.publish.isSinonProxy, true);
st.equals(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns1.publish.isSinonProxy, true);
st.equals(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(sns.publish.isSinonProxy, true);
st.equals(docClient.put.isSinonProxy, true);
st.equals(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.put.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equals(data, 'message');
docClient.get({}, function(err, data){
st.equals(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
console.warn(err);
st.equals(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equals(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equals(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equals(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equals(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equals(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> upgrade packages
I got a DeprecationWarning after upgrading, so I fixed the test.
```
DeprecationWarning: equals() is deprecated, use equal() instead
```
<DFF> @@ -21,7 +21,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -31,7 +31,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -39,12 +39,12 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
- st.equals(data.test, 'message');
+ st.equal(data.test, 'message');
st.end();
});
});
@@ -62,7 +62,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -71,7 +71,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
- st.equals(data.Body, 'body');
+ st.equal(data.Body, 'body');
st.end();
});
});
@@ -84,7 +84,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -97,7 +97,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
- st.equals(data, 'test');
+ st.equal(data, 'test');
st.end();
});
});
@@ -110,7 +110,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
st.end();
});
});
@@ -126,10 +126,10 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
sns1.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
st.end();
});
});
@@ -143,9 +143,9 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -161,11 +161,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -179,11 +179,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -209,11 +209,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -232,9 +232,9 @@ test('AWS.mock function should mock AWS service and method on the service', func
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
- st.equals(promise.constructor.name, 'P');
+ st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -262,7 +262,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -275,7 +275,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -285,7 +285,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -295,7 +295,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -305,7 +305,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), '');
+ st.equal(actual.toString(), '');
st.end();
}));
});
@@ -315,7 +315,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), '');
+ st.equal(actual.toString(), '');
st.end();
}));
});
@@ -323,25 +323,25 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
- st.equals(typeof req.on, 'function');
+ st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
- st.equals(typeof req.send, 'function');
+ st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
- st.equals(AWS.SNS.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
@@ -349,13 +349,13 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'message');
});
const sns = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
- st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
+ st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
@@ -366,15 +366,15 @@ test('AWS.mock function should mock AWS service and method on the service', func
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns1.publish.isSinonProxy, true);
- st.equals(sns2.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns1.publish.isSinonProxy, true);
+ st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
- st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
+ st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
@@ -385,15 +385,15 @@ test('AWS.mock function should mock AWS service and method on the service', func
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns1.publish.isSinonProxy, true);
- st.equals(sns2.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns1.publish.isSinonProxy, true);
+ st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
@@ -410,21 +410,21 @@ test('AWS.mock function should mock AWS service and method on the service', func
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(sns.publish.isSinonProxy, true);
- st.equals(docClient.put.isSinonProxy, true);
- st.equals(dynamoDb.putItem.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(sns.publish.isSinonProxy, true);
+ st.equal(docClient.put.isSinonProxy, true);
+ st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
@@ -437,22 +437,22 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.put.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.put.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
docClient.get({}, function(err, data){
- st.equals(data, 'test');
+ st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
@@ -463,11 +463,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.query.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
- console.warn(err);
- st.equals(data, 'test');
+ st.is(err, null);
+ st.equal(data, 'test');
st.end();
});
});
@@ -477,37 +477,37 @@ test('AWS.mock function should mock AWS service and method on the service', func
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
@@ -527,18 +527,18 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
csd.search({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
- st.equals(stub.stub.isSinonProxy, true);
+ st.equal(stub.stub.isSinonProxy, true);
st.end();
});
@@ -569,7 +569,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
- st.equals(req.on('httpHeaders', ()=>{}), req);
+ st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
@@ -581,7 +581,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
req.send(async (err, data) => {
returnedValue = data.Body;
});
- st.equals(returnedValue, 'body');
+ st.equal(returnedValue, 'body');
st.end();
});
@@ -708,7 +708,7 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -739,7 +739,7 @@ test('AWS.setSDKInstance function should mock a specific AWS module', function(t
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message2');
+ st.equal(data, 'message2');
st.end();
});
});
| 99 | upgrade packages | 99 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
819 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equals(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equals(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equals(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equals(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equals(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equals(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equals(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equals(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equals(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equals(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equals(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equals(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equals(promise.constructor.name, 'P');
promise.then(function(data) {
st.equals(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equals(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equals(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equals(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equals(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns1.publish.isSinonProxy, true);
st.equals(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(sns1.publish.isSinonProxy, true);
st.equals(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equals(AWS.SNS.isSinonProxy, true);
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(sns.publish.isSinonProxy, true);
st.equals(docClient.put.isSinonProxy, true);
st.equals(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.put.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equals(data, 'message');
docClient.get({}, function(err, data){
st.equals(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
console.warn(err);
st.equals(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.isSinonProxy, true);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
st.equals(AWS.DynamoDB.isSinonProxy, true);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equals(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equals(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equals(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equals(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equals(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equals(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> upgrade packages
I got a DeprecationWarning after upgrading, so I fixed the test.
```
DeprecationWarning: equals() is deprecated, use equal() instead
```
<DFF> @@ -21,7 +21,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -31,7 +31,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -39,12 +39,12 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
- st.equals(data.test, 'message');
+ st.equal(data.test, 'message');
st.end();
});
});
@@ -62,7 +62,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -71,7 +71,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
- st.equals(data.Body, 'body');
+ st.equal(data.Body, 'body');
st.end();
});
});
@@ -84,7 +84,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -97,7 +97,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
- st.equals(data, 'test');
+ st.equal(data, 'test');
st.end();
});
});
@@ -110,7 +110,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
st.end();
});
});
@@ -126,10 +126,10 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
sns1.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
- st.equals(data, 'message 2');
+ st.equal(data, 'message 2');
st.end();
});
});
@@ -143,9 +143,9 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -161,11 +161,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -179,11 +179,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -209,11 +209,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
- st.equals(data, error);
+ st.equal(data, error);
st.end();
});
});
@@ -232,9 +232,9 @@ test('AWS.mock function should mock AWS service and method on the service', func
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
- st.equals(promise.constructor.name, 'P');
+ st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -262,7 +262,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -275,7 +275,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -285,7 +285,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -295,7 +295,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), 'body');
+ st.equal(actual.toString(), 'body');
st.end();
}));
});
@@ -305,7 +305,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), '');
+ st.equal(actual.toString(), '');
st.end();
}));
});
@@ -315,7 +315,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
- st.equals(actual.toString(), '');
+ st.equal(actual.toString(), '');
st.end();
}));
});
@@ -323,25 +323,25 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
- st.equals(typeof req.on, 'function');
+ st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
- st.equals(typeof req.send, 'function');
+ st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
- st.equals(AWS.SNS.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
@@ -349,13 +349,13 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'message');
});
const sns = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
- st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
+ st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
@@ -366,15 +366,15 @@ test('AWS.mock function should mock AWS service and method on the service', func
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns1.publish.isSinonProxy, true);
- st.equals(sns2.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns1.publish.isSinonProxy, true);
+ st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
- st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
+ st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
@@ -385,15 +385,15 @@ test('AWS.mock function should mock AWS service and method on the service', func
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(sns1.publish.isSinonProxy, true);
- st.equals(sns2.publish.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(sns1.publish.isSinonProxy, true);
+ st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns1.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns2.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
@@ -410,21 +410,21 @@ test('AWS.mock function should mock AWS service and method on the service', func
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.SNS.isSinonProxy, true);
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(sns.publish.isSinonProxy, true);
- st.equals(docClient.put.isSinonProxy, true);
- st.equals(dynamoDb.putItem.isSinonProxy, true);
+ st.equal(AWS.SNS.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(sns.publish.isSinonProxy, true);
+ st.equal(docClient.put.isSinonProxy, true);
+ st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
- st.equals(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(sns.publish.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
@@ -437,22 +437,22 @@ test('AWS.mock function should mock AWS service and method on the service', func
callback(null, 'test');
});
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.put.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.put.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
docClient.get({}, function(err, data){
- st.equals(data, 'test');
+ st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.put.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
@@ -463,11 +463,11 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(docClient.query.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
- console.warn(err);
- st.equals(data, 'test');
+ st.is(err, null);
+ st.equal(data, 'test');
st.end();
});
});
@@ -477,37 +477,37 @@ test('AWS.mock function should mock AWS service and method on the service', func
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
dynamoDb = new AWS.DynamoDB();
- st.equals(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.isSinonProxy, true);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.isSinonProxy, true);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB.DocumentClient');
// the first assertion is true because DynamoDB is still mocked
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
- st.equals(AWS.DynamoDB.isSinonProxy, true);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.getItem.isSinonProxy, true);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), true);
+ st.equal(AWS.DynamoDB.isSinonProxy, true);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
- st.equals(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
- st.equals(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
- st.equals(docClient.get.hasOwnProperty('isSinonProxy'), false);
- st.equals(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
+ st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
+ st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
+ st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
@@ -527,18 +527,18 @@ test('AWS.mock function should mock AWS service and method on the service', func
});
csd.search({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
- st.equals(data, 'message');
+ st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
- st.equals(stub.stub.isSinonProxy, true);
+ st.equal(stub.stub.isSinonProxy, true);
st.end();
});
@@ -569,7 +569,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
- st.equals(req.on('httpHeaders', ()=>{}), req);
+ st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
@@ -581,7 +581,7 @@ test('AWS.mock function should mock AWS service and method on the service', func
req.send(async (err, data) => {
returnedValue = data.Body;
});
- st.equals(returnedValue, 'body');
+ st.equal(returnedValue, 'body');
st.end();
});
@@ -708,7 +708,7 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message');
+ st.equal(data, 'message');
st.end();
});
});
@@ -739,7 +739,7 @@ test('AWS.setSDKInstance function should mock a specific AWS module', function(t
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
- st.equals(data, 'message2');
+ st.equal(data, 'message2');
st.end();
});
});
| 99 | upgrade packages | 99 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
820 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](https://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import AWSMock from 'aws-sdk-mock';
import AWS from 'aws-sdk';
import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> change badges in readme to use Shields.io for "flat-square"
<DFF> @@ -2,8 +2,8 @@
AWSome mocks for Javascript aws-sdk services.
-[](https://travis-ci.org/dwyl/aws-sdk-mock)
-[](https://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
+[](https://travis-ci.org/dwyl/aws-sdk-mock)
+[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
| 2 | change badges in readme to use Shields.io for "flat-square" | 2 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
821 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](https://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import AWSMock from 'aws-sdk-mock';
import AWS from 'aws-sdk';
import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
describe('the module', () => {
/**
TESTS below here
**/
it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: 'foo', sk: 'bar'});
});
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> change badges in readme to use Shields.io for "flat-square"
<DFF> @@ -2,8 +2,8 @@
AWSome mocks for Javascript aws-sdk services.
-[](https://travis-ci.org/dwyl/aws-sdk-mock)
-[](https://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
+[](https://travis-ci.org/dwyl/aws-sdk-mock)
+[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
| 2 | change badges in readme to use Shields.io for "flat-square" | 2 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
822 | <NME> index.d.ts
<BEF> declare module 'aws-sdk-mock' {
function mock(service: string, method: string, replace: any): void;
function mock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
function remock(service: string, method: string, replace: any): void;
function remock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
function restore(service?: string, method?: string): void;
function setSDK(path: string): void;
}
export type Callback<D> = (err: AWSError | undefined, data: D) => void;
export type ReplaceFn<C extends ClientName, M extends MethodName<C>> = (params: AWSRequest<C, M>, callback: AWSCallback<C, M>) => void
export function mock<C extends ClientName, M extends MethodName<C>>(
service: C,
method: M,
replace: ReplaceFn<C, M>,
): void;
export function mock<C extends ClientName, NC extends NestedClientName<C>>(service: NestedClientFullName<C, NC>, method: NestedMethodName<C, NC>, replace: any): void;
export function mock<C extends ClientName>(service: C, method: MethodName<C>, replace: any): void;
export function remock<C extends ClientName, M extends MethodName<C>>(
service: C,
method: M,
replace: ReplaceFn<C, M>,
): void;
export function remock<C extends ClientName>(service: C, method: MethodName<C>, replace: any): void;
export function remock<C extends ClientName, NC extends NestedClientName<C>>(service: NestedClientFullName<C, NC>, method: NestedMethodName<C, NC>, replace: any): void;
export function restore<C extends ClientName>(service?: C, method?: MethodName<C>): void;
export function restore<C extends ClientName, NC extends NestedClientName<C>>(service?: NestedClientFullName<C, NC>, method?: NestedMethodName<C, NC>): void;
export function setSDK(path: string): void;
export function setSDKInstance(instance: typeof import('aws-sdk')): void;
/**
* The SDK defines a class for each service as well as a namespace with the same name.
* Nested clients, e.g. DynamoDB.DocumentClient, are defined on the namespace, not the class.
* That is why we need to fetch these separately as defined below in the NestedClientName<C> type
*
* The NestedClientFullName type supports validating strings representing a nested clients name in dot notation
*
* We add the ts-ignore comments to avoid the type system to trip over the many possible values for NestedClientName<C>
*/
export type NestedClientName<C extends ClientName> = keyof typeof AWS[C];
// @ts-ignore
export type NestedClientFullName<C extends ClientName, NC extends NestedClientName<C>> = `${C}.${NC}`;
// @ts-ignore
export type NestedClient<C extends ClientName, NC extends NestedClientName<C>> = InstanceType<(typeof AWS)[C][NC]>;
// @ts-ignore
export type NestedMethodName<C extends ClientName, NC extends NestedClientName<C>> = keyof ExtractMethod<NestedClient<C, NC>>;
<MSG> 'reorder'
<DFF> @@ -1,19 +1,20 @@
declare module 'aws-sdk-mock' {
- function mock(service: string, method: string, replace: any): void;
-
function mock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
- function remock(service: string, method: string, replace: any): void;
+ function mock(service: string, method: string, replace: any): void;
+
function remock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
+ function remock(service: string, method: string, replace: any): void;
+
function restore(service?: string, method?: string): void;
function setSDK(path: string): void;
| 4 | 'reorder' | 3 | .ts | d | apache-2.0 | dwyl/aws-sdk-mock |
823 | <NME> index.d.ts
<BEF> declare module 'aws-sdk-mock' {
function mock(service: string, method: string, replace: any): void;
function mock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
function remock(service: string, method: string, replace: any): void;
function remock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
function restore(service?: string, method?: string): void;
function setSDK(path: string): void;
}
export type Callback<D> = (err: AWSError | undefined, data: D) => void;
export type ReplaceFn<C extends ClientName, M extends MethodName<C>> = (params: AWSRequest<C, M>, callback: AWSCallback<C, M>) => void
export function mock<C extends ClientName, M extends MethodName<C>>(
service: C,
method: M,
replace: ReplaceFn<C, M>,
): void;
export function mock<C extends ClientName, NC extends NestedClientName<C>>(service: NestedClientFullName<C, NC>, method: NestedMethodName<C, NC>, replace: any): void;
export function mock<C extends ClientName>(service: C, method: MethodName<C>, replace: any): void;
export function remock<C extends ClientName, M extends MethodName<C>>(
service: C,
method: M,
replace: ReplaceFn<C, M>,
): void;
export function remock<C extends ClientName>(service: C, method: MethodName<C>, replace: any): void;
export function remock<C extends ClientName, NC extends NestedClientName<C>>(service: NestedClientFullName<C, NC>, method: NestedMethodName<C, NC>, replace: any): void;
export function restore<C extends ClientName>(service?: C, method?: MethodName<C>): void;
export function restore<C extends ClientName, NC extends NestedClientName<C>>(service?: NestedClientFullName<C, NC>, method?: NestedMethodName<C, NC>): void;
export function setSDK(path: string): void;
export function setSDKInstance(instance: typeof import('aws-sdk')): void;
/**
* The SDK defines a class for each service as well as a namespace with the same name.
* Nested clients, e.g. DynamoDB.DocumentClient, are defined on the namespace, not the class.
* That is why we need to fetch these separately as defined below in the NestedClientName<C> type
*
* The NestedClientFullName type supports validating strings representing a nested clients name in dot notation
*
* We add the ts-ignore comments to avoid the type system to trip over the many possible values for NestedClientName<C>
*/
export type NestedClientName<C extends ClientName> = keyof typeof AWS[C];
// @ts-ignore
export type NestedClientFullName<C extends ClientName, NC extends NestedClientName<C>> = `${C}.${NC}`;
// @ts-ignore
export type NestedClient<C extends ClientName, NC extends NestedClientName<C>> = InstanceType<(typeof AWS)[C][NC]>;
// @ts-ignore
export type NestedMethodName<C extends ClientName, NC extends NestedClientName<C>> = keyof ExtractMethod<NestedClient<C, NC>>;
<MSG> 'reorder'
<DFF> @@ -1,19 +1,20 @@
declare module 'aws-sdk-mock' {
- function mock(service: string, method: string, replace: any): void;
-
function mock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
- function remock(service: string, method: string, replace: any): void;
+ function mock(service: string, method: string, replace: any): void;
+
function remock(
service: string,
method: string,
replace: (params: any, callback: (err: any, data: any) => void) => void
): void;
+ function remock(service: string, method: string, replace: any): void;
+
function restore(service?: string, method?: string): void;
function setSDK(path: string): void;
| 4 | 'reorder' | 3 | .ts | d | apache-2.0 | dwyl/aws-sdk-mock |
824 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.ok(signer);
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Update "Modules with multi-parameter..." test to assert on returned type
<DFF> @@ -492,11 +492,11 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
- awsMock.setSDK('aws-sdk');
- awsMock.mock('CloudFront.Signer', 'getSignedUrl');
- var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
- st.ok(signer);
- st.end();
+ awsMock.setSDK('aws-sdk');
+ awsMock.mock('CloudFront.Signer', 'getSignedUrl');
+ var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
+ st.type(signer, 'Signer');
+ st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
| 5 | Update "Modules with multi-parameter..." test to assert on returned type | 5 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
825 | <NME> index.test.js
<BEF> 'use strict';
const tap = require('tap');
const test = tap.test;
const awsMock = require('../index.js');
const AWS = require('aws-sdk');
const isNodeStream = require('is-node-stream');
const concatStream = require('concat-stream');
const Readable = require('stream').Readable;
const jest = require('jest-mock');
const sinon = require('sinon');
AWS.config.paramValidation = false;
tap.afterEach(() => {
awsMock.restore();
});
test('AWS.mock function should mock AWS service and method on the service', function(t){
t.test('mock function replaces method with a function that returns replace string', function(st){
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('mock function replaces method with replace function', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('method which accepts any number of arguments can be mocked', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3();
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
awsMock.mock('S3', 'upload', function(params, options, callback) {
callback(null, options);
});
s3.upload({}, {test: 'message'}, function(err, data) {
st.equal(data.test, 'message');
st.end();
});
});
});
t.test('method fails on invalid input if paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', notKey: 'k'}, function(err, data) {
st.ok(err);
st.notOk(data);
st.end();
});
});
t.test('method with no input rules can be mocked even if paramValidation is set', function(st) {
awsMock.mock('S3', 'getSignedUrl', 'message');
const s3 = new AWS.S3({paramValidation: true});
s3.getSignedUrl('getObject', {}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
t.test('method succeeds on valid input when paramValidation is set', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3({paramValidation: true});
s3.getObject({Bucket: 'b', Key: 'k'}, function(err, data) {
st.notOk(err);
st.equal(data.Body, 'body');
st.end();
});
});
t.test('method is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'test');
});
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('service is not re-mocked if a mock already exists', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'test');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'test');
st.end();
});
});
t.test('service is re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
t.test('all instances of service are re-mocked when remock called', function(st){
awsMock.mock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 1');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
awsMock.remock('SNS', 'subscribe', function(params, callback){
callback(null, 'message 2');
});
sns1.subscribe({}, function(err, data){
st.equal(data, 'message 2');
sns2.subscribe({}, function(err, data){
st.equal(data, 'message 2');
st.end();
});
});
});
t.test('multiple methods can be mocked on the same service', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}, function(err, data) {
st.equal(data, 'message');
lambda.createFunction({}, function(err, data) {
st.equal(data, 'message');
st.end();
});
});
});
if (typeof Promise === 'function') {
t.test('promises are supported', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
callback(error, 'message');
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('replacement returns thennable', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params) {
return Promise.resolve('message')
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
return Promise.reject(error)
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('no unhandled promise rejections when promises are not used', function(st) {
process.on('unhandledRejection', function(reason, promise) {
st.fail('unhandledRejection, reason follows');
st.error(reason);
});
awsMock.mock('S3', 'getObject', function(params, callback) {
callback('This is a test error to see if promise rejections go unhandled');
});
const S3 = new AWS.S3();
S3.getObject({}, function(err, data) {});
st.end();
});
t.test('promises work with async completion', function(st){
const error = new Error('on purpose');
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
setTimeout(callback.bind(this, null, 'message'), 10);
});
awsMock.mock('Lambda', 'createFunction', function(params, callback) {
setTimeout(callback.bind(this, error, 'message'), 10);
});
const lambda = new AWS.Lambda();
lambda.getFunction({}).promise().then(function(data) {
st.equal(data, 'message');
}).then(function(){
return lambda.createFunction({}).promise();
}).catch(function(data){
st.equal(data, error);
st.end();
});
});
t.test('promises can be configured', function(st){
awsMock.mock('Lambda', 'getFunction', function(params, callback) {
callback(null, 'message');
});
const lambda = new AWS.Lambda();
function P(handler) {
const self = this;
function yay (value) {
self.value = value;
}
handler(yay, function(){});
}
P.prototype.then = function(yay) { if (this.value) yay(this.value) };
AWS.config.setPromisesDependency(P);
const promise = lambda.getFunction({}).promise();
st.equal(promise.constructor.name, 'P');
promise.then(function(data) {
st.equal(data, 'message');
st.end();
});
});
}
t.test('request object supports createReadStream', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
let req = s3.getObject('getObject', function(err, data) {});
st.ok(isNodeStream(req.createReadStream()));
// with or without callback
req = s3.getObject('getObject');
st.ok(isNodeStream(req.createReadStream()));
// stream is currently always empty but that's subject to change.
// let's just consume it and ignore the contents
req = s3.getObject('getObject');
const stream = req.createReadStream();
stream.pipe(concatStream(function() {
st.end();
}));
});
t.test('request object createReadStream works with streams', function(st) {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
awsMock.mock('S3', 'getObject', bodyStream);
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with returned streams', function(st) {
awsMock.mock('S3', 'getObject', () => {
const bodyStream = new Readable();
bodyStream.push('body');
bodyStream.push(null);
return bodyStream;
});
const stream = new AWS.S3().getObject('getObject').createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with strings', function(st) {
awsMock.mock('S3', 'getObject', 'body');
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream works with buffers', function(st) {
awsMock.mock('S3', 'getObject', Buffer.alloc(4, 'body'));
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), 'body');
st.end();
}));
});
t.test('request object createReadStream ignores functions', function(st) {
awsMock.mock('S3', 'getObject', function(){});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('request object createReadStream ignores non-buffer objects', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
const stream = req.createReadStream();
stream.pipe(concatStream(function(actual) {
st.equal(actual.toString(), '');
st.end();
}));
});
t.test('call on method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.on, 'function');
st.end();
});
t.test('call send method of request object', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
st.equal(typeof req.send, 'function');
st.end();
});
t.test('all the methods on a service are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
st.equal(AWS.SNS.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('only the method on the service is restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('method on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS', 'publish');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), true);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all methods on all service instances are restored', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
const sns1 = new AWS.SNS();
const sns2 = new AWS.SNS();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(sns1.publish.isSinonProxy, true);
st.equal(sns2.publish.isSinonProxy, true);
awsMock.restore('SNS');
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(sns1.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(sns2.publish.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('all the services are restored when no arguments given to awsMock.restore', function(st){
awsMock.mock('SNS', 'publish', function(params, callback){
callback(null, 'message');
});
awsMock.mock('DynamoDB', 'putItem', function(params, callback){
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback){
callback(null, 'test');
});
const sns = new AWS.SNS();
const docClient = new AWS.DynamoDB.DocumentClient();
const dynamoDb = new AWS.DynamoDB();
st.equal(AWS.SNS.isSinonProxy, true);
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(sns.publish.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(dynamoDb.putItem.isSinonProxy, true);
awsMock.restore();
st.equal(AWS.SNS.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(sns.publish.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.putItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('a nested service can be mocked properly', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'put', 'message');
const docClient = new AWS.DynamoDB.DocumentClient();
awsMock.mock('DynamoDB.DocumentClient', 'put', function(params, callback) {
callback(null, 'test');
});
awsMock.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, 'test');
});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.put.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
docClient.put({}, function(err, data){
st.equal(data, 'message');
docClient.get({}, function(err, data){
st.equal(data, 'test');
awsMock.restore('DynamoDB.DocumentClient', 'get');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
awsMock.restore('DynamoDB.DocumentClient');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.put.hasOwnProperty('isSinonProxy'), false);
st.end();
});
});
});
t.test('a nested service can be mocked properly even when paramValidation is set', function(st){
awsMock.mock('DynamoDB.DocumentClient', 'query', function(params, callback) {
callback(null, 'test');
});
const docClient = new AWS.DynamoDB.DocumentClient({paramValidation: true});
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(docClient.query.isSinonProxy, true);
docClient.query({}, function(err, data){
st.equal(err, null);
st.equal(data, 'test');
st.end();
});
});
t.test('a mocked service and a mocked nested service can coexist as long as the nested service is mocked first', function(st) {
awsMock.mock('DynamoDB.DocumentClient', 'get', 'message');
awsMock.mock('DynamoDB', 'getItem', 'test');
const docClient = new AWS.DynamoDB.DocumentClient();
let dynamoDb = new AWS.DynamoDB();
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.isSinonProxy, true);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.isSinonProxy, true);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.isSinonProxy, true);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
awsMock.mock('DynamoDB', 'getItem', 'test');
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.ok(signer);
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.isSinonProxy, true);
awsMock.restore('DynamoDB');
st.equal(AWS.DynamoDB.DocumentClient.hasOwnProperty('isSinonProxy'), false);
st.equal(AWS.DynamoDB.hasOwnProperty('isSinonProxy'), false);
st.equal(docClient.get.hasOwnProperty('isSinonProxy'), false);
st.equal(dynamoDb.getItem.hasOwnProperty('isSinonProxy'), false);
st.end();
});
t.test('Mocked services should use the implementation configuration arguments without complaining they are missing', function(st) {
awsMock.mock('CloudSearchDomain', 'search', function(params, callback) {
return callback(null, 'message');
});
const csd = new AWS.CloudSearchDomain({
endpoint: 'some endpoint',
region: 'eu-west'
});
awsMock.mock('CloudSearchDomain', 'suggest', function(params, callback) {
return callback(null, 'message');
});
csd.search({}, function(err, data) {
st.equal(data, 'message');
});
csd.suggest({}, function(err, data) {
st.equal(data, 'message');
});
st.end();
});
t.skip('Mocked service should return the sinon stub', function(st) {
// TODO: the stub is only returned if an instance was already constructed
const stub = awsMock.mock('CloudSearchDomain', 'search');
st.equal(stub.stub.isSinonProxy, true);
st.end();
});
t.test('Restore should not fail when the stub did not exist.', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('Lambda');
awsMock.restore('SES', 'sendEmail');
awsMock.restore('CloudSearchDomain', 'doesnotexist');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Restore should not fail when service was not mocked', function (st) {
// This test will fail when restoring throws unneeded errors.
try {
awsMock.restore('CloudFormation');
awsMock.restore('UnknownService');
st.end();
} catch (e) {
console.log(e);
}
});
t.test('Mocked service should allow chained calls after listening to events', function (st) {
awsMock.mock('S3', 'getObject');
const s3 = new AWS.S3();
const req = s3.getObject({Bucket: 'b', notKey: 'k'});
st.equal(req.on('httpHeaders', ()=>{}), req);
st.end();
});
t.test('Mocked service should return replaced function when request send is called', function(st) {
awsMock.mock('S3', 'getObject', {Body: 'body'});
let returnedValue = '';
const s3 = new AWS.S3();
const req = s3.getObject('getObject', {});
req.send(async (err, data) => {
returnedValue = data.Body;
});
st.equal(returnedValue, 'body');
st.end();
});
t.test('mock function replaces method with a sinon stub and returns successfully using callback', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a sinon stub and returns successfully using promise', function(st) {
const sinonStub = sinon.stub();
sinonStub.returns('message');
awsMock.mock('DynamoDB', 'getItem', sinonStub);
const db = new AWS.DynamoDB();
db.getItem({}).promise().then(function(data){
st.equal(data, 'message');
st.equal(sinonStub.called, true);
st.end();
});
});
t.test('mock function replaces method with a jest mock and returns successfully', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock returning successfully and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn().mockReturnValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and resolves successfully', function(st) {
const jestMock = jest.fn().mockResolvedValue('message');
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(data, 'message');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and fails successfully', function(st) {
const jestMock = jest.fn(() => {
throw new Error('something went wrong')
});
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock and rejects successfully', function(st) {
const jestMock = jest.fn().mockRejectedValue(new Error('something went wrong'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err){
st.equal(err.message, 'something went wrong');
st.equal(jestMock.mock.calls.length, 1);
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem({}, function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation and allows mocked method to be called with only callback', function(st) {
const jestMock = jest.fn((params, cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.test('mock function replaces method with a jest mock with implementation expecting only a callback', function(st) {
const jestMock = jest.fn((cb) => cb(null, 'item'));
awsMock.mock('DynamoDB', 'getItem', jestMock);
const db = new AWS.DynamoDB();
db.getItem(function(err, data){
st.equal(jestMock.mock.calls.length, 1);
st.equal(data, 'item');
st.end();
});
});
t.end();
});
test('AWS.setSDK function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('SNS', 'publish', 'message');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message');
st.end();
});
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
awsMock.setSDK('aws-sdk');
awsMock.mock('CloudFront.Signer', 'getSignedUrl');
const signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
st.type(signer, 'Signer');
st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
awsMock.setSDK('sinon');
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDK('aws-sdk');
st.end();
});
t.end();
});
test('AWS.setSDKInstance function should mock a specific AWS module', function(t) {
t.test('Specific Modules can be set for mocking', function(st) {
const aws2 = require('aws-sdk');
awsMock.setSDKInstance(aws2);
awsMock.mock('SNS', 'publish', 'message2');
const sns = new AWS.SNS();
sns.publish({}, function(err, data){
st.equal(data, 'message2');
st.end();
});
});
t.test('Setting the aws-sdk to the wrong instance can cause an exception when mocking', function(st) {
const bad = {};
awsMock.setSDKInstance(bad);
st.throws(function() {
awsMock.mock('SNS', 'publish', 'message');
});
awsMock.setSDKInstance(AWS);
st.end();
});
t.end();
});
<MSG> Update "Modules with multi-parameter..." test to assert on returned type
<DFF> @@ -492,11 +492,11 @@ test('AWS.setSDK function should mock a specific AWS module', function(t) {
});
t.test('Modules with multi-parameter constructors can be set for mocking', function(st) {
- awsMock.setSDK('aws-sdk');
- awsMock.mock('CloudFront.Signer', 'getSignedUrl');
- var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
- st.ok(signer);
- st.end();
+ awsMock.setSDK('aws-sdk');
+ awsMock.mock('CloudFront.Signer', 'getSignedUrl');
+ var signer = new AWS.CloudFront.Signer('key-pair-id', 'private-key');
+ st.type(signer, 'Signer');
+ st.end();
});
t.test('Setting the aws-sdk to the wrong module can cause an exception when mocking', function(st) {
| 5 | Update "Modules with multi-parameter..." test to assert on returned type | 5 | .js | test | apache-2.0 | dwyl/aws-sdk-mock |
826 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
```js
var AWS = require('aws-sdk-mock');
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
// or AWS.restore(); this will restore all the methods and services
```
You can also pass Sinon spies to the mock:
```js
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #187 from wzr1337/wzr1337-patch-1-typescript-example
docs(readme.md): Give a full example in typescript
<DFF> @@ -46,6 +46,7 @@ npm install aws-sdk-mock --save-dev
### Use in your Tests
+#### Using plain JavaScript
```js
var AWS = require('aws-sdk-mock');
@@ -70,6 +71,57 @@ AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
+#### Using TypeScript
+
+```typescript
+import * as AWSMock from "aws-sdk-mock";
+import * as AWS from "aws-sdk";
+import { GetItemInput } from "aws-sdk/clients/dynamodb";
+
+beforeAll(async (done) => {
+ //get requires env vars
+ done();
+ });
+
+describe("the module", () => {
+
+/**
+ TESTS below here
+**/
+
+ it("should mock getItem from DynamoDB", async () => {
+ // Overwriting DynamoDB.getItem()
+ AWSMock.setSDKInstance(AWS);
+ AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
+ console.log('DynamoDB', 'getItem', 'mock called');
+ callback(null, {pk: "foo", sk: "bar"});
+ })
+
+ let input:GetItemInput = { TableName: '', Key: {} };
+ const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
+ expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+
+ AWSMock.restore('DynamoDB');
+ });
+
+ it("should mock reading from DocumentClient", async () => {
+ // Overwriting DynamoDB.DocumentClient.get()
+ AWSMock.setSDKInstance(AWS);
+ AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
+ console.log('DynamoDB.DocumentClient', 'get', 'mock called');
+ callback(null, {pk: "foo", sk: "bar"});
+ })
+
+ let input:GetItemInput = { TableName: '', Key: {} };
+ const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
+ expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+
+ AWSMock.restore('DynamoDB.DocumentClient');
+ });
+});
+```
+
+#### Sinon
You can also pass Sinon spies to the mock:
```js
| 52 | Merge pull request #187 from wzr1337/wzr1337-patch-1-typescript-example | 0 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
827 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
```js
var AWS = require('aws-sdk-mock');
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
AWS.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
// or AWS.restore(); this will restore all the methods and services
```
You can also pass Sinon spies to the mock:
```js
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `ConfigError: Missing region in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #187 from wzr1337/wzr1337-patch-1-typescript-example
docs(readme.md): Give a full example in typescript
<DFF> @@ -46,6 +46,7 @@ npm install aws-sdk-mock --save-dev
### Use in your Tests
+#### Using plain JavaScript
```js
var AWS = require('aws-sdk-mock');
@@ -70,6 +71,57 @@ AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
+#### Using TypeScript
+
+```typescript
+import * as AWSMock from "aws-sdk-mock";
+import * as AWS from "aws-sdk";
+import { GetItemInput } from "aws-sdk/clients/dynamodb";
+
+beforeAll(async (done) => {
+ //get requires env vars
+ done();
+ });
+
+describe("the module", () => {
+
+/**
+ TESTS below here
+**/
+
+ it("should mock getItem from DynamoDB", async () => {
+ // Overwriting DynamoDB.getItem()
+ AWSMock.setSDKInstance(AWS);
+ AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
+ console.log('DynamoDB', 'getItem', 'mock called');
+ callback(null, {pk: "foo", sk: "bar"});
+ })
+
+ let input:GetItemInput = { TableName: '', Key: {} };
+ const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
+ expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+
+ AWSMock.restore('DynamoDB');
+ });
+
+ it("should mock reading from DocumentClient", async () => {
+ // Overwriting DynamoDB.DocumentClient.get()
+ AWSMock.setSDKInstance(AWS);
+ AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
+ console.log('DynamoDB.DocumentClient', 'get', 'mock called');
+ callback(null, {pk: "foo", sk: "bar"});
+ })
+
+ let input:GetItemInput = { TableName: '', Key: {} };
+ const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
+ expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+
+ AWSMock.restore('DynamoDB.DocumentClient');
+ });
+});
+```
+
+#### Sinon
You can also pass Sinon spies to the mock:
```js
| 52 | Merge pull request #187 from wzr1337/wzr1337-patch-1-typescript-example | 0 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
828 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
https://github.com/dwyl/learn-aws-lambda
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, "successfully put item in database");
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
awsMock.mock("S3", "getObject", Buffer.from(require("fs").readFileSync("testFile.csv")));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import * as AWSMock from "aws-sdk-mock";
import * as AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
//get requires env vars
done();
});
describe("the module", () => {
/**
TESTS below here
**/
it("should mock getItem from DynamoDB", async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: "foo", sk: "bar"});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it("should mock reading from DocumentClient", async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: "foo", sk: "bar"});
})
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `region not defined in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #223 from TastefulElk/master
Fix examples and cleanup README
<DFF> @@ -18,7 +18,7 @@ If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
-https://github.com/dwyl/learn-aws-lambda
+<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
@@ -47,18 +47,19 @@ npm install aws-sdk-mock --save-dev
### Use in your Tests
#### Using plain JavaScript
+
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
- callback(null, "successfully put item in database");
+ callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
-awsMock.mock("S3", "getObject", Buffer.from(require("fs").readFileSync("testFile.csv")));
+awsMock.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
@@ -74,47 +75,47 @@ AWS.restore('S3');
#### Using TypeScript
```typescript
-import * as AWSMock from "aws-sdk-mock";
-import * as AWS from "aws-sdk";
-import { GetItemInput } from "aws-sdk/clients/dynamodb";
+import AWSMock from 'aws-sdk-mock';
+import AWS from 'aws-sdk';
+import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
-describe("the module", () => {
+describe('the module', () => {
/**
TESTS below here
**/
- it("should mock getItem from DynamoDB", async () => {
+ it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
- callback(null, {pk: "foo", sk: "bar"});
+ callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
- expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+ expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
- it("should mock reading from DocumentClient", async () => {
+ it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
- callback(null, {pk: "foo", sk: "bar"});
- })
+ callback(null, {pk: 'foo', sk: 'bar'});
+ });
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
- expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+ expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
@@ -122,6 +123,7 @@ describe("the module", () => {
```
#### Sinon
+
You can also pass Sinon spies to the mock:
```js
@@ -146,6 +148,7 @@ assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct pa
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `region not defined in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
+
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
@@ -157,6 +160,7 @@ exports.handler = function(event, context) {
```
Example 2:
+
```js
const AWS = require('aws-sdk');
@@ -170,6 +174,7 @@ exports.handler = function(event, context) {
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
+
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
@@ -181,6 +186,7 @@ exports.handler = function(event, context) {
```
Example 2 (will work):
+
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
@@ -228,18 +234,18 @@ const csd = new AWS.CloudSearchDomain({
region: 'eu-west'
});
```
-Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
+Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
-
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
+
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
@@ -256,6 +262,7 @@ AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
+
```js
// test code
const AWSMock = require('aws-sdk-mock');
@@ -267,12 +274,12 @@ AWSMock.mock('SQS', /* ... */);
const sqs = new AWS.SQS();
```
-
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
+
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
@@ -291,14 +298,12 @@ AWS.Promise = Q.Promise;
Replaces a method on an AWS service with a replacement function or string.
-
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
-
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
@@ -311,19 +316,16 @@ Removes the mock to restore the specified AWS service
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
-
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
-
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
-
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
@@ -340,8 +342,6 @@ Explicitly set the `aws-sdk` instance to use
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
-
-
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
| 24 | Merge pull request #223 from TastefulElk/master | 24 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
829 | <NME> README.md
<BEF> # aws-sdk-mock
AWSome mocks for Javascript aws-sdk services.
[](https://travis-ci.org/dwyl/aws-sdk-mock)
[](http://codecov.io/github/dwyl/aws-sdk-mock?branch=master)
[](https://david-dm.org/dwyl/aws-sdk-mock)
[](https://david-dm.org/dwyl/aws-sdk-mock#info=devDependencies)
[](https://snyk.io/test/github/dwyl/aws-sdk-mock?targetFile=package.json)
<!-- broken see: https://github.com/dwyl/aws-sdk-mock/issues/161#issuecomment-444181270
[](https://nodei.co/npm/aws-sdk-mock/)
-->
This module was created to help test AWS Lambda functions but can be used in any situation where the AWS SDK needs to be mocked.
If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
https://github.com/dwyl/learn-aws-lambda
* [Why](#why)
* [What](#what)
* [Getting Started](#how)
* [Documentation](#documentation)
* [Background Reading](#background-reading)
## Why?
Testing your code is *essential* everywhere you need *reliability*.
Using stubs means you can prevent a specific method from being called directly. In our case we want to prevent the actual AWS services to be called while testing functions that use the AWS SDK.
## What?
Uses [Sinon.js](https://sinonjs.org/) under the hood to mock the AWS SDK services and their associated methods.
## *How*? (*Usage*)
### *install* `aws-sdk-mock` from NPM
```sh
npm install aws-sdk-mock --save-dev
```
### Use in your Tests
#### Using plain JavaScript
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
callback(null, "successfully put item in database");
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
awsMock.mock("S3", "getObject", Buffer.from(require("fs").readFileSync("testFile.csv")));
/**
TESTS
**/
AWS.restore('SNS', 'publish');
AWS.restore('DynamoDB');
AWS.restore('S3');
// or AWS.restore(); this will restore all the methods and services
```
#### Using TypeScript
```typescript
import * as AWSMock from "aws-sdk-mock";
import * as AWS from "aws-sdk";
import { GetItemInput } from "aws-sdk/clients/dynamodb";
beforeAll(async (done) => {
//get requires env vars
done();
});
describe("the module", () => {
/**
TESTS below here
**/
it("should mock getItem from DynamoDB", async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
callback(null, {pk: "foo", sk: "bar"});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
it("should mock reading from DocumentClient", async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
callback(null, {pk: "foo", sk: "bar"});
})
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
});
```
#### Sinon
You can also pass Sinon spies to the mock:
```js
const updateTableSpy = sinon.spy();
AWS.mock('DynamoDB', 'updateTable', updateTableSpy);
// Object under test
myDynamoManager.scaleDownTable();
// Assert on your Sinon spy as normal
assert.isTrue(updateTableSpy.calledOnce, 'should update dynamo table via AWS SDK');
const expectedParams = {
TableName: 'testTableName',
ProvisionedThroughput: {
ReadCapacityUnits: 1,
WriteCapacityUnits: 1
}
};
assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct parameters');
```
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `region not defined in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
exports.handler = function(event, context) {
// do something with the services e.g. sns.publish
}
```
Example 2:
```js
const AWS = require('aws-sdk');
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
}
```
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
// do something with the services e.g. sns.publish
});
}
```
Example 2 (will work):
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
const dynamoDb = AWS.DynamoDB();
someAsyncFunction(() => {
// do something with the services e.g. sns.publish
});
}
```
### Nested services
It is possible to mock nested services like `DynamoDB.DocumentClient`. Simply use this dot-notation name as the `service` parameter to the `mock()` and `restore()` methods:
```js
AWS.mock('DynamoDB.DocumentClient', 'get', function(params, callback) {
callback(null, {Item: {Key: 'Value'}});
});
```
**NB: Use caution when mocking both a nested service and its parent service.** The nested service should be mocked before and restored after its parent:
```js
// OK
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.restore('DynamoDB');
AWS.restore('DynamoDB.DocumentClient');
// Not OK
AWS.mock('DynamoDB', 'describeTable', 'message');
AWS.mock('DynamoDB.DocumentClient', 'get', 'message');
// Not OK
AWS.restore('DynamoDB.DocumentClient');
AWS.restore('DynamoDB');
```
### Don't worry about the constructor configuration
Some constructors of the aws-sdk will require you to pass through a configuration object.
```js
const csd = new AWS.CloudSearchDomain({
endpoint: 'your.end.point',
region: 'eu-west'
});
```
Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
/**
TESTS
**/
```
### Setting the `aws-sdk` object explicitly
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
```js
// test code
const AWSMock = require('aws-sdk-mock');
import AWS from 'aws-sdk';
AWSMock.setSDKInstance(AWS);
AWSMock.mock('SQS', /* ... */);
// implementation code
const sqs = new AWS.SQS();
```
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
AWS.Promise = Q.Promise;
/**
TESTS
**/
```
## Documentation
### `AWS.mock(service, method, replace)`
Replaces a method on an AWS service with a replacement function or string.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Optional | AWS service to restore - If only the service is specified, all the methods are restored |
| `method` | string | Optional | Method on AWS service to restore |
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `path` | string | Required | Path to a nested AWS SDK node module |
### `AWS.setSDKInstance(sdk)`
Explicitly set the `aws-sdk` instance to use
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
* [AWS Lambda](https://github.com/dwyl/learn-aws-lambda)
**Contributions welcome! Please submit issues or PRs if you think of anything that needs updating/improving**
<MSG> Merge pull request #223 from TastefulElk/master
Fix examples and cleanup README
<DFF> @@ -18,7 +18,7 @@ If you are *new* to Amazon WebServices Lambda
(*or need a refresher*),
please checkout our our
***Beginners Guide to AWS Lambda***:
-https://github.com/dwyl/learn-aws-lambda
+<https://github.com/dwyl/learn-aws-lambda>
* [Why](#why)
* [What](#what)
@@ -47,18 +47,19 @@ npm install aws-sdk-mock --save-dev
### Use in your Tests
#### Using plain JavaScript
+
```js
const AWS = require('aws-sdk-mock');
AWS.mock('DynamoDB', 'putItem', function (params, callback){
- callback(null, "successfully put item in database");
+ callback(null, 'successfully put item in database');
});
AWS.mock('SNS', 'publish', 'test-message');
// S3 getObject mock - return a Buffer object with file data
-awsMock.mock("S3", "getObject", Buffer.from(require("fs").readFileSync("testFile.csv")));
+awsMock.mock('S3', 'getObject', Buffer.from(require('fs').readFileSync('testFile.csv')));
/**
@@ -74,47 +75,47 @@ AWS.restore('S3');
#### Using TypeScript
```typescript
-import * as AWSMock from "aws-sdk-mock";
-import * as AWS from "aws-sdk";
-import { GetItemInput } from "aws-sdk/clients/dynamodb";
+import AWSMock from 'aws-sdk-mock';
+import AWS from 'aws-sdk';
+import { GetItemInput } from 'aws-sdk/clients/dynamodb';
beforeAll(async (done) => {
//get requires env vars
done();
});
-describe("the module", () => {
+describe('the module', () => {
/**
TESTS below here
**/
- it("should mock getItem from DynamoDB", async () => {
+ it('should mock getItem from DynamoDB', async () => {
// Overwriting DynamoDB.getItem()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB', 'getItem', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB', 'getItem', 'mock called');
- callback(null, {pk: "foo", sk: "bar"});
+ callback(null, {pk: 'foo', sk: 'bar'});
})
const input:GetItemInput = { TableName: '', Key: {} };
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
- expect(await dynamodb.getItem(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+ expect(await dynamodb.getItem(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB');
});
- it("should mock reading from DocumentClient", async () => {
+ it('should mock reading from DocumentClient', async () => {
// Overwriting DynamoDB.DocumentClient.get()
AWSMock.setSDKInstance(AWS);
AWSMock.mock('DynamoDB.DocumentClient', 'get', (params: GetItemInput, callback: Function) => {
console.log('DynamoDB.DocumentClient', 'get', 'mock called');
- callback(null, {pk: "foo", sk: "bar"});
- })
+ callback(null, {pk: 'foo', sk: 'bar'});
+ });
const input:GetItemInput = { TableName: '', Key: {} };
const client = new AWS.DynamoDB.DocumentClient({apiVersion: '2012-08-10'});
- expect(await client.get(input).promise()).toStrictEqual( { pk: 'foo', sk: 'bar' });
+ expect(await client.get(input).promise()).toStrictEqual({ pk: 'foo', sk: 'bar' });
AWSMock.restore('DynamoDB.DocumentClient');
});
@@ -122,6 +123,7 @@ describe("the module", () => {
```
#### Sinon
+
You can also pass Sinon spies to the mock:
```js
@@ -146,6 +148,7 @@ assert.isTrue(updateTableSpy.calledWith(expectedParams), 'should pass correct pa
**NB: The AWS Service needs to be initialised inside the function being tested in order for the SDK method to be mocked** e.g for an AWS Lambda function example 1 will cause an error `region not defined in config` whereas in example 2 the sdk will be successfully mocked.
Example 1:
+
```js
const AWS = require('aws-sdk');
const sns = AWS.SNS();
@@ -157,6 +160,7 @@ exports.handler = function(event, context) {
```
Example 2:
+
```js
const AWS = require('aws-sdk');
@@ -170,6 +174,7 @@ exports.handler = function(event, context) {
Also note that if you initialise an AWS service inside a callback from an async function inside the handler function, that won't work either.
Example 1 (won't work):
+
```js
exports.handler = function(event, context) {
someAsyncFunction(() => {
@@ -181,6 +186,7 @@ exports.handler = function(event, context) {
```
Example 2 (will work):
+
```js
exports.handler = function(event, context) {
const sns = AWS.SNS();
@@ -228,18 +234,18 @@ const csd = new AWS.CloudSearchDomain({
region: 'eu-west'
});
```
-Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
+Most mocking solutions with throw an `InvalidEndpoint: AWS.CloudSearchDomain requires an explicit 'endpoint' configuration option` when you try to mock this.
**aws-sdk-mock** will take care of this during mock creation so you **won't get any configuration errors**!<br>
If configurations errors still occur it means you passed wrong configuration in your implementation.
-
### Setting the `aws-sdk` module explicitly
Project structures that don't include the `aws-sdk` at the top level `node_modules` project folder will not be properly mocked. An example of this would be installing the `aws-sdk` in a nested project directory. You can get around this by explicitly setting the path to a nested `aws-sdk` module using `setSDK()`.
Example:
+
```js
const path = require('path');
const AWS = require('aws-sdk-mock');
@@ -256,6 +262,7 @@ AWS.setSDK(path.resolve('../../functions/foo/node_modules/aws-sdk'));
Due to transpiling, code written in TypeScript or ES6 may not correctly mock because the `aws-sdk` object created within `aws-sdk-mock` will not be equal to the object created within the code to test. In addition, it is sometimes convenient to have multiple SDK instances in a test. For either scenario, it is possible to pass in the SDK object directly using `setSDKInstance()`.
Example:
+
```js
// test code
const AWSMock = require('aws-sdk-mock');
@@ -267,12 +274,12 @@ AWSMock.mock('SQS', /* ... */);
const sqs = new AWS.SQS();
```
-
### Configuring promises
If your environment lacks a global Promise constructor (e.g. nodejs 0.10), you can explicitly set the promises on `aws-sdk-mock`. Set the value of `AWS.Promise` to the constructor for your chosen promise library.
Example (if Q is your promise library of choice):
+
```js
const AWS = require('aws-sdk-mock'),
Q = require('q');
@@ -291,14 +298,12 @@ AWS.Promise = Q.Promise;
Replaces a method on an AWS service with a replacement function or string.
-
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
-
### `AWS.restore(service, method)`
Removes the mock to restore the specified AWS service
@@ -311,19 +316,16 @@ Removes the mock to restore the specified AWS service
If `AWS.restore` is called without arguments (`AWS.restore()`) then all the services and their associated methods are restored
i.e. equivalent to a 'restore all' function.
-
### `AWS.remock(service, method, replace)`
Updates the `replace` method on an existing mocked service.
-
| Param | Type | Optional/Required | Description |
| :------------- | :------------- | :------------- | :------------- |
| `service` | string | Required | AWS service to mock e.g. SNS, DynamoDB, S3 |
| `method` | string | Required | method on AWS service to mock e.g. 'publish' (for SNS), 'putItem' for 'DynamoDB' |
| `replace` | string or function | Required | A string or function to replace the method |
-
### `AWS.setSDK(path)`
Explicitly set the require path for the `aws-sdk`
@@ -340,8 +342,6 @@ Explicitly set the `aws-sdk` instance to use
| :------------- | :------------- | :------------- | :------------- |
| `sdk` | object | Required | The AWS SDK object |
-
-
## Background Reading
* [Mocking using Sinon.js](http://sinonjs.org/docs/)
| 24 | Merge pull request #223 from TastefulElk/master | 24 | .md | md | apache-2.0 | dwyl/aws-sdk-mock |
830 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
public bool bInheritHandle;
}
[DllImport("kernel32.dll")]
static internal extern int CancelIo(int hFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fix for #3 and #23 the blocking CloseDevice()
<DFF> @@ -36,12 +36,18 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll")]
- static internal extern int CancelIo(int hFile);
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelIo(IntPtr hFile);
+
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelSynchronousIo(IntPtr hObject);
+
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
| 9 | Fix for #3 and #23 the blocking CloseDevice() | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
831 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
public bool bInheritHandle;
}
[DllImport("kernel32.dll")]
static internal extern int CancelIo(int hFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fix for #3 and #23 the blocking CloseDevice()
<DFF> @@ -36,12 +36,18 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll")]
- static internal extern int CancelIo(int hFile);
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelIo(IntPtr hFile);
+
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ static internal extern bool CancelSynchronousIo(IntPtr hObject);
+
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
| 9 | Fix for #3 and #23 the blocking CloseDevice() | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
832 | <NME> HidReport.cs
<BEF> using System;
namespace HidLibrary
{
public class HidReport
{
private byte _reportId;
private byte[] _data = new byte[] {};
private readonly HidDeviceData.ReadStatus _status;
public HidReport(int reportSize)
{
Array.Resize(ref _data, reportSize);
}
public HidReport(int reportSize, HidDeviceData deviceData)
{
_status = deviceData.Status;
Array.Resize(ref _data, reportSize - 1);
if ((deviceData.Data != null))
{
if (deviceData.Data.Length > 0)
{
_reportId = deviceData.Data[0];
Exists = true;
if (deviceData.Data.Length > 1)
{
var dataLength = reportSize - 1;
if (deviceData.Data.Length < reportSize - 1) dataLength = deviceData.Data.Length;
Array.Copy(deviceData.Data, 1, _data, 0, dataLength);
}
}
else Exists = false;
}
else Exists = false;
}
public bool Exists { get; private set; }
public HidDeviceData.ReadStatus ReadStatus { get { return _status; } }
public byte ReportId
{
get { return _reportId; }
set
{
_reportId = value;
Exists = true;
}
}
public byte[] Data
{
get { return _data; }
set
{
_data = value;
Exists = true;
}
}
public byte[] GetBytes()
{
byte[] data = null;
Array.Resize(ref data, _data.Length + 1);
data[0] = _reportId;
Array.Copy(_data, 0, data, 1, _data.Length);
return data;
}
}
}
<MSG> Fixes #26 reported by @marekr.
<DFF> @@ -11,7 +11,7 @@ namespace HidLibrary
public HidReport(int reportSize)
{
- Array.Resize(ref _data, reportSize);
+ Array.Resize(ref _data, reportSize - 1);
}
public HidReport(int reportSize, HidDeviceData deviceData)
| 1 | Fixes #26 reported by @marekr. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
833 | <NME> HidReport.cs
<BEF> using System;
namespace HidLibrary
{
public class HidReport
{
private byte _reportId;
private byte[] _data = new byte[] {};
private readonly HidDeviceData.ReadStatus _status;
public HidReport(int reportSize)
{
Array.Resize(ref _data, reportSize);
}
public HidReport(int reportSize, HidDeviceData deviceData)
{
_status = deviceData.Status;
Array.Resize(ref _data, reportSize - 1);
if ((deviceData.Data != null))
{
if (deviceData.Data.Length > 0)
{
_reportId = deviceData.Data[0];
Exists = true;
if (deviceData.Data.Length > 1)
{
var dataLength = reportSize - 1;
if (deviceData.Data.Length < reportSize - 1) dataLength = deviceData.Data.Length;
Array.Copy(deviceData.Data, 1, _data, 0, dataLength);
}
}
else Exists = false;
}
else Exists = false;
}
public bool Exists { get; private set; }
public HidDeviceData.ReadStatus ReadStatus { get { return _status; } }
public byte ReportId
{
get { return _reportId; }
set
{
_reportId = value;
Exists = true;
}
}
public byte[] Data
{
get { return _data; }
set
{
_data = value;
Exists = true;
}
}
public byte[] GetBytes()
{
byte[] data = null;
Array.Resize(ref data, _data.Length + 1);
data[0] = _reportId;
Array.Copy(_data, 0, data, 1, _data.Length);
return data;
}
}
}
<MSG> Fixes #26 reported by @marekr.
<DFF> @@ -11,7 +11,7 @@ namespace HidLibrary
public HidReport(int reportSize)
{
- Array.Resize(ref _data, reportSize);
+ Array.Resize(ref _data, reportSize - 1);
}
public HidReport(int reportSize, HidDeviceData deviceData)
| 1 | Fixes #26 reported by @marekr. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
834 | <NME> HidLibrary.nuspec
<BEF> <?xml version="1.0" encoding="UTF-8"?>
<package xmlns="http://schemas.microsoft.com/packaging/2010/07/nuspec.xsd">
<metadata>
<id>hidlibrary</id>
<version>$version$</version>
<title>Hid Library</title>
<authors>Mike O'Brien</authors>
<description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</description>
<releaseNotes />
<copyright />
<language>en-US</language>
<licenseUrl>https://github.com/mikeobrien/HidLibrary/blob/master/LICENSE</licenseUrl>
<projectUrl>https://github.com/mikeobrien/HidLibrary</projectUrl>
<owners>Mike O'Brien</owners>
<summary>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</summary>
<iconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</iconUrl>
<tags>usb hid</tags>
<dependencies>
<group targetFramework="net20">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net35">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
</dependencies>
</metadata>
<files>
<file src="net20\HidLibrary.*" target="lib\net20" exclude="*.xml" />
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
</files>
</package>
<MSG> Add netstandard2 target to nuspec file.
netstandard2 build is already in src\HidLibrary\HidLibrary.csproj, but this was missing in the nuget package.
<DFF> @@ -25,6 +25,10 @@
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
+ <group targetFramework="net45">
+ </group>
+ <group targetFramework="netstandard2">
+ </group>
</dependencies>
</metadata>
<files>
@@ -32,5 +36,6 @@
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
+ <file src="netstandard2\HidLibrary.*" target="lib\netstandard2" exclude="*.xml" />
</files>
</package>
\ No newline at end of file
| 5 | Add netstandard2 target to nuspec file. | 0 | .nuspec | nuspec | mit | mikeobrien/HidLibrary |
835 | <NME> HidLibrary.nuspec
<BEF> <?xml version="1.0" encoding="UTF-8"?>
<package xmlns="http://schemas.microsoft.com/packaging/2010/07/nuspec.xsd">
<metadata>
<id>hidlibrary</id>
<version>$version$</version>
<title>Hid Library</title>
<authors>Mike O'Brien</authors>
<description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</description>
<releaseNotes />
<copyright />
<language>en-US</language>
<licenseUrl>https://github.com/mikeobrien/HidLibrary/blob/master/LICENSE</licenseUrl>
<projectUrl>https://github.com/mikeobrien/HidLibrary</projectUrl>
<owners>Mike O'Brien</owners>
<summary>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</summary>
<iconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</iconUrl>
<tags>usb hid</tags>
<dependencies>
<group targetFramework="net20">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net35">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
</dependencies>
</metadata>
<files>
<file src="net20\HidLibrary.*" target="lib\net20" exclude="*.xml" />
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
</files>
</package>
<MSG> Add netstandard2 target to nuspec file.
netstandard2 build is already in src\HidLibrary\HidLibrary.csproj, but this was missing in the nuget package.
<DFF> @@ -25,6 +25,10 @@
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
+ <group targetFramework="net45">
+ </group>
+ <group targetFramework="netstandard2">
+ </group>
</dependencies>
</metadata>
<files>
@@ -32,5 +36,6 @@
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
+ <file src="netstandard2\HidLibrary.*" target="lib\netstandard2" exclude="*.xml" />
</files>
</package>
\ No newline at end of file
| 5 | Add netstandard2 target to nuspec file. | 0 | .nuspec | nuspec | mit | mikeobrien/HidLibrary |
836 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fixed WriteReport stalling problem on Windows 10 .NET4.6.2
<DFF> @@ -48,10 +48,10 @@ namespace HidLibrary
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -209,7 +209,7 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
| 3 | Fixed WriteReport stalling problem on Windows 10 .NET4.6.2 | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
837 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fixed WriteReport stalling problem on Windows 10 .NET4.6.2
<DFF> @@ -48,10 +48,10 @@ namespace HidLibrary
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -209,7 +209,7 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
| 3 | Fixed WriteReport stalling problem on Windows 10 .NET4.6.2 | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
838 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
Installation
------------
[TBD]
Props
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [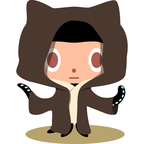](https://github.com/jwelch222) | [](https://github.com/thammer) | [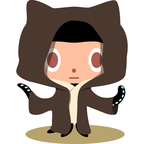](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [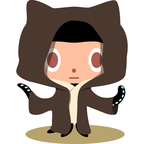](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [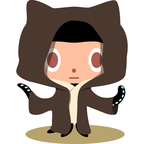](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Updated the readme
<DFF> @@ -6,7 +6,7 @@ This library enables you to enumerate and communicate with Hid compatible USB de
Installation
------------
- [TBD]
+ PM> Install-Package hidlibrary
Props
------------
| 1 | Updated the readme | 1 | .md | md | mit | mikeobrien/HidLibrary |
839 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
Installation
------------
[TBD]
Props
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [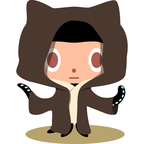](https://github.com/jwelch222) | [](https://github.com/thammer) | [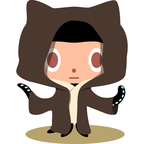](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [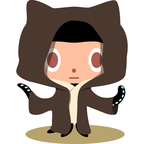](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [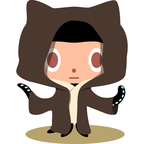](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Updated the readme
<DFF> @@ -6,7 +6,7 @@ This library enables you to enumerate and communicate with Hid compatible USB de
Installation
------------
- [TBD]
+ PM> Install-Package hidlibrary
Props
------------
| 1 | Updated the readme | 1 | .md | md | mit | mikeobrien/HidLibrary |
840 | <NME> ErrorKeyValuePairTests.java
<BEF> package com.github.structlog4j;
import static org.junit.Assert.*;
import com.github.structlog4j.samples.TestSecurityContext;
import oracle.jrockit.jfr.jdkevents.ThrowableTracer;
import org.junit.Before;
import org.junit.Test;
import org.slf4j.impl.LogEntry;
import org.slf4j.impl.LogEntry;
import org.slf4j.impl.TestLogger;
import java.util.LinkedList;
/**
* Tests for handling of invalid input
*/
public class ErrorKeyValuePairTests {
private SLogger log;
@Before
public void setup() {
log = (SLogger) SLoggerFactory.getLogger(BasicKeyValuePairTests.class);
entries = ((TestLogger)log.getSlfjLogger()).getEntries();
}
}
@Test
public void justKeyButNoValueTest() {
log.error("This is an error","just_key_but_no_value");
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),1,entries.size());
// the key with missing value was not logged
assertEquals(entries.toString(),"This is an error",entries.get(0).getMessage());
}
@Test
public void keyWithSpacesTest() {
log.error("This is an error","key with spaces",1L);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Key with spaces was passed in: key with spaces",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
assertEquals(entries.toString(),"This is an error",entries.get(1).getMessage());
}
@Test
public void keyWithSpacesRecoverTest() {
Throwable t = new RuntimeException("Important exception");
TestSecurityContext toLog = new TestSecurityContext("test_user","TEST_TENANT");
log.error("This is an error","key with spaces",1L,"good_key_that_will_be_skipped",2L,toLog,t);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Key with spaces was passed in: key with spaces",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
// the second key was ignored even though it was valid, we simply could not rely on the order any more with corrupted keys
assertEquals(entries.toString(),"This is an error userName=test_user tenantId=TEST_TENANT errorMessage=\"Important exception\"",entries.get(1).getMessage());
// validate we did not lose the exception even if it was after the key that had the error
assertTrue(entries.toString(),entries.get(1).getError().isPresent());
}
@Test
public void iToLogWithNullTest() {
IToLog toLog = new IToLog() {
@Override
public Object[] toLog() {
return null;
}
};
log.error("This is an error",toLog);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Null returned from class com.github.structlog4j.ErrorKeyValuePairTests$1.toLog()",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
assertEquals(entries.toString(),"This is an error",entries.get(1).getMessage());
}
@Test
public void iToLogWithWrongNumberOfParametersTest() {
IToLog toLog = new IToLog() {
@Override
public Object[] toLog() {
// do not return second key
return new Object[]{"key1","Value1","key2"};
}
};
log.error("This is an error",toLog);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Odd number of parameters (3) returned from class com.github.structlog4j.ErrorKeyValuePairTests$2.toLog()",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
assertEquals(entries.toString(),"This is an error",entries.get(1).getMessage());
}
@Test
public void iToLogWithKeyWithSpacesTest() {
IToLog toLog = new IToLog() {
@Override
public Object[] toLog() {
// do not return second key
return new Object[]{"key1","Value1","key with spaces","Value 2"};
}
};
log.error("This is an error",toLog);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Key with spaces was passed in from class com.github.structlog4j.ErrorKeyValuePairTests$3.toLog(): key with spaces",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
assertEquals(entries.toString(),"This is an error key1=Value1",entries.get(1).getMessage());
}
@Test
public void iToLogWithNullKeyTest() {
IToLog toLog = new IToLog() {
@Override
public Object[] toLog() {
// do not return second key
return new Object[]{"key1","Value1",null,"Value 2"};
}
};
log.error("This is an error",toLog);
// does not actually generate an error, just shows the value as empty
assertEquals(entries.toString(),2,entries.size());
assertEquals(entries.toString(),"Non-String or null key was passed in from class com.github.structlog4j.ErrorKeyValuePairTests$4.toLog(): null (null)",entries.get(0).getMessage());
// validate that despite the error we still managed to process the log entry and logged as much as we could
assertEquals(entries.toString(),"This is an error key1=Value1",entries.get(1).getMessage());
}
}
<MSG> 0.2: added mandatory context supplier
<DFF> @@ -3,7 +3,6 @@ package com.github.structlog4j;
import static org.junit.Assert.*;
import com.github.structlog4j.samples.TestSecurityContext;
-import oracle.jrockit.jfr.jdkevents.ThrowableTracer;
import org.junit.Before;
import org.junit.Test;
import org.slf4j.impl.LogEntry;
@@ -21,6 +20,8 @@ public class ErrorKeyValuePairTests {
@Before
public void setup() {
+ StructLog4J.clearMandatoryContextSupplier();
+
log = (SLogger) SLoggerFactory.getLogger(BasicKeyValuePairTests.class);
entries = ((TestLogger)log.getSlfjLogger()).getEntries();
}
| 2 | 0.2: added mandatory context supplier | 1 | .java | java | mit | jacek99/structlog4j |
841 | <NME> README.md
<BEF> # structlog4j
Structured logging API for Java, with default logback implementation
Just pass in key/value pairs as parameters (all keys **must** be String, values can be anything), e.g.
log.info("Starting processing",
"user", securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId());
which would generate a log message like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## Logging exceptions
There is no separate API for Throwable (like in SLF4j), just pass in the exception as one of the parameters (order is not
important) and we will log its root cause message and the entire exception stack trace:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
"user",securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId(),
e);
}
which would generate a log message like:
Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
...followed by regular full stack trace of the exception...
## Enforcing custom logging format per object
If you wish, any POJO in your app can implement the **IToLog** interface, e.g.
public class TenantSecurityContext implements IToLog {
private String userName;
private String tenantId;
@Override
public Object[] toLog() {
return new Object[]{"userName",getUserName(),"tenantId",getTenantId()};
}
}
Then you can just pass in the object instance directly, without the need to specify any key/value pairs:
log.info("Starting processing",securityContext);
and that will generate a log entry like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## All together now
You can mix and match all of these together without any issues:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
securityContext,
e,
"hostname", InetAddress.getLocalHost().getHostName());
}
and you would get:
Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
...followed by regular full stack trace of the exception...
## Specifying mandatory context key/value pairs
If you have specific key/value pairs that you would like logged automatically with every log entry (host name and service name are a good example),
then you just have to specify a mandatory context lambda:
StructLog4J.setMandatoryContextSupplier(() -> new Object[]{
"hostname", InetAddress.getLocalHost().getHostName(),
"serviceName","MyService"});
Now these mandatory key/value pairs will be logged automatically on **every** log entry, without the need to specify them manually.
# Logging Formats
## Key/Value Pairs
By default we log in the standard key/value pair format, e.g.:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
No extra configuration is necesary.
## JSON
If you want all messages to be logged in JSON instead, e.g.
{
"message": "Started processing",
"user": johndoe@gmail.com,
"tenantId": "SOME_TENANT_ID"
}
then you need to add the JSON formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-json:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.json.JsonFormatter;
StructLog4J.setFormatter(JsonFormatter.getInstance());
That's it.
## YAML
If you want all messages to be logged in YAML instead, e.g.
message: Started processing
user: johndoe@gmail.com
tenantId: SOME_TENANT_ID
then you need to add the YAML formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-yaml:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.yaml.YamlFormatter;
StructLog4J.setFormatter(YamlFormatter.getInstance());
That's it.
# License
MIT License.
<MSG> Updated docs
<DFF> @@ -1,2 +1,112 @@
-# structlog4j
-Structured logging API for Java, with default logback implementation
+# StructLog4J
+
+Structured logging Java, on top of the SLF4J API.
+Designed to generate easily parsable log messages for consumption in services such as LogStash, Splunk, ElasticSearch, etc.
+
+# Overview
+
+Standard Java messages look something like this
+
+ Handled 4 events while processing the import batch processing
+
+This is human readable, but very difficult to parse by code in a log aggergation serivce, as every developer
+can enter any free form text they want, in any format.
+
+Instead, we can generated a message like this
+
+ Handled events service="Import Batch" numberOfEvents=4
+
+This is very easy to parse, the message itself is just a plain description and all the context information is
+passed as separate key/value pairs (or in the future JSON, YAML, etc)
+
+# Usage
+
+StructLog4J is implemented itself on top of the SLF4J API. Therefore any application that already uses SLF4J can
+start using it immediately as it requires no other changes to existing logging configuration.
+
+Instead of the standard SLF4J Logger, you must instantiate instead the StructLog4J Logger:
+
+ private ILogger log = SLoggerFactory.getLogger(MyClass.class);
+
+The **ILogger** interface is very simple and offers just these basic methods:
+
+ public interface ILogger {
+ public void error(String message, Object...params);
+ public void warn(String message, Object...params);
+ public void info(String message, Object...params);
+ public void debug(String message, Object...params);
+ public void trace(String message, Object...params);
+ }
+
+## Logging key value pairs
+
+Just pass in key/value pairs as parameters (all keys **must** be String, values can be anything), e.g.
+
+ log.info("Starting processing","user",securityContext.getPrincipal().getName(),"tenanId",securityContext.getTenantId());
+
+which would generate a log message like:
+
+ Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
+
+## Logging exceptions
+
+There is no separate API for Throwable (like in SLF4j), just pass in the exception as one of the parameters (order is not
+important) and we will log its root cause message and the entire exception stack trace:
+
+ } catch (Exception e) {
+ log.error("Error occcurred during batch processing","user",securityContext.getPrincipal().getName(),"tenanId",securityContext.getTenantId(), e);
+ }
+
+which would generate a log message like:
+
+ Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
+ ...followed by regular full stack trace of the exception...
+
+## Enforcing custom logging format per object
+
+If you wish, any POJO in your app can implement the **IToLog** interface, e.g.
+
+ public class TenantSecurityContext implements IToLog {
+
+ private String userName;
+ private String tenantId;
+
+ @Override
+ public Object[] toLog() {
+ return new Object[]{"userName",getUserName(),"tenantId",getTenantId()};
+ }
+ }
+
+Then you can just pass in the object instance directly, without the need to specify any key/value pairs:
+
+ log.info("Starting processing",securityContext);
+
+and that will generate a log entry like:
+
+ Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
+
+## All together now
+
+You can mix and match all of these together without any issues:
+
+ } catch (Exception e) {
+ log.error("Error occcurred during batch processing",securityContext, e, "hostname", InetAddress.getLocalHost().getHostName());
+ }
+
+and you would get:
+
+ Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
+ ...followed by regular full stack trace of the exception...
+
+# License
+
+MIT License.
+
+Use it at will, just don't sue me.
+
+# Dependencies
+
+The only dependency is the SLF4 API (MIT License as well).
+
+That's it. This library is geared towards easy integration into existing applications with strict license review process.
+
| 112 | Updated docs | 2 | .md | md | mit | jacek99/structlog4j |
842 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = 0xffff;
[StructLayout(LayoutKind.Sequential)]
internal struct OVERLAPPED
{
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, [Out] byte[] lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fixes for ReadReport timeouts and lost reports after wait timeouts (#80)
1. Fixed the issue with Read timing out even when Read time out is set to 0. This boils down to using an incorrect value for WAIT_INFINITE
2. Fixed the issue with ReadReport failing after being blocked initially on a read. The Wait after readreport is also now conditional, based on whether or not the ReadReport succeeded. There is also an additional call to get overlapped result to retrieve data after a Wait
<DFF> @@ -17,7 +17,7 @@ namespace HidLibrary
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
- internal const int WAIT_INFINITE = 0xffff;
+ internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct OVERLAPPED
{
@@ -55,10 +55,13 @@ namespace HidLibrary
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
- static internal extern bool ReadFile(IntPtr hFile, [Out] byte[] lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
+ static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
+
+ [DllImport("kernel32.dll", SetLastError = true)]
+ static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
| 5 | Fixes for ReadReport timeouts and lost reports after wait timeouts (#80) | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
843 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = 0xffff;
[StructLayout(LayoutKind.Sequential)]
internal struct OVERLAPPED
{
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, [Out] byte[] lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fixes for ReadReport timeouts and lost reports after wait timeouts (#80)
1. Fixed the issue with Read timing out even when Read time out is set to 0. This boils down to using an incorrect value for WAIT_INFINITE
2. Fixed the issue with ReadReport failing after being blocked initially on a read. The Wait after readreport is also now conditional, based on whether or not the ReadReport succeeded. There is also an additional call to get overlapped result to retrieve data after a Wait
<DFF> @@ -17,7 +17,7 @@ namespace HidLibrary
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
- internal const int WAIT_INFINITE = 0xffff;
+ internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct OVERLAPPED
{
@@ -55,10 +55,13 @@ namespace HidLibrary
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
- static internal extern bool ReadFile(IntPtr hFile, [Out] byte[] lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
+ static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
+
+ [DllImport("kernel32.dll", SetLastError = true)]
+ static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
| 5 | Fixes for ReadReport timeouts and lost reports after wait timeouts (#80) | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
844 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
}
else
{
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Function was leaking handles.
I am no professional coder but this catched my eye.
Patch fixed it for me.
<DFF> @@ -439,6 +439,7 @@ namespace HidLibrary
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
+ finally { CloseDeviceIO(overlapped.EventHandle); }
}
else
{
| 1 | Function was leaking handles. I am no professional coder but this catched my eye. Patch fixed it for me. | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
845 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
}
else
{
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Function was leaking handles.
I am no professional coder but this catched my eye.
Patch fixed it for me.
<DFF> @@ -439,6 +439,7 @@ namespace HidLibrary
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
+ finally { CloseDeviceIO(overlapped.EventHandle); }
}
else
{
| 1 | Function was leaking handles. I am no professional coder but this catched my eye. Patch fixed it for me. | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
846 | <NME> build.gradle
<BEF> apply plugin: 'java'
repositories {
mavenCentral()
}
dependencies {
// Lombok is KING
compileOnly "org.projectlombok:lombok:$lombokVersion"
compile "org.slf4j:slf4j-api:$slf4jVersion"
testCompile project(":structlog4j-test")
testCompileOnly "org.projectlombok:lombok:$lombokVersion"
}
<MSG> Gradle fixes for Bintray jcenter
<DFF> @@ -1,9 +1,3 @@
-apply plugin: 'java'
-
-repositories {
- mavenCentral()
-}
-
dependencies {
// Lombok is KING
| 0 | Gradle fixes for Bintray jcenter | 6 | .gradle | gradle | mit | jacek99/structlog4j |
847 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
<MSG> Merge pull request #34 from ptrandem/master
ReadFeatureData added
<DFF> @@ -297,7 +297,7 @@ namespace HidLibrary
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
| 1 | Merge pull request #34 from ptrandem/master | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
848 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
<MSG> Merge pull request #34 from ptrandem/master
ReadFeatureData added
<DFF> @@ -297,7 +297,7 @@ namespace HidLibrary
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
| 1 | Merge pull request #34 from ptrandem/master | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
849 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [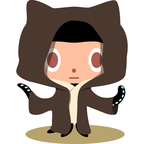](https://github.com/jwelch222) | [](https://github.com/thammer) | [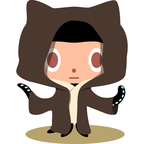](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [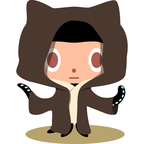](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [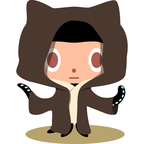](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Updated readme.
<DFF> @@ -3,6 +3,8 @@
Hid Library
=============
+[](http://www.nuget.org/packages/HidLibrary/) [](http://www.nuget.org/packages/HidLibrary/)
+
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
| 2 | Updated readme. | 0 | .md | md | mit | mikeobrien/HidLibrary |
850 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [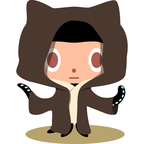](https://github.com/jwelch222) | [](https://github.com/thammer) | [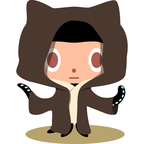](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [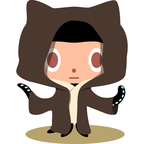](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [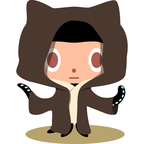](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Updated readme.
<DFF> @@ -3,6 +3,8 @@
Hid Library
=============
+[](http://www.nuget.org/packages/HidLibrary/) [](http://www.nuget.org/packages/HidLibrary/)
+
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
| 2 | Updated readme. | 0 | .md | md | mit | mikeobrien/HidLibrary |
851 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Text;
namespace HidLibrary
{
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
}
}
<MSG> Changed implementation of Product, Manufacturer, and SerialNumber getters to match the rest of the API
<DFF> @@ -1,6 +1,5 @@
using System;
using System.Runtime.InteropServices;
-using System.Text;
namespace HidLibrary
{
@@ -331,12 +330,12 @@ namespace HidLibrary
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
| 3 | Changed implementation of Product, Manufacturer, and SerialNumber getters to match the rest of the API | 4 | .cs | cs | mit | mikeobrien/HidLibrary |
852 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Text;
namespace HidLibrary
{
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
}
}
<MSG> Changed implementation of Product, Manufacturer, and SerialNumber getters to match the rest of the API
<DFF> @@ -1,6 +1,5 @@
using System;
using System.Runtime.InteropServices;
-using System.Text;
namespace HidLibrary
{
@@ -331,12 +330,12 @@ namespace HidLibrary
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
| 3 | Changed implementation of Product, Manufacturer, and SerialNumber getters to match the rest of the API | 4 | .cs | cs | mit | mikeobrien/HidLibrary |
853 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
<Authors>Mike O'Brien</Authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath="" />
<None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
</Project>
<MSG> Update copyright range
Co-authored-by: Austin Mullins <a01c12215ebbb29091dc2873291b153b83e56a40@gmail.com>
<DFF> @@ -5,7 +5,8 @@
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
- <Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
+ <Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
+
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
@@ -25,4 +26,4 @@
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
-</Project>
\ No newline at end of file
+</Project>
| 3 | Update copyright range | 2 | .csproj | csproj | mit | mikeobrien/HidLibrary |
854 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
<Authors>Mike O'Brien</Authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath="" />
<None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
</Project>
<MSG> Update copyright range
Co-authored-by: Austin Mullins <a01c12215ebbb29091dc2873291b153b83e56a40@gmail.com>
<DFF> @@ -5,7 +5,8 @@
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
- <Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
+ <Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
+
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
@@ -25,4 +26,4 @@
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
-</Project>
\ No newline at end of file
+</Project>
| 3 | Update copyright range | 2 | .csproj | csproj | mit | mikeobrien/HidLibrary |
855 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
public bool ReadProduct(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadManufacturer(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Fix #62: Increase buffer size for device string read operations.
<DFF> @@ -225,7 +225,7 @@ namespace HidLibrary
public bool ReadProduct(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -252,7 +252,7 @@ namespace HidLibrary
public bool ReadManufacturer(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -279,7 +279,7 @@ namespace HidLibrary
public bool ReadSerialNumber(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
| 3 | Fix #62: Increase buffer size for device string read operations. | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
856 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
public bool ReadProduct(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadManufacturer(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Fix #62: Increase buffer size for device string read operations.
<DFF> @@ -225,7 +225,7 @@ namespace HidLibrary
public bool ReadProduct(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -252,7 +252,7 @@ namespace HidLibrary
public bool ReadManufacturer(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -279,7 +279,7 @@ namespace HidLibrary
public bool ReadSerialNumber(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
| 3 | Fix #62: Increase buffer size for device string read operations. | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
857 | <NME> xunit.runner.visualstudio.targets
<BEF> ADDFILE
<MSG> Added missing packages because I don't have package restore setup for this build.
<DFF> @@ -0,0 +1,20 @@
+<?xml version="1.0" encoding="utf-8"?>
+<Project ToolsVersion="12.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
+ <Target Name="AddXunitTestAdapterLibs" AfterTargets="_ComputeAppxPackagePayload" BeforeTargets="_GenerateAppxManifest">
+ <ItemGroup>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)xunit.runner.visualstudio.wpa81.dll">
+ <TargetPath>xunit.runner.visualstudio.wpa81.dll</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)xunit.runner.visualstudio.wpa81.pri">
+ <TargetPath>xunit.runner.visualstudio.wpa81.pri</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)..\_common\xunit.runner.utility.dotnet.dll">
+ <TargetPath>xunit.runner.utility.dotnet.dll</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibsToRemove Include="@(AppxPackagePayload)" Condition="'%(TargetPath)' == 'xunit.runner.visualstudio.testadapter.dll'"/>
+ <xUnitVsLibsToRemove Include="@(AppxPackagePayload)" Condition="'%(TargetPath)' == 'xunit.runner.utility.desktop.dll'"/>
+ <AppxPackagePayload Remove="@(xUnitVsLibsToRemove)" />
+ <AppxPackagePayload Include="@(xUnitVsLibs)" />
+ </ItemGroup>
+ </Target>
+</Project>
| 20 | Added missing packages because I don't have package restore setup for this build. | 0 | .targets | runner | mit | mikeobrien/HidLibrary |
858 | <NME> xunit.runner.visualstudio.targets
<BEF> ADDFILE
<MSG> Added missing packages because I don't have package restore setup for this build.
<DFF> @@ -0,0 +1,20 @@
+<?xml version="1.0" encoding="utf-8"?>
+<Project ToolsVersion="12.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
+ <Target Name="AddXunitTestAdapterLibs" AfterTargets="_ComputeAppxPackagePayload" BeforeTargets="_GenerateAppxManifest">
+ <ItemGroup>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)xunit.runner.visualstudio.wpa81.dll">
+ <TargetPath>xunit.runner.visualstudio.wpa81.dll</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)xunit.runner.visualstudio.wpa81.pri">
+ <TargetPath>xunit.runner.visualstudio.wpa81.pri</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibs Include="$(MSBuildThisFileDirectory)..\_common\xunit.runner.utility.dotnet.dll">
+ <TargetPath>xunit.runner.utility.dotnet.dll</TargetPath>
+ </xUnitVsLibs>
+ <xUnitVsLibsToRemove Include="@(AppxPackagePayload)" Condition="'%(TargetPath)' == 'xunit.runner.visualstudio.testadapter.dll'"/>
+ <xUnitVsLibsToRemove Include="@(AppxPackagePayload)" Condition="'%(TargetPath)' == 'xunit.runner.utility.desktop.dll'"/>
+ <AppxPackagePayload Remove="@(xUnitVsLibsToRemove)" />
+ <AppxPackagePayload Include="@(xUnitVsLibs)" />
+ </ItemGroup>
+ </Target>
+</Project>
| 20 | Added missing packages because I don't have package restore setup for this build. | 0 | .targets | runner | mit | mikeobrien/HidLibrary |
859 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [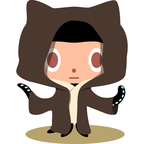](https://github.com/jwelch222) | [](https://github.com/thammer) | [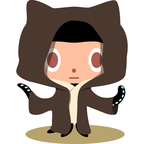](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [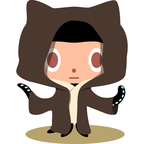](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [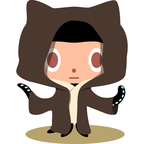](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Update readme
<DFF> @@ -3,6 +3,8 @@ Hid Library
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
+**Note:** There has been quite a bit of interest in this little library over the years but unfortunately I'm not able to support it at this time. I'm also unable to answer questions about using it with different devices. So you're on your own if you decide to use it. Please feel free to fork it or send me a pull request if you would like to contribute.
+
Installation
------------
| 2 | Update readme | 0 | .md | md | mit | mikeobrien/HidLibrary |
860 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Contributors
------------
| [](https://github.com/bwegman) | [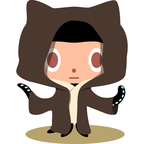](https://github.com/jwelch222) | [](https://github.com/thammer) | [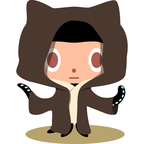](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [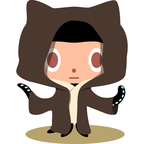](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [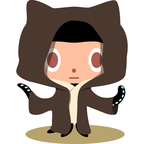](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Update readme
<DFF> @@ -3,6 +3,8 @@ Hid Library
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
+**Note:** There has been quite a bit of interest in this little library over the years but unfortunately I'm not able to support it at this time. I'm also unable to answer questions about using it with different devices. So you're on your own if you decide to use it. Please feel free to fork it or send me a pull request if you would like to contribute.
+
Installation
------------
| 2 | Update readme | 0 | .md | md | mit | mikeobrien/HidLibrary |
861 | <NME> HidLibrary.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</ProjectGuid>
<OutputType>Library</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>HidLibrary</RootNamespace>
<AssemblyName>HidLibrary</AssemblyName>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<DefineConstants>TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<PlatformTarget>AnyCPU</PlatformTarget>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="System" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'net35' AND '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="Microsoft.CSharp" />
</ItemGroup>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
<MSG> Remove things that the SDK already takes care of
<DFF> @@ -1,18 +1,7 @@
-<?xml version="1.0" encoding="utf-8"?>
-<Project Sdk="Microsoft.NET.Sdk">
+<Project Sdk="Microsoft.NET.Sdk">
+
<PropertyGroup>
- <Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
- <Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
- <ProductVersion>8.0.30703</ProductVersion>
- <SchemaVersion>2.0</SchemaVersion>
- <ProjectGuid>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</ProjectGuid>
- <OutputType>Library</OutputType>
- <AppDesignerFolder>Properties</AppDesignerFolder>
- <RootNamespace>HidLibrary</RootNamespace>
- <AssemblyName>HidLibrary</AssemblyName>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
- <FileAlignment>512</FileAlignment>
- <TargetFrameworkProfile />
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
@@ -25,50 +14,13 @@
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
- <DebugSymbols>true</DebugSymbols>
- <DebugType>full</DebugType>
- <Optimize>false</Optimize>
- <OutputPath>bin\Debug\</OutputPath>
- <DefineConstants>DEBUG;TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
- <DebugType>pdbonly</DebugType>
- <Optimize>true</Optimize>
- <OutputPath>bin\Release\</OutputPath>
- <DefineConstants>TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <PlatformTarget>AnyCPU</PlatformTarget>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
+
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="System" />
- <Reference Include="System.Data" />
- <Reference Include="System.Xml" />
- </ItemGroup>
+
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="System.Core" />
- <Reference Include="System.Xml.Linq" />
- <Reference Include="System.Data.DataSetExtensions" />
- </ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'net35' AND '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="Microsoft.CSharp" />
- </ItemGroup>
- <!-- To modify your build process, add your task inside one of the targets below and uncomment it.
- Other similar extension points exist, see Microsoft.Common.targets.
- <Target Name="BeforeBuild">
- </Target>
- <Target Name="AfterBuild">
- </Target>
- -->
-</Project>
\ No newline at end of file
+
+</Project>
| 6 | Remove things that the SDK already takes care of | 54 | .csproj | csproj | mit | mikeobrien/HidLibrary |
862 | <NME> HidLibrary.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</ProjectGuid>
<OutputType>Library</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>HidLibrary</RootNamespace>
<AssemblyName>HidLibrary</AssemblyName>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Title>Hid Library</Title>
<Authors>Mike O'Brien</Authors>
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<DefineConstants>TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<PlatformTarget>AnyCPU</PlatformTarget>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="System" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'net35' AND '$(TargetFramework)' != 'netstandard2' ">
<Reference Include="Microsoft.CSharp" />
</ItemGroup>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
<MSG> Remove things that the SDK already takes care of
<DFF> @@ -1,18 +1,7 @@
-<?xml version="1.0" encoding="utf-8"?>
-<Project Sdk="Microsoft.NET.Sdk">
+<Project Sdk="Microsoft.NET.Sdk">
+
<PropertyGroup>
- <Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
- <Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
- <ProductVersion>8.0.30703</ProductVersion>
- <SchemaVersion>2.0</SchemaVersion>
- <ProjectGuid>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</ProjectGuid>
- <OutputType>Library</OutputType>
- <AppDesignerFolder>Properties</AppDesignerFolder>
- <RootNamespace>HidLibrary</RootNamespace>
- <AssemblyName>HidLibrary</AssemblyName>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
- <FileAlignment>512</FileAlignment>
- <TargetFrameworkProfile />
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
@@ -25,50 +14,13 @@
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
- <DebugSymbols>true</DebugSymbols>
- <DebugType>full</DebugType>
- <Optimize>false</Optimize>
- <OutputPath>bin\Debug\</OutputPath>
- <DefineConstants>DEBUG;TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
- <DebugType>pdbonly</DebugType>
- <Optimize>true</Optimize>
- <OutputPath>bin\Release\</OutputPath>
- <DefineConstants>TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <PlatformTarget>AnyCPU</PlatformTarget>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
+
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="System" />
- <Reference Include="System.Data" />
- <Reference Include="System.Xml" />
- </ItemGroup>
+
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="System.Core" />
- <Reference Include="System.Xml.Linq" />
- <Reference Include="System.Data.DataSetExtensions" />
- </ItemGroup>
- <ItemGroup Condition=" '$(TargetFramework)' != 'net20' AND '$(TargetFramework)' != 'net35' AND '$(TargetFramework)' != 'netstandard2' ">
- <Reference Include="Microsoft.CSharp" />
- </ItemGroup>
- <!-- To modify your build process, add your task inside one of the targets below and uncomment it.
- Other similar extension points exist, see Microsoft.Common.targets.
- <Target Name="BeforeBuild">
- </Target>
- <Target Name="AfterBuild">
- </Target>
- -->
-</Project>
\ No newline at end of file
+
+</Project>
| 6 | Remove things that the SDK already takes care of | 54 | .csproj | csproj | mit | mikeobrien/HidLibrary |
863 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", EntryPoint = "SetupDiGetDeviceRegistryProperty")]
public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", EntryPoint = "SetupDiGetDevicePropertyW", SetLastError = true)]
public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #119 from Techsola/unicode
Use Unicode functions consistently and remove helper extension methods that were made public
<DFF> @@ -1,6 +1,8 @@
using System;
using System.Runtime.InteropServices;
+[module: DefaultCharSet(CharSet.Unicode)]
+
namespace HidLibrary
{
internal static class NativeMethods
@@ -27,16 +29,16 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
+ [DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
+ [DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -106,7 +108,7 @@ namespace HidLibrary
internal IntPtr Reserved;
}
- [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
+ [StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
@@ -124,11 +126,11 @@ namespace HidLibrary
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
- [DllImport("setupapi.dll", EntryPoint = "SetupDiGetDeviceRegistryProperty")]
- public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
+ [DllImport("setupapi.dll")]
+ public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
- [DllImport("setupapi.dll", EntryPoint = "SetupDiGetDevicePropertyW", SetLastError = true)]
- public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
+ [DllImport("setupapi.dll", SetLastError = true)]
+ public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
@@ -139,13 +141,13 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
+ [DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
- static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
+ [DllImport("setupapi.dll")]
+ static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
@@ -206,13 +208,13 @@ namespace HidLibrary
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
| 18 | Merge pull request #119 from Techsola/unicode | 16 | .cs | cs | mit | mikeobrien/HidLibrary |
864 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", EntryPoint = "SetupDiGetDeviceRegistryProperty")]
public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", EntryPoint = "SetupDiGetDevicePropertyW", SetLastError = true)]
public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll", CharSet = CharSet.Unicode)]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #119 from Techsola/unicode
Use Unicode functions consistently and remove helper extension methods that were made public
<DFF> @@ -1,6 +1,8 @@
using System;
using System.Runtime.InteropServices;
+[module: DefaultCharSet(CharSet.Unicode)]
+
namespace HidLibrary
{
internal static class NativeMethods
@@ -27,16 +29,16 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
+ [DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
+ [DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -106,7 +108,7 @@ namespace HidLibrary
internal IntPtr Reserved;
}
- [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
+ [StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
@@ -124,11 +126,11 @@ namespace HidLibrary
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
- [DllImport("setupapi.dll", EntryPoint = "SetupDiGetDeviceRegistryProperty")]
- public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
+ [DllImport("setupapi.dll")]
+ public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
- [DllImport("setupapi.dll", EntryPoint = "SetupDiGetDevicePropertyW", SetLastError = true)]
- public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
+ [DllImport("setupapi.dll", SetLastError = true)]
+ public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
@@ -139,13 +141,13 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
+ [DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
- static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
+ [DllImport("setupapi.dll")]
+ static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
@@ -206,13 +208,13 @@ namespace HidLibrary
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
- [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ [DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
| 18 | Merge pull request #119 from Techsola/unicode | 16 | .cs | cs | mit | mikeobrien/HidLibrary |
865 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Remove the intermediate byte[] heap allocation
<DFF> @@ -125,10 +125,10 @@ namespace HidLibrary
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
- public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
+ public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, SetLastError = true)]
- public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
+ public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
| 2 | Remove the intermediate byte[] heap allocation | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
866 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Remove the intermediate byte[] heap allocation
<DFF> @@ -125,10 +125,10 @@ namespace HidLibrary
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
- public static extern bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize);
+ public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, SetLastError = true)]
- public static extern bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, byte[] propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
+ public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
| 2 | Remove the intermediate byte[] heap allocation | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
867 | <NME> AssemblyInfo.cs
<BEF> ADDFILE
<MSG> Added two simple examples of communicating with a Griffin PowerMate.
<DFF> @@ -0,0 +1,36 @@
+using System.Reflection;
+using System.Runtime.CompilerServices;
+using System.Runtime.InteropServices;
+
+// General Information about an assembly is controlled through the following
+// set of attributes. Change these attribute values to modify the information
+// associated with an assembly.
+[assembly: AssemblyTitle("PowerMate")]
+[assembly: AssemblyDescription("")]
+[assembly: AssemblyConfiguration("")]
+[assembly: AssemblyCompany("")]
+[assembly: AssemblyProduct("PowerMate")]
+[assembly: AssemblyCopyright("Copyright © 2012")]
+[assembly: AssemblyTrademark("")]
+[assembly: AssemblyCulture("")]
+
+// Setting ComVisible to false makes the types in this assembly not visible
+// to COM components. If you need to access a type in this assembly from
+// COM, set the ComVisible attribute to true on that type.
+[assembly: ComVisible(false)]
+
+// The following GUID is for the ID of the typelib if this project is exposed to COM
+[assembly: Guid("83d3f5e1-4ff3-4945-8133-46e7d793ecff")]
+
+// Version information for an assembly consists of the following four values:
+//
+// Major Version
+// Minor Version
+// Build Number
+// Revision
+//
+// You can specify all the values or you can default the Build and Revision Numbers
+// by using the '*' as shown below:
+// [assembly: AssemblyVersion("1.0.*")]
+[assembly: AssemblyVersion("1.0.0.0")]
+[assembly: AssemblyFileVersion("1.0.0.0")]
| 36 | Added two simple examples of communicating with a Griffin PowerMate. | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
868 | <NME> AssemblyInfo.cs
<BEF> ADDFILE
<MSG> Added two simple examples of communicating with a Griffin PowerMate.
<DFF> @@ -0,0 +1,36 @@
+using System.Reflection;
+using System.Runtime.CompilerServices;
+using System.Runtime.InteropServices;
+
+// General Information about an assembly is controlled through the following
+// set of attributes. Change these attribute values to modify the information
+// associated with an assembly.
+[assembly: AssemblyTitle("PowerMate")]
+[assembly: AssemblyDescription("")]
+[assembly: AssemblyConfiguration("")]
+[assembly: AssemblyCompany("")]
+[assembly: AssemblyProduct("PowerMate")]
+[assembly: AssemblyCopyright("Copyright © 2012")]
+[assembly: AssemblyTrademark("")]
+[assembly: AssemblyCulture("")]
+
+// Setting ComVisible to false makes the types in this assembly not visible
+// to COM components. If you need to access a type in this assembly from
+// COM, set the ComVisible attribute to true on that type.
+[assembly: ComVisible(false)]
+
+// The following GUID is for the ID of the typelib if this project is exposed to COM
+[assembly: Guid("83d3f5e1-4ff3-4945-8133-46e7d793ecff")]
+
+// Version information for an assembly consists of the following four values:
+//
+// Major Version
+// Minor Version
+// Build Number
+// Revision
+//
+// You can specify all the values or you can default the Build and Revision Numbers
+// by using the '*' as shown below:
+// [assembly: AssemblyVersion("1.0.*")]
+[assembly: AssemblyVersion("1.0.0.0")]
+[assembly: AssemblyFileVersion("1.0.0.0")]
| 36 | Added two simple examples of communicating with a Griffin PowerMate. | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
869 | <NME> SLoggerFactory.java
<BEF> package com.github.structlog4j;
import lombok.experimental.UtilityClass;
/**
* Structured logger factory
* @author Jacek Furmankiewicz
*/
@UtilityClass
public class SLoggerFactory {
/**
* Returns logger for explicit name
*/
public ILogger getLogger(String name) {
return new SLogger(name);
}
/**
* Returns logger for source class that is generating entries
*/
public ILogger getLogger(Class<?> source) {
return new SLogger(source);
}
}
<MSG> updated docs
<DFF> @@ -11,6 +11,8 @@ public class SLoggerFactory {
/**
* Returns logger for explicit name
+ * @param name Logger name
+ * @return Structured logger
*/
public ILogger getLogger(String name) {
return new SLogger(name);
@@ -18,6 +20,8 @@ public class SLoggerFactory {
/**
* Returns logger for source class that is generating entries
+ * @param source Logger class source
+ * @return Structured logger
*/
public ILogger getLogger(Class<?> source) {
return new SLogger(source);
| 4 | updated docs | 0 | .java | java | mit | jacek99/structlog4j |
870 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
<MSG> #33 - Added GetFeature functionality
<DFF> @@ -297,7 +297,7 @@ namespace HidLibrary
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
| 1 | #33 - Added GetFeature functionality | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
871 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
<MSG> #33 - Added GetFeature functionality
<DFF> @@ -297,7 +297,7 @@ namespace HidLibrary
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
| 1 | #33 - Added GetFeature functionality | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
872 | <NME> Main.Designer.cs
<BEF> namespace TestHarness
{
partial class Main
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
this.Refresh = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
this.ErrorBeep = new System.Windows.Forms.Button();
this.Output = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// Devices
//
this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
this.Devices.Size = new System.Drawing.Size(549, 147);
this.Devices.TabIndex = 0;
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
// Refresh
//
this.Refresh.Location = new System.Drawing.Point(350, 164);
this.Refresh.Name = "Refresh";
this.Refresh.Size = new System.Drawing.Size(75, 23);
this.Refresh.TabIndex = 1;
this.Refresh.Text = "Refresh";
this.Refresh.UseVisualStyleBackColor = true;
this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
this.Scan.Location = new System.Drawing.Point(93, 164);
this.Scan.Name = "Scan";
this.Scan.Size = new System.Drawing.Size(75, 23);
this.Scan.TabIndex = 2;
this.Scan.Text = "Scan";
this.Scan.UseVisualStyleBackColor = true;
this.Scan.MouseDown += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseDown);
this.Scan.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseUp);
//
// Read
//
this.Read.Location = new System.Drawing.Point(12, 164);
this.Read.Name = "Read";
this.Read.Size = new System.Drawing.Size(75, 23);
this.Read.TabIndex = 3;
this.Read.Text = "Read";
this.Read.UseVisualStyleBackColor = true;
this.Read.Click += new System.EventHandler(this.Read_Click);
//
// SuccessBeep
//
this.SuccessBeep.Location = new System.Drawing.Point(255, 164);
this.SuccessBeep.Name = "SuccessBeep";
this.SuccessBeep.Size = new System.Drawing.Size(89, 23);
this.SuccessBeep.TabIndex = 4;
this.SuccessBeep.Text = "Success Beep";
this.SuccessBeep.UseVisualStyleBackColor = true;
this.SuccessBeep.Click += new System.EventHandler(this.SuccessBeep_Click);
//
// ErrorBeep
//
this.ErrorBeep.Location = new System.Drawing.Point(174, 164);
this.ErrorBeep.Name = "ErrorBeep";
this.ErrorBeep.Size = new System.Drawing.Size(75, 23);
this.ErrorBeep.TabIndex = 5;
this.ErrorBeep.Text = "Error Beep";
this.ErrorBeep.UseVisualStyleBackColor = true;
this.ErrorBeep.Click += new System.EventHandler(this.ErrorBeep_Click);
//
// Output
//
this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
this.Output.Size = new System.Drawing.Size(549, 274);
this.Output.TabIndex = 6;
//
// Main
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(549, 470);
this.Controls.Add(this.Output);
this.Controls.Add(this.ErrorBeep);
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
this.Controls.Add(this.Refresh);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
this.Load += new System.EventHandler(this.Main_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.ListBox Devices;
private System.Windows.Forms.Button Refresh;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
private System.Windows.Forms.Button ErrorBeep;
private System.Windows.Forms.TextBox Output;
}
}
<MSG> Merge pull request #120 from Techsola/housekeeping
Match assembly version to package version, simplify csproj, and address Visual Studio IDE complaints
<DFF> @@ -29,7 +29,7 @@
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
- this.Refresh = new System.Windows.Forms.Button();
+ this.RefreshButton = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
@@ -39,8 +39,8 @@
//
// Devices
//
- this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
@@ -49,15 +49,15 @@
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
- // Refresh
+ // RefreshButton
//
- this.Refresh.Location = new System.Drawing.Point(350, 164);
- this.Refresh.Name = "Refresh";
- this.Refresh.Size = new System.Drawing.Size(75, 23);
- this.Refresh.TabIndex = 1;
- this.Refresh.Text = "Refresh";
- this.Refresh.UseVisualStyleBackColor = true;
- this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
+ this.RefreshButton.Location = new System.Drawing.Point(350, 164);
+ this.RefreshButton.Name = "RefreshButton";
+ this.RefreshButton.Size = new System.Drawing.Size(75, 23);
+ this.RefreshButton.TabIndex = 1;
+ this.RefreshButton.Text = "Refresh";
+ this.RefreshButton.UseVisualStyleBackColor = true;
+ this.RefreshButton.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
@@ -102,9 +102,9 @@
//
// Output
//
- this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
- | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
+ | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
@@ -121,7 +121,7 @@
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
- this.Controls.Add(this.Refresh);
+ this.Controls.Add(this.RefreshButton);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
@@ -134,7 +134,7 @@
#endregion
private System.Windows.Forms.ListBox Devices;
- private System.Windows.Forms.Button Refresh;
+ private System.Windows.Forms.Button RefreshButton;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
| 16 | Merge pull request #120 from Techsola/housekeeping | 16 | .cs | Designer | mit | mikeobrien/HidLibrary |
873 | <NME> Main.Designer.cs
<BEF> namespace TestHarness
{
partial class Main
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
this.Refresh = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
this.ErrorBeep = new System.Windows.Forms.Button();
this.Output = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// Devices
//
this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
this.Devices.Size = new System.Drawing.Size(549, 147);
this.Devices.TabIndex = 0;
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
// Refresh
//
this.Refresh.Location = new System.Drawing.Point(350, 164);
this.Refresh.Name = "Refresh";
this.Refresh.Size = new System.Drawing.Size(75, 23);
this.Refresh.TabIndex = 1;
this.Refresh.Text = "Refresh";
this.Refresh.UseVisualStyleBackColor = true;
this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
this.Scan.Location = new System.Drawing.Point(93, 164);
this.Scan.Name = "Scan";
this.Scan.Size = new System.Drawing.Size(75, 23);
this.Scan.TabIndex = 2;
this.Scan.Text = "Scan";
this.Scan.UseVisualStyleBackColor = true;
this.Scan.MouseDown += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseDown);
this.Scan.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseUp);
//
// Read
//
this.Read.Location = new System.Drawing.Point(12, 164);
this.Read.Name = "Read";
this.Read.Size = new System.Drawing.Size(75, 23);
this.Read.TabIndex = 3;
this.Read.Text = "Read";
this.Read.UseVisualStyleBackColor = true;
this.Read.Click += new System.EventHandler(this.Read_Click);
//
// SuccessBeep
//
this.SuccessBeep.Location = new System.Drawing.Point(255, 164);
this.SuccessBeep.Name = "SuccessBeep";
this.SuccessBeep.Size = new System.Drawing.Size(89, 23);
this.SuccessBeep.TabIndex = 4;
this.SuccessBeep.Text = "Success Beep";
this.SuccessBeep.UseVisualStyleBackColor = true;
this.SuccessBeep.Click += new System.EventHandler(this.SuccessBeep_Click);
//
// ErrorBeep
//
this.ErrorBeep.Location = new System.Drawing.Point(174, 164);
this.ErrorBeep.Name = "ErrorBeep";
this.ErrorBeep.Size = new System.Drawing.Size(75, 23);
this.ErrorBeep.TabIndex = 5;
this.ErrorBeep.Text = "Error Beep";
this.ErrorBeep.UseVisualStyleBackColor = true;
this.ErrorBeep.Click += new System.EventHandler(this.ErrorBeep_Click);
//
// Output
//
this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
this.Output.Size = new System.Drawing.Size(549, 274);
this.Output.TabIndex = 6;
//
// Main
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(549, 470);
this.Controls.Add(this.Output);
this.Controls.Add(this.ErrorBeep);
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
this.Controls.Add(this.Refresh);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
this.Load += new System.EventHandler(this.Main_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.ListBox Devices;
private System.Windows.Forms.Button Refresh;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
private System.Windows.Forms.Button ErrorBeep;
private System.Windows.Forms.TextBox Output;
}
}
<MSG> Merge pull request #120 from Techsola/housekeeping
Match assembly version to package version, simplify csproj, and address Visual Studio IDE complaints
<DFF> @@ -29,7 +29,7 @@
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
- this.Refresh = new System.Windows.Forms.Button();
+ this.RefreshButton = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
@@ -39,8 +39,8 @@
//
// Devices
//
- this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
@@ -49,15 +49,15 @@
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
- // Refresh
+ // RefreshButton
//
- this.Refresh.Location = new System.Drawing.Point(350, 164);
- this.Refresh.Name = "Refresh";
- this.Refresh.Size = new System.Drawing.Size(75, 23);
- this.Refresh.TabIndex = 1;
- this.Refresh.Text = "Refresh";
- this.Refresh.UseVisualStyleBackColor = true;
- this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
+ this.RefreshButton.Location = new System.Drawing.Point(350, 164);
+ this.RefreshButton.Name = "RefreshButton";
+ this.RefreshButton.Size = new System.Drawing.Size(75, 23);
+ this.RefreshButton.TabIndex = 1;
+ this.RefreshButton.Text = "Refresh";
+ this.RefreshButton.UseVisualStyleBackColor = true;
+ this.RefreshButton.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
@@ -102,9 +102,9 @@
//
// Output
//
- this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
- | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
+ | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
@@ -121,7 +121,7 @@
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
- this.Controls.Add(this.Refresh);
+ this.Controls.Add(this.RefreshButton);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
@@ -134,7 +134,7 @@
#endregion
private System.Windows.Forms.ListBox Devices;
- private System.Windows.Forms.Button Refresh;
+ private System.Windows.Forms.Button RefreshButton;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
| 16 | Merge pull request #120 from Techsola/housekeeping | 16 | .cs | Designer | mit | mikeobrien/HidLibrary |
874 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #106 from vostrenkov/master
Fixed WriteReport stalling problem on Windows 10 .NET4.6.2
<DFF> @@ -48,10 +48,10 @@ namespace HidLibrary
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -209,7 +209,7 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
| 3 | Merge pull request #106 from vostrenkov/master | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
875 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #106 from vostrenkov/master
Fixed WriteReport stalling problem on Windows 10 .NET4.6.2
<DFF> @@ -48,10 +48,10 @@ namespace HidLibrary
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelSynchronousIo(IntPtr hObject);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
- [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
+ [DllImport("kernel32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, int hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
@@ -209,7 +209,7 @@ namespace HidLibrary
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
| 3 | Merge pull request #106 from vostrenkov/master | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
876 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
public bool ReadProduct(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadManufacturer(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Merge pull request #64 from amullins83/master
Fix #62: Increase buffer size for device string read operations.
<DFF> @@ -225,7 +225,7 @@ namespace HidLibrary
public bool ReadProduct(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -252,7 +252,7 @@ namespace HidLibrary
public bool ReadManufacturer(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -279,7 +279,7 @@ namespace HidLibrary
public bool ReadSerialNumber(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
| 3 | Merge pull request #64 from amullins83/master | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
877 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
public bool ReadProduct(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadManufacturer(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[64];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Merge pull request #64 from amullins83/master
Fix #62: Increase buffer size for device string read operations.
<DFF> @@ -225,7 +225,7 @@ namespace HidLibrary
public bool ReadProduct(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -252,7 +252,7 @@ namespace HidLibrary
public bool ReadManufacturer(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
@@ -279,7 +279,7 @@ namespace HidLibrary
public bool ReadSerialNumber(out byte[] data)
{
- data = new byte[64];
+ data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
| 3 | Merge pull request #64 from amullins83/master | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
878 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
}
[DllImport("hid.dll")]
static internal extern bool HidD_FlushQueue(int hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
<MSG> Merge pull request #18 from thammer/master
Examples for PowerMate and updated HidLibrary
<DFF> @@ -272,22 +272,22 @@ namespace HidLibrary
}
[DllImport("hid.dll")]
- static internal extern bool HidD_FlushQueue(int hidDeviceObject);
+ static internal extern bool HidD_FlushQueue(IntPtr hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
+ static internal extern bool HidD_GetNumInputBuffers(IntPtr hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
@@ -296,13 +296,13 @@ namespace HidLibrary
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
+ static internal extern bool HidD_SetNumInputBuffers(IntPtr hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
| 7 | Merge pull request #18 from thammer/master | 7 | .cs | cs | mit | mikeobrien/HidLibrary |
879 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
}
[DllImport("hid.dll")]
static internal extern bool HidD_FlushQueue(int hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
<MSG> Merge pull request #18 from thammer/master
Examples for PowerMate and updated HidLibrary
<DFF> @@ -272,22 +272,22 @@ namespace HidLibrary
}
[DllImport("hid.dll")]
- static internal extern bool HidD_FlushQueue(int hidDeviceObject);
+ static internal extern bool HidD_FlushQueue(IntPtr hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
+ static internal extern bool HidD_GetNumInputBuffers(IntPtr hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
@@ -296,13 +296,13 @@ namespace HidLibrary
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
+ static internal extern bool HidD_SetNumInputBuffers(IntPtr hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
| 7 | Merge pull request #18 from thammer/master | 7 | .cs | cs | mit | mikeobrien/HidLibrary |
880 | <NME> HidFastReadDevice.cs
<BEF> using System.Threading.Tasks;
namespace HidLibrary
{
public class HidFastReadDevice : HidDevice
{
internal HidFastReadDevice(string devicePath, string description = null)
: base(devicePath, description) { }
// FastRead assumes that the device is connected,
// which could cause stability issues if hardware is
// disconnected during a read
public HidDeviceData FastRead()
{
return FastRead(0);
}
public HidDeviceData FastRead(int timeout)
{
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
public void FastRead(ReadCallback callback)
{
FastRead(callback, 0);
}
public void FastRead(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(FastRead);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> FastReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(FastRead);
#if NET20 || NET35
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport FastReadReport()
{
return FastReadReport(0);
}
public HidReport FastReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, FastRead(timeout));
}
public void FastReadReport(ReadReportCallback callback)
{
FastReadReport(callback, 0);
}
public void FastReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> FastReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
#if NET20 || NET35
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
}
}
<MSG> Add NET5_0 or greater compatibility (#133)
<DFF> @@ -42,7 +42,7 @@ namespace HidLibrary
public async Task<HidDeviceData> FastReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(FastRead);
-#if NET20 || NET35
+#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
@@ -74,7 +74,7 @@ namespace HidLibrary
public async Task<HidReport> FastReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
-#if NET20 || NET35
+#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
| 2 | Add NET5_0 or greater compatibility (#133) | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
881 | <NME> HidFastReadDevice.cs
<BEF> using System.Threading.Tasks;
namespace HidLibrary
{
public class HidFastReadDevice : HidDevice
{
internal HidFastReadDevice(string devicePath, string description = null)
: base(devicePath, description) { }
// FastRead assumes that the device is connected,
// which could cause stability issues if hardware is
// disconnected during a read
public HidDeviceData FastRead()
{
return FastRead(0);
}
public HidDeviceData FastRead(int timeout)
{
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
public void FastRead(ReadCallback callback)
{
FastRead(callback, 0);
}
public void FastRead(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(FastRead);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> FastReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(FastRead);
#if NET20 || NET35
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport FastReadReport()
{
return FastReadReport(0);
}
public HidReport FastReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, FastRead(timeout));
}
public void FastReadReport(ReadReportCallback callback)
{
FastReadReport(callback, 0);
}
public void FastReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> FastReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
#if NET20 || NET35
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
}
}
<MSG> Add NET5_0 or greater compatibility (#133)
<DFF> @@ -42,7 +42,7 @@ namespace HidLibrary
public async Task<HidDeviceData> FastReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(FastRead);
-#if NET20 || NET35
+#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
@@ -74,7 +74,7 @@ namespace HidLibrary
public async Task<HidReport> FastReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(FastReadReport);
-#if NET20 || NET35
+#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
| 2 | Add NET5_0 or greater compatibility (#133) | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
882 | <NME> TestLoggerFactory.java
<BEF> ADDFILE
<MSG> JUnit tests added for key/value pairs
<DFF> @@ -0,0 +1,16 @@
+package org.slf4j.impl;
+
+import org.slf4j.ILoggerFactory;
+import org.slf4j.Logger;
+import org.slf4j.LoggerFactory;
+
+/**
+ * Test Logger Factory for tests
+ * @author Jacek Furmankiewicz
+ */
+public class TestLoggerFactory implements ILoggerFactory {
+ @Override
+ public Logger getLogger(String name) {
+ return new TestLogger(name);
+ }
+}
| 16 | JUnit tests added for key/value pairs | 0 | .java | java | mit | jacek99/structlog4j |
883 | <NME> Tests.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<PackageReference Include="Microsoft.NET.Test.Sdk" Version="15.0.0" />
<PackageReference Include="Should" Version="1.1.20" />
<PackageReference Include="xunit" Version="2.4.1" />
<PackageReference Include="xunit.runner.visualstudio" Version="2.4.1" />
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj" />
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
<Reference Include="nunit.framework">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.framework.dll</HintPath>
</Reference>
<Reference Include="nunit.mocks">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.mocks.dll</HintPath>
</Reference>
<Reference Include="pnunit.framework">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\pnunit.framework.dll</HintPath>
</Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
<MSG> Make HidDevices.EnumerateDevices internal for use with other enumerators. Add HidFastReadEnumerator. Convert NUnit tests to XUnit.
<DFF> @@ -1,5 +1,6 @@
<?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
+ <Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
@@ -13,6 +14,8 @@
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
+ <NuGetPackageImportStamp>
+ </NuGetPackageImportStamp>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
@@ -38,15 +41,6 @@
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
- <Reference Include="nunit.framework">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.framework.dll</HintPath>
- </Reference>
- <Reference Include="nunit.mocks">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.mocks.dll</HintPath>
- </Reference>
- <Reference Include="pnunit.framework">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\pnunit.framework.dll</HintPath>
- </Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
@@ -57,10 +51,23 @@
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
+ <Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
+ </Reference>
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
+ <Compile Include="HidFastReadEnumerator.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
@@ -76,6 +83,12 @@
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
+ <Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
+ <PropertyGroup>
+ <ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
+ </PropertyGroup>
+ <Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
+ </Target>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
| 22 | Make HidDevices.EnumerateDevices internal for use with other enumerators. Add HidFastReadEnumerator. Convert NUnit tests to XUnit. | 9 | .csproj | csproj | mit | mikeobrien/HidLibrary |
884 | <NME> Tests.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<PackageReference Include="Microsoft.NET.Test.Sdk" Version="15.0.0" />
<PackageReference Include="Should" Version="1.1.20" />
<PackageReference Include="xunit" Version="2.4.1" />
<PackageReference Include="xunit.runner.visualstudio" Version="2.4.1" />
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj" />
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
<Reference Include="nunit.framework">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.framework.dll</HintPath>
</Reference>
<Reference Include="nunit.mocks">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.mocks.dll</HintPath>
</Reference>
<Reference Include="pnunit.framework">
<HintPath>..\packages\NUnit.2.5.10.11092\lib\pnunit.framework.dll</HintPath>
</Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
<MSG> Make HidDevices.EnumerateDevices internal for use with other enumerators. Add HidFastReadEnumerator. Convert NUnit tests to XUnit.
<DFF> @@ -1,5 +1,6 @@
<?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
+ <Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
@@ -13,6 +14,8 @@
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
+ <NuGetPackageImportStamp>
+ </NuGetPackageImportStamp>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
@@ -38,15 +41,6 @@
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
- <Reference Include="nunit.framework">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.framework.dll</HintPath>
- </Reference>
- <Reference Include="nunit.mocks">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\nunit.mocks.dll</HintPath>
- </Reference>
- <Reference Include="pnunit.framework">
- <HintPath>..\packages\NUnit.2.5.10.11092\lib\pnunit.framework.dll</HintPath>
- </Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
@@ -57,10 +51,23 @@
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
+ <Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
+ </Reference>
+ <Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
+ <HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
+ </Reference>
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
+ <Compile Include="HidFastReadEnumerator.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
@@ -76,6 +83,12 @@
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
+ <Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
+ <PropertyGroup>
+ <ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
+ </PropertyGroup>
+ <Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
+ </Target>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
| 22 | Make HidDevices.EnumerateDevices internal for use with other enumerators. Add HidFastReadEnumerator. Convert NUnit tests to XUnit. | 9 | .csproj | csproj | mit | mikeobrien/HidLibrary |
885 | <NME> Extensions.cs
<BEF> using System.Text;
namespace HidLibrary
{
public static class Extensions
{
public static string ToUTF8String(this byte[] buffer)
{
var value = Encoding.UTF8.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
public static string ToUTF16String(this byte[] buffer)
{
var value = Encoding.Unicode.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
}
}
<MSG> Obsolete and hide unused extension methods that were unnecessarily exposed in previous releases
<DFF> @@ -1,15 +1,23 @@
-using System.Text;
+using System;
+using System.ComponentModel;
+using System.Text;
namespace HidLibrary
{
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This class will be removed in a future version.")]
public static class Extensions
{
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This method will be removed in a future version.")]
public static string ToUTF8String(this byte[] buffer)
{
var value = Encoding.UTF8.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This method will be removed in a future version.")]
public static string ToUTF16String(this byte[] buffer)
{
var value = Encoding.Unicode.GetString(buffer);
| 9 | Obsolete and hide unused extension methods that were unnecessarily exposed in previous releases | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
886 | <NME> Extensions.cs
<BEF> using System.Text;
namespace HidLibrary
{
public static class Extensions
{
public static string ToUTF8String(this byte[] buffer)
{
var value = Encoding.UTF8.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
public static string ToUTF16String(this byte[] buffer)
{
var value = Encoding.Unicode.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
}
}
<MSG> Obsolete and hide unused extension methods that were unnecessarily exposed in previous releases
<DFF> @@ -1,15 +1,23 @@
-using System.Text;
+using System;
+using System.ComponentModel;
+using System.Text;
namespace HidLibrary
{
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This class will be removed in a future version.")]
public static class Extensions
{
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This method will be removed in a future version.")]
public static string ToUTF8String(this byte[] buffer)
{
var value = Encoding.UTF8.GetString(buffer);
return value.Remove(value.IndexOf((char)0));
}
+ [EditorBrowsable(EditorBrowsableState.Never)]
+ [Obsolete("This method will be removed in a future version.")]
public static string ToUTF16String(this byte[] buffer)
{
var value = Encoding.Unicode.GetString(buffer);
| 9 | Obsolete and hide unused extension methods that were unnecessarily exposed in previous releases | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
887 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #127 from mjmatthiesen/mjmatthiesen/fix-native-methods
Fix Native Methods so that full strings are retrieved correctly.
<DFF> @@ -209,12 +209,12 @@ namespace HidLibrary
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
| 3 | Merge pull request #127 from mjmatthiesen/mjmatthiesen/fix-native-methods | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
888 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Merge pull request #127 from mjmatthiesen/mjmatthiesen/fix-native-methods
Fix Native Methods so that full strings are retrieved correctly.
<DFF> @@ -209,12 +209,12 @@ namespace HidLibrary
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
| 3 | Merge pull request #127 from mjmatthiesen/mjmatthiesen/fix-native-methods | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
889 | <NME> README.md
<BEF> 
Structured logging Java, on top of the SLF4J API.
Designed to generate easily parsable log messages for consumption in services such as LogStash, Splunk, ElasticSearch, etc.
## Adding to your project
The artifacts for this library are published to the popular Bintray JCenter Maven repository.
### Gradle
repositories {
jcenter()
}
compile 'structlog4j:structlog4j-api:1.0.0'
// Optional JSON formatter
compile 'structlog4j:structlog4j-json:1.0.0'
// Optional YAML formatter
compile 'structlog4j:structlog4j-yaml:1.0.0'
### Maven
<repositories>
<repository>
<id>jcenter</id>
<url>http://jcenter.bintray.com</url>
</repository>
</repositories>
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-api</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
<!-- Optional JSON formatter -->
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-json</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
<!-- Optional YAML formatter -->
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-yaml</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
# Overview
} catch (Exception e) {
log.error("Error occcurred during batch processing",
"user",securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId(),
e);
}
which would generate a log message like:
Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
...followed by regular full stack trace of the exception...
## Enforcing custom logging format per object
{
"message": "Processed flight records",
"recordCount": 23,
"airlineCode": "UA",
"flightNumber": "1234",
"airlineName": "United"
}
or in YAML (if using our YAML formatter):
message: Processed flight records
recordCount: 23
airlineCode: UA
flightNumber: 1234
airlineName: United
This is very easy to parse, the message itself is just a plain description and all the context information is
passed as separate key/value pairs.
When this type of log entry is forwarded to a log aggregation service (such as Splunk, Logstash, etc) it is trivial to parse it and extract context information from it.
Thus, it is very easy to perform log analytics, which are criticial to many open applications (especially multi-tenant cloud applications).
# Usage
StructLog4J is implemented itself on top of the SLF4J API. Therefore any application that already uses SLF4J can
} catch (Exception e) {
log.error("Error occcurred during batch processing",
securityContext,
e,
"hostname", InetAddress.getLocalHost().getHostName());
}
and you would get:
Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
...followed by regular full stack trace of the exception...
# License
}
## Logging key value pairs
Just pass in key/value pairs as parameters (all keys **must** be String, values can be anything), e.g.
log.info("Starting processing",
"user", securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId());
which would generate a log message like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## Logging exceptions
There is no separate API for Throwable (like in SLF4j), just pass in the exception as one of the parameters (order is not
important) and we will log its root cause message and the entire exception stack trace:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
"user",securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId(),
e);
}
which would generate a log message like:
Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
...followed by regular full stack trace of the exception...
## Enforcing custom logging format per object
If you wish, any POJO in your app can implement the **IToLog** interface, e.g.
public class TenantSecurityContext implements IToLog {
private String userName;
private String tenantId;
@Override
public Object[] toLog() {
return new Object[]{"userName",getUserName(),"tenantId",getTenantId()};
}
}
Then you can just pass in the object instance directly, without the need to specify any key/value pairs:
log.info("Starting processing",securityContext);
and that will generate a log entry like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## All together now
You can mix and match all of these together without any issues:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
securityContext,
e,
"hostname", InetAddress.getLocalHost().getHostName());
}
and you would get:
Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
...followed by regular full stack trace of the exception...
## Specifying mandatory context key/value pairs
If you have specific key/value pairs that you would like logged automatically with every log entry (host name and service name are a good example),
then you just have to specify a mandatory context lambda:
StructLog4J.setMandatoryContextSupplier(() -> new Object[]{
"hostname", InetAddress.getLocalHost().getHostName(),
"serviceName","MyService"});
Now these mandatory key/value pairs will be logged automatically on **every** log entry, without the need to specify them manually.
# Logging Formats
## Key/Value Pairs
By default we log in the standard key/value pair format, e.g.:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
No extra configuration is necesary.
## JSON
If you want all messages to be logged in JSON instead, e.g.
{
"message": "Started processing",
"user": johndoe@gmail.com,
"tenantId": "SOME_TENANT_ID"
}
then you need to add the JSON formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-json:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.json.JsonFormatter;
StructLog4J.setFormatter(JsonFormatter.getInstance());
That's it.
## YAML
If you want all messages to be logged in YAML instead, e.g.
message: Started processing
user: johndoe@gmail.com
tenantId: SOME_TENANT_ID
then you need to add the YAML formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-yaml:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.yaml.YamlFormatter;
StructLog4J.setFormatter(YamlFormatter.getInstance());
That's it.
# License
MIT License.
<MSG> Updated docs
<DFF> @@ -57,13 +57,13 @@ important) and we will log its root cause message and the entire exception stack
} catch (Exception e) {
log.error("Error occcurred during batch processing",
"user",securityContext.getPrincipal().getName(),
- "tenanId",securityContext.getTenantId(),
+ "tenanId",securityContext.getTenantId(),
e);
}
which would generate a log message like:
- Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
+ Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You"
...followed by regular full stack trace of the exception...
## Enforcing custom logging format per object
@@ -95,14 +95,14 @@ You can mix and match all of these together without any issues:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
- securityContext,
- e,
+ securityContext,
+ e,
"hostname", InetAddress.getLocalHost().getHostName());
}
and you would get:
- Error occurreded during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
+ Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
...followed by regular full stack trace of the exception...
# License
| 5 | Updated docs | 5 | .md | md | mit | jacek99/structlog4j |
890 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace HidLibrary
{
public delegate void InsertedEventHandler();
public delegate void RemovedEventHandler();
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
void Read(ReadCallback callback);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadFeatureData(out byte[] data, byte reportId = 0);
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data, int timeout);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
bool WriteFeatureData(byte[] data);
}
}
<MSG> Merge pull request #51 from sblakemore/async_interface
Add Async methods to IHidDevice interface
<DFF> @@ -1,7 +1,5 @@
using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
+using System.Threading.Tasks;
namespace HidLibrary
{
@@ -51,10 +49,18 @@ namespace HidLibrary
void Read(ReadCallback callback);
+ void Read(ReadCallback callback, int timeout);
+
+ Task<HidDeviceData> ReadAsync(int timeout = 0);
+
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
+ void ReadReport(ReadReportCallback callback, int timeout);
+
+ Task<HidReport> ReadReportAsync(int timeout = 0);
+
HidReport ReadReport(int timeout);
HidReport ReadReport();
@@ -72,12 +78,20 @@ namespace HidLibrary
bool Write(byte[] data, int timeout);
+ void Write(byte[] data, WriteCallback callback, int timeout);
+
+ Task<bool> WriteAsync(byte[] data, int timeout = 0);
+
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
+ void WriteReport(HidReport report, WriteCallback callback, int timeout);
+
+ Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
+
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
| 17 | Merge pull request #51 from sblakemore/async_interface | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
891 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace HidLibrary
{
public delegate void InsertedEventHandler();
public delegate void RemovedEventHandler();
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
void Read(ReadCallback callback);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadFeatureData(out byte[] data, byte reportId = 0);
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data, int timeout);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
bool WriteFeatureData(byte[] data);
}
}
<MSG> Merge pull request #51 from sblakemore/async_interface
Add Async methods to IHidDevice interface
<DFF> @@ -1,7 +1,5 @@
using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
+using System.Threading.Tasks;
namespace HidLibrary
{
@@ -51,10 +49,18 @@ namespace HidLibrary
void Read(ReadCallback callback);
+ void Read(ReadCallback callback, int timeout);
+
+ Task<HidDeviceData> ReadAsync(int timeout = 0);
+
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
+ void ReadReport(ReadReportCallback callback, int timeout);
+
+ Task<HidReport> ReadReportAsync(int timeout = 0);
+
HidReport ReadReport(int timeout);
HidReport ReadReport();
@@ -72,12 +78,20 @@ namespace HidLibrary
bool Write(byte[] data, int timeout);
+ void Write(byte[] data, WriteCallback callback, int timeout);
+
+ Task<bool> WriteAsync(byte[] data, int timeout = 0);
+
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
+ void WriteReport(HidReport report, WriteCallback callback, int timeout);
+
+ Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
+
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
| 17 | Merge pull request #51 from sblakemore/async_interface | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
892 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
[](http://www.nuget.org/packages/HidLibrary/) [](http://build.mikeobrien.net/viewType.html?buildTypeId=hidlibrary&guest=1)
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Added contributors to the readme.
<DFF> @@ -11,13 +11,28 @@ Installation
------------
PM> Install-Package hidlibrary
-
+
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
-| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
+| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
+
+Contributors
+------------
+
+| [](https://github.com/bwegman) | [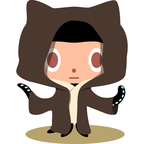](https://github.com/jwelch222) | [](https://github.com/thammer) | [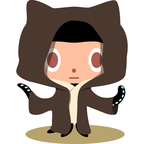](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
+|:---:|:---:|:---:|:---:|:---:|
+| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
+
+| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
+|:---:|:---:|:---:|:---:|:---:|
+| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
+
+| [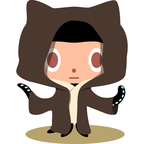](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [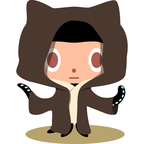](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
+|:---:|:---:|:---:|:---:|:---:|
+| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
| 17 | Added contributors to the readme. | 2 | .md | md | mit | mikeobrien/HidLibrary |
893 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
[](http://www.nuget.org/packages/HidLibrary/) [](http://build.mikeobrien.net/viewType.html?buildTypeId=hidlibrary&guest=1)
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Added contributors to the readme.
<DFF> @@ -11,13 +11,28 @@ Installation
------------
PM> Install-Package hidlibrary
-
+
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
-| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
+| [Mike O'Brien](https://github.com/mikeobrien) | [Austin Mullins](https://github.com/amullins83) |
+
+Contributors
+------------
+
+| [](https://github.com/bwegman) | [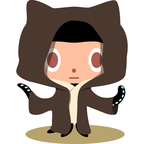](https://github.com/jwelch222) | [](https://github.com/thammer) | [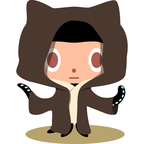](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
+|:---:|:---:|:---:|:---:|:---:|
+| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
+
+| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
+|:---:|:---:|:---:|:---:|:---:|
+| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
+
+| [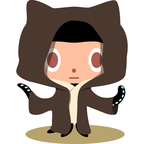](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [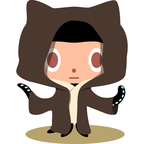](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
+|:---:|:---:|:---:|:---:|:---:|
+| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
| 17 | Added contributors to the readme. | 2 | .md | md | mit | mikeobrien/HidLibrary |
894 | <NME> Tests.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{5AFA775C-12DA-453A-8611-11E9FAEE7A8B}</ProjectGuid>
<OutputType>Library</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>Tests</RootNamespace>
<AssemblyName>Tests</AssemblyName>
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
<NuGetPackageImportStamp>
</NuGetPackageImportStamp>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<DefineConstants>TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<ItemGroup>
<Reference Include="NSubstitute, Version=1.7.2.0, Culture=neutral, PublicKeyToken=92dd2e9066daa5ca, processorArchitecture=MSIL">
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
<Reference Include="System" />
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
<Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
</Reference>
<Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
</Reference>
<Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
</Reference>
<Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
</Reference>
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
<Compile Include="HidFastReadEnumerator.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
<None Include="packages.config" />
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
<Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<ItemGroup>
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
<PropertyGroup>
<ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
</PropertyGroup>
<Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
</Target>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<MSG> Target Net Standard 2.0 (#105)
* Added net standard 2.0 target to HidLibrary
* Moved nuspec metadata into csproj file (msbuild can now diretly create nupkg packages)
* Move test project into updated csproj format.
Also, fixed one warning about public func that is not a [Fact].
<DFF> @@ -1,99 +1,15 @@
<?xml version="1.0" encoding="utf-8"?>
-<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
- <Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
+<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
- <Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
- <Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
- <ProductVersion>8.0.30703</ProductVersion>
- <SchemaVersion>2.0</SchemaVersion>
- <ProjectGuid>{5AFA775C-12DA-453A-8611-11E9FAEE7A8B}</ProjectGuid>
- <OutputType>Library</OutputType>
- <AppDesignerFolder>Properties</AppDesignerFolder>
- <RootNamespace>Tests</RootNamespace>
- <AssemblyName>Tests</AssemblyName>
- <TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
- <FileAlignment>512</FileAlignment>
- <TargetFrameworkProfile />
- <NuGetPackageImportStamp>
- </NuGetPackageImportStamp>
+ <TargetFramework>net46</TargetFramework>
</PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
- <DebugSymbols>true</DebugSymbols>
- <DebugType>full</DebugType>
- <Optimize>false</Optimize>
- <OutputPath>bin\Debug\</OutputPath>
- <DefineConstants>DEBUG;TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
- <DebugType>pdbonly</DebugType>
- <Optimize>true</Optimize>
- <OutputPath>bin\Release\</OutputPath>
- <DefineConstants>TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <ItemGroup>
- <Reference Include="NSubstitute, Version=1.7.2.0, Culture=neutral, PublicKeyToken=92dd2e9066daa5ca, processorArchitecture=MSIL">
- <SpecificVersion>False</SpecificVersion>
- <HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
- </Reference>
- <Reference Include="Should">
- <HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
- </Reference>
- <Reference Include="System" />
- <Reference Include="System.Core" />
- <Reference Include="System.Xml.Linq" />
- <Reference Include="System.Data.DataSetExtensions" />
- <Reference Include="Microsoft.CSharp" />
- <Reference Include="System.Data" />
- <Reference Include="System.Xml" />
- <Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
- </Reference>
- <Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
- </Reference>
- <Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
- </Reference>
- <Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
- </Reference>
- </ItemGroup>
- <ItemGroup>
- <Compile Include="HidEnumerator.cs" />
- <Compile Include="Hid.cs" />
- <Compile Include="HidFastReadEnumerator.cs" />
- <Compile Include="Properties\AssemblyInfo.cs" />
- </ItemGroup>
- <ItemGroup>
- <None Include="packages.config" />
- </ItemGroup>
<ItemGroup>
- <ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
- <Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
- <Name>HidLibrary</Name>
- </ProjectReference>
+ <PackageReference Include="Microsoft.NET.Test.Sdk" Version="15.0.0" />
+ <PackageReference Include="Should" Version="1.1.20" />
+ <PackageReference Include="xunit" Version="2.4.1" />
+ <PackageReference Include="xunit.runner.visualstudio" Version="2.4.1" />
</ItemGroup>
<ItemGroup>
- <Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
+ <ProjectReference Include="..\HidLibrary\HidLibrary.csproj" />
</ItemGroup>
- <Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
- <Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
- <PropertyGroup>
- <ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
- </PropertyGroup>
- <Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
- </Target>
- <!-- To modify your build process, add your task inside one of the targets below and uncomment it.
- Other similar extension points exist, see Microsoft.Common.targets.
- <Target Name="BeforeBuild">
- </Target>
- <Target Name="AfterBuild">
- </Target>
- -->
-</Project>
\ No newline at end of file
+</Project>
| 8 | Target Net Standard 2.0 (#105) | 92 | .csproj | csproj | mit | mikeobrien/HidLibrary |
895 | <NME> Tests.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{5AFA775C-12DA-453A-8611-11E9FAEE7A8B}</ProjectGuid>
<OutputType>Library</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>Tests</RootNamespace>
<AssemblyName>Tests</AssemblyName>
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<FileAlignment>512</FileAlignment>
<TargetFrameworkProfile />
<NuGetPackageImportStamp>
</NuGetPackageImportStamp>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<DefineConstants>TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<ItemGroup>
<Reference Include="NSubstitute, Version=1.7.2.0, Culture=neutral, PublicKeyToken=92dd2e9066daa5ca, processorArchitecture=MSIL">
<SpecificVersion>False</SpecificVersion>
<HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
</Reference>
<Reference Include="Should">
<HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
</Reference>
<Reference Include="System" />
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Xml" />
<Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
</Reference>
<Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
</Reference>
<Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
</Reference>
<Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
<HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
</Reference>
</ItemGroup>
<ItemGroup>
<Compile Include="HidEnumerator.cs" />
<Compile Include="Hid.cs" />
<Compile Include="HidFastReadEnumerator.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<ItemGroup>
<None Include="packages.config" />
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
<Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<ItemGroup>
<Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
<PropertyGroup>
<ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
</PropertyGroup>
<Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
</Target>
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<MSG> Target Net Standard 2.0 (#105)
* Added net standard 2.0 target to HidLibrary
* Moved nuspec metadata into csproj file (msbuild can now diretly create nupkg packages)
* Move test project into updated csproj format.
Also, fixed one warning about public func that is not a [Fact].
<DFF> @@ -1,99 +1,15 @@
<?xml version="1.0" encoding="utf-8"?>
-<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
- <Import Project="..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props" Condition="Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" />
+<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
- <Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
- <Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform>
- <ProductVersion>8.0.30703</ProductVersion>
- <SchemaVersion>2.0</SchemaVersion>
- <ProjectGuid>{5AFA775C-12DA-453A-8611-11E9FAEE7A8B}</ProjectGuid>
- <OutputType>Library</OutputType>
- <AppDesignerFolder>Properties</AppDesignerFolder>
- <RootNamespace>Tests</RootNamespace>
- <AssemblyName>Tests</AssemblyName>
- <TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
- <FileAlignment>512</FileAlignment>
- <TargetFrameworkProfile />
- <NuGetPackageImportStamp>
- </NuGetPackageImportStamp>
+ <TargetFramework>net46</TargetFramework>
</PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' ">
- <DebugSymbols>true</DebugSymbols>
- <DebugType>full</DebugType>
- <Optimize>false</Optimize>
- <OutputPath>bin\Debug\</OutputPath>
- <DefineConstants>DEBUG;TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' ">
- <DebugType>pdbonly</DebugType>
- <Optimize>true</Optimize>
- <OutputPath>bin\Release\</OutputPath>
- <DefineConstants>TRACE</DefineConstants>
- <ErrorReport>prompt</ErrorReport>
- <WarningLevel>4</WarningLevel>
- <Prefer32Bit>false</Prefer32Bit>
- </PropertyGroup>
- <ItemGroup>
- <Reference Include="NSubstitute, Version=1.7.2.0, Culture=neutral, PublicKeyToken=92dd2e9066daa5ca, processorArchitecture=MSIL">
- <SpecificVersion>False</SpecificVersion>
- <HintPath>..\packages\NSubstitute.1.7.2.0\lib\NET45\NSubstitute.dll</HintPath>
- </Reference>
- <Reference Include="Should">
- <HintPath>..\packages\Should.1.1.12.0\lib\Should.dll</HintPath>
- </Reference>
- <Reference Include="System" />
- <Reference Include="System.Core" />
- <Reference Include="System.Xml.Linq" />
- <Reference Include="System.Data.DataSetExtensions" />
- <Reference Include="Microsoft.CSharp" />
- <Reference Include="System.Data" />
- <Reference Include="System.Xml" />
- <Reference Include="xunit.abstractions, Version=2.0.0.0, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.abstractions.2.0.0\lib\net35\xunit.abstractions.dll</HintPath>
- </Reference>
- <Reference Include="xunit.assert, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.assert.2.1.0\lib\dotnet\xunit.assert.dll</HintPath>
- </Reference>
- <Reference Include="xunit.core, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.extensibility.core.2.1.0\lib\dotnet\xunit.core.dll</HintPath>
- </Reference>
- <Reference Include="xunit.execution.desktop, Version=2.1.0.3179, Culture=neutral, PublicKeyToken=8d05b1bb7a6fdb6c, processorArchitecture=MSIL">
- <HintPath>..\packages\xunit.extensibility.execution.2.1.0\lib\net45\xunit.execution.desktop.dll</HintPath>
- </Reference>
- </ItemGroup>
- <ItemGroup>
- <Compile Include="HidEnumerator.cs" />
- <Compile Include="Hid.cs" />
- <Compile Include="HidFastReadEnumerator.cs" />
- <Compile Include="Properties\AssemblyInfo.cs" />
- </ItemGroup>
- <ItemGroup>
- <None Include="packages.config" />
- </ItemGroup>
<ItemGroup>
- <ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
- <Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
- <Name>HidLibrary</Name>
- </ProjectReference>
+ <PackageReference Include="Microsoft.NET.Test.Sdk" Version="15.0.0" />
+ <PackageReference Include="Should" Version="1.1.20" />
+ <PackageReference Include="xunit" Version="2.4.1" />
+ <PackageReference Include="xunit.runner.visualstudio" Version="2.4.1" />
</ItemGroup>
<ItemGroup>
- <Service Include="{82A7F48D-3B50-4B1E-B82E-3ADA8210C358}" />
+ <ProjectReference Include="..\HidLibrary\HidLibrary.csproj" />
</ItemGroup>
- <Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
- <Target Name="EnsureNuGetPackageBuildImports" BeforeTargets="PrepareForBuild">
- <PropertyGroup>
- <ErrorText>This project references NuGet package(s) that are missing on this computer. Use NuGet Package Restore to download them. For more information, see http://go.microsoft.com/fwlink/?LinkID=322105. The missing file is {0}.</ErrorText>
- </PropertyGroup>
- <Error Condition="!Exists('..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props')" Text="$([System.String]::Format('$(ErrorText)', '..\packages\xunit.runner.visualstudio.2.1.0\build\net20\xunit.runner.visualstudio.props'))" />
- </Target>
- <!-- To modify your build process, add your task inside one of the targets below and uncomment it.
- Other similar extension points exist, see Microsoft.Common.targets.
- <Target Name="BeforeBuild">
- </Target>
- <Target Name="AfterBuild">
- </Target>
- -->
-</Project>
\ No newline at end of file
+</Project>
| 8 | Target Net Standard 2.0 (#105) | 92 | .csproj | csproj | mit | mikeobrien/HidLibrary |
896 | <NME> README.md
<BEF> 
Structured logging Java, on top of the SLF4J API.
Designed to generate easily parsable log messages for consumption in services such as LogStash, Splunk, ElasticSearch, etc.
## Adding to your project
The artifacts for this library are published to the popular Bintray JCenter Maven repository.
### Gradle
repositories {
jcenter()
}
compile 'structlog4j:structlog4j-api:1.0.0'
// Optional JSON formatter
compile 'structlog4j:structlog4j-json:1.0.0'
// Optional YAML formatter
compile 'structlog4j:structlog4j-yaml:1.0.0'
### Maven
<repositories>
<repository>
<id>jcenter</id>
<url>http://jcenter.bintray.com</url>
</repository>
</repositories>
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-api</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
<!-- Optional JSON formatter -->
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-json</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
<!-- Optional YAML formatter -->
<dependency>
<groupId>structlog4j</groupId>
<artifactId>structlog4j-yaml</artifactId>
<version>1.0.0</version>
<type>pom</type>
</dependency>
# Overview
Standard Java messages look something like this
Processed 23 flight records for flight UA1234 for airline United
This is human readable, but very difficult to parse by code in a log aggregation serivce, as every developer can enter any free form text they want, in any format.
Instead, we can generate a message like this
Processed flight records recordCount=23 airlineCode=UA flightNumber=1234 airlineName=United
or as JSON (if using our JSON formatter):
{
"message": "Processed flight records",
"recordCount": 23,
"airlineCode": "UA",
"flightNumber": "1234",
"airlineName": "United"
}
or in YAML (if using our YAML formatter):
message: Processed flight records
recordCount: 23
airlineCode: UA
flightNumber: 1234
airlineName: United
This is very easy to parse, the message itself is just a plain description and all the context information is
passed as separate key/value pairs.
When this type of log entry is forwarded to a log aggregation service (such as Splunk, Logstash, etc) it is trivial to parse it and extract context information from it.
Thus, it is very easy to perform log analytics, which are criticial to many open applications (especially multi-tenant cloud applications).
# Usage
StructLog4J is implemented itself on top of the SLF4J API. Therefore any application that already uses SLF4J can
start using it immediately as it requires no other changes to existing logging configuration.
Instead of the standard SLF4J Logger, you must instantiate the StructLog4J Logger:
private ILogger log = SLoggerFactory.getLogger(MyClass.class);
The **ILogger** interface is very simple and offers just these basic methods:
public interface ILogger {
public void error(String message, Object...params);
public void warn(String message, Object...params);
public void info(String message, Object...params);
public void debug(String message, Object...params);
public void trace(String message, Object...params);
}
## Logging key value pairs
Just pass in key/value pairs as parameters (all keys **must** be String, values can be anything), e.g.
log.info("Starting processing",
"user", securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId());
which would generate a log message like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## Logging exceptions
There is no separate API for Throwable (like in SLF4j), just pass in the exception as one of the parameters (order is not
important) and we will log its root cause message and the entire exception stack trace:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
"user",securityContext.getPrincipal().getName(),
"tenanId",securityContext.getTenantId(),
e);
}
## Specifying mandatory context key/value pairs
If you have specific key/value pairs that you would like logged automatically with every log entry (host nanem and service name are a good example),
then you just have to specify a mandatory context lambda:
StructLog4J.setMandatoryContextSupplier(() -> new Object[]{
If you wish, any POJO in your app can implement the **IToLog** interface, e.g.
public class TenantSecurityContext implements IToLog {
private String userName;
private String tenantId;
@Override
public Object[] toLog() {
return new Object[]{"userName",getUserName(),"tenantId",getTenantId()};
}
}
Then you can just pass in the object instance directly, without the need to specify any key/value pairs:
log.info("Starting processing",securityContext);
and that will generate a log entry like:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
## All together now
You can mix and match all of these together without any issues:
} catch (Exception e) {
log.error("Error occcurred during batch processing",
securityContext,
e,
"hostname", InetAddress.getLocalHost().getHostName());
}
and you would get:
Error occurred during batch processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID errorMessage="ORA-14094: Oracle Hates You" hostname=DEV_SERVER1
...followed by regular full stack trace of the exception...
## Specifying mandatory context key/value pairs
If you have specific key/value pairs that you would like logged automatically with every log entry (host name and service name are a good example),
then you just have to specify a mandatory context lambda:
StructLog4J.setMandatoryContextSupplier(() -> new Object[]{
"hostname", InetAddress.getLocalHost().getHostName(),
"serviceName","MyService"});
Now these mandatory key/value pairs will be logged automatically on **every** log entry, without the need to specify them manually.
# Logging Formats
## Key/Value Pairs
By default we log in the standard key/value pair format, e.g.:
Starting processing user=johndoe@gmail.com tenantId=SOME_TENANT_ID
No extra configuration is necesary.
## JSON
If you want all messages to be logged in JSON instead, e.g.
{
"message": "Started processing",
"user": johndoe@gmail.com,
"tenantId": "SOME_TENANT_ID"
}
then you need to add the JSON formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-json:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.json.JsonFormatter;
StructLog4J.setFormatter(JsonFormatter.getInstance());
That's it.
## YAML
If you want all messages to be logged in YAML instead, e.g.
message: Started processing
user: johndoe@gmail.com
tenantId: SOME_TENANT_ID
then you need to add the YAML formatter library as a dependency (where $version is the current library version):
compile 'structlog4j:structlog4j-yaml:$version'
and then just execute the following code in the startup main() of your application:
import com.github.structlog4j.yaml.YamlFormatter;
StructLog4J.setFormatter(YamlFormatter.getInstance());
That's it.
# License
MIT License.
<MSG> fixed up docs
<DFF> @@ -135,7 +135,7 @@ and you would get:
## Specifying mandatory context key/value pairs
-If you have specific key/value pairs that you would like logged automatically with every log entry (host nanem and service name are a good example),
+If you have specific key/value pairs that you would like logged automatically with every log entry (host name and service name are a good example),
then you just have to specify a mandatory context lambda:
StructLog4J.setMandatoryContextSupplier(() -> new Object[]{
| 1 | fixed up docs | 1 | .md | md | mit | jacek99/structlog4j |
897 | <NME> HidDevices.cs
<BEF> using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Runtime.InteropServices;
namespace HidLibrary
{
public class HidDevices
{
private static Guid _hidClassGuid = Guid.Empty;
public static bool IsConnected(string devicePath)
{
return EnumerateDevices().Any(x => x.Path == devicePath);
}
public static HidDevice GetDevice(string devicePath)
{
return Enumerate(devicePath).FirstOrDefault();
}
public static IEnumerable<HidDevice> Enumerate()
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(string devicePath)
{
return EnumerateDevices().Where(x => x.Path == devicePath).Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, params int[] productIds)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productIds.Contains(x.Attributes.ProductId));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, int productId, ushort UsagePage)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
}
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
{
var devices = new List<DeviceInfo>();
var hidClass = HidClassGuid;
var deviceInfoSet = NativeMethods.SetupDiGetClassDevs(ref hidClass, null, hwndParent: IntPtr.Zero, NativeMethods.DIGCF_PRESENT | NativeMethods.DIGCF_DEVICEINTERFACE);
if (deviceInfoSet.ToInt64() != NativeMethods.INVALID_HANDLE_VALUE)
{
var deviceInfoData = CreateDeviceInfoData();
var deviceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInfo(deviceInfoSet, deviceIndex, ref deviceInfoData))
{
deviceIndex += 1;
var deviceInterfaceData = new NativeMethods.SP_DEVICE_INTERFACE_DATA();
deviceInterfaceData.cbSize = Marshal.SizeOf(deviceInterfaceData);
var deviceInterfaceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInterfaces(deviceInfoSet, ref deviceInfoData, ref hidClass, deviceInterfaceIndex, ref deviceInterfaceData))
{
deviceInterfaceIndex++;
var devicePath = GetDevicePath(deviceInfoSet, deviceInterfaceData);
var description = GetBusReportedDeviceDescription(deviceInfoSet, ref deviceInfoData) ??
GetDeviceDescription(deviceInfoSet, ref deviceInfoData);
devices.Add(new DeviceInfo { Path = devicePath, Description = description });
}
}
NativeMethods.SetupDiDestroyDeviceInfoList(deviceInfoSet);
}
return devices;
}
private static NativeMethods.SP_DEVINFO_DATA CreateDeviceInfoData()
{
var deviceInfoData = new NativeMethods.SP_DEVINFO_DATA();
deviceInfoData.cbSize = Marshal.SizeOf(deviceInfoData);
deviceInfoData.DevInst = 0;
deviceInfoData.ClassGuid = Guid.Empty;
deviceInfoData.Reserved = IntPtr.Zero;
return deviceInfoData;
}
private static string GetDevicePath(IntPtr deviceInfoSet, NativeMethods.SP_DEVICE_INTERFACE_DATA deviceInterfaceData)
{
var bufferSize = 0;
var interfaceDetail = new NativeMethods.SP_DEVICE_INTERFACE_DETAIL_DATA { Size = IntPtr.Size == 4 ? 4 + Marshal.SystemDefaultCharSize : 8 };
NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, IntPtr.Zero, 0, ref bufferSize, IntPtr.Zero);
return NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, ref interfaceDetail, bufferSize, ref bufferSize, IntPtr.Zero) ?
interfaceDetail.DevicePath : null;
}
private static Guid HidClassGuid
{
get
{
if (_hidClassGuid.Equals(Guid.Empty)) NativeMethods.HidD_GetHidGuid(ref _hidClassGuid);
return _hidClassGuid;
}
}
private static string GetDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
var requiredSize = 0;
var type = 0;
if (NativeMethods.SetupDiGetDeviceRegistryProperty(deviceInfoSet,
ref devinfoData,
NativeMethods.SPDRP_DEVICEDESC,
ref type,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize))
{
return new string(descriptionBuffer);
}
return null;
}
}
private static string GetBusReportedDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
if (Environment.OSVersion.Version.Major > 5)
{
uint propertyType = 0;
var requiredSize = 0;
if (NativeMethods.SetupDiGetDeviceProperty(deviceInfoSet,
ref devinfoData,
ref NativeMethods.DEVPKEY_Device_BusReportedDeviceDesc,
ref propertyType,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize,
0))
{
return new string(descriptionBuffer);
}
}
return null;
}
}
}
}
<MSG> Add Override to Enumerate by UsagePage (#124)
Simple override to select all devices that match the usage page. This is useful if the machine may have multiple hid devices in the same class, or if trying to find a specific type of device without knowing the specifics of vendor or product id.
<DFF> @@ -41,11 +41,17 @@ namespace HidLibrary
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
}
+
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
+ public static IEnumerable<HidDevice> Enumerate(ushort UsagePage)
+ {
+ return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => (ushort)x.Capabilities.UsagePage == UsagePage);
+ }
+
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
| 6 | Add Override to Enumerate by UsagePage (#124) | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
898 | <NME> HidDevices.cs
<BEF> using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Runtime.InteropServices;
namespace HidLibrary
{
public class HidDevices
{
private static Guid _hidClassGuid = Guid.Empty;
public static bool IsConnected(string devicePath)
{
return EnumerateDevices().Any(x => x.Path == devicePath);
}
public static HidDevice GetDevice(string devicePath)
{
return Enumerate(devicePath).FirstOrDefault();
}
public static IEnumerable<HidDevice> Enumerate()
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(string devicePath)
{
return EnumerateDevices().Where(x => x.Path == devicePath).Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, params int[] productIds)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productIds.Contains(x.Attributes.ProductId));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, int productId, ushort UsagePage)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
}
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
{
var devices = new List<DeviceInfo>();
var hidClass = HidClassGuid;
var deviceInfoSet = NativeMethods.SetupDiGetClassDevs(ref hidClass, null, hwndParent: IntPtr.Zero, NativeMethods.DIGCF_PRESENT | NativeMethods.DIGCF_DEVICEINTERFACE);
if (deviceInfoSet.ToInt64() != NativeMethods.INVALID_HANDLE_VALUE)
{
var deviceInfoData = CreateDeviceInfoData();
var deviceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInfo(deviceInfoSet, deviceIndex, ref deviceInfoData))
{
deviceIndex += 1;
var deviceInterfaceData = new NativeMethods.SP_DEVICE_INTERFACE_DATA();
deviceInterfaceData.cbSize = Marshal.SizeOf(deviceInterfaceData);
var deviceInterfaceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInterfaces(deviceInfoSet, ref deviceInfoData, ref hidClass, deviceInterfaceIndex, ref deviceInterfaceData))
{
deviceInterfaceIndex++;
var devicePath = GetDevicePath(deviceInfoSet, deviceInterfaceData);
var description = GetBusReportedDeviceDescription(deviceInfoSet, ref deviceInfoData) ??
GetDeviceDescription(deviceInfoSet, ref deviceInfoData);
devices.Add(new DeviceInfo { Path = devicePath, Description = description });
}
}
NativeMethods.SetupDiDestroyDeviceInfoList(deviceInfoSet);
}
return devices;
}
private static NativeMethods.SP_DEVINFO_DATA CreateDeviceInfoData()
{
var deviceInfoData = new NativeMethods.SP_DEVINFO_DATA();
deviceInfoData.cbSize = Marshal.SizeOf(deviceInfoData);
deviceInfoData.DevInst = 0;
deviceInfoData.ClassGuid = Guid.Empty;
deviceInfoData.Reserved = IntPtr.Zero;
return deviceInfoData;
}
private static string GetDevicePath(IntPtr deviceInfoSet, NativeMethods.SP_DEVICE_INTERFACE_DATA deviceInterfaceData)
{
var bufferSize = 0;
var interfaceDetail = new NativeMethods.SP_DEVICE_INTERFACE_DETAIL_DATA { Size = IntPtr.Size == 4 ? 4 + Marshal.SystemDefaultCharSize : 8 };
NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, IntPtr.Zero, 0, ref bufferSize, IntPtr.Zero);
return NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, ref interfaceDetail, bufferSize, ref bufferSize, IntPtr.Zero) ?
interfaceDetail.DevicePath : null;
}
private static Guid HidClassGuid
{
get
{
if (_hidClassGuid.Equals(Guid.Empty)) NativeMethods.HidD_GetHidGuid(ref _hidClassGuid);
return _hidClassGuid;
}
}
private static string GetDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
var requiredSize = 0;
var type = 0;
if (NativeMethods.SetupDiGetDeviceRegistryProperty(deviceInfoSet,
ref devinfoData,
NativeMethods.SPDRP_DEVICEDESC,
ref type,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize))
{
return new string(descriptionBuffer);
}
return null;
}
}
private static string GetBusReportedDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
if (Environment.OSVersion.Version.Major > 5)
{
uint propertyType = 0;
var requiredSize = 0;
if (NativeMethods.SetupDiGetDeviceProperty(deviceInfoSet,
ref devinfoData,
ref NativeMethods.DEVPKEY_Device_BusReportedDeviceDesc,
ref propertyType,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize,
0))
{
return new string(descriptionBuffer);
}
}
return null;
}
}
}
}
<MSG> Add Override to Enumerate by UsagePage (#124)
Simple override to select all devices that match the usage page. This is useful if the machine may have multiple hid devices in the same class, or if trying to find a specific type of device without knowing the specifics of vendor or product id.
<DFF> @@ -41,11 +41,17 @@ namespace HidLibrary
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
}
+
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
+ public static IEnumerable<HidDevice> Enumerate(ushort UsagePage)
+ {
+ return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => (ushort)x.Capabilities.UsagePage == UsagePage);
+ }
+
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
| 6 | Add Override to Enumerate by UsagePage (#124) | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
899 | <NME> StructLog4J.java
<BEF> package com.github.structlog4j;
import lombok.experimental.UtilityClass;
import java.util.Optional;
import java.util.function.Function;
/**
* Common settings
*
* @author Jacek Furmakiewicz
*/
@UtilityClass // Lombok
public class StructLog4J {
static final String VALUE_NULL = "null";
private IFormatter formatter = KeyValuePairFormatter.getInstance();
private Optional<IToLog> mandatoryContextSupplier = Optional.empty();
// default formatter just does a toString(), regardless of object type
private Function<Object,String> defaultValueFormatter = (value) -> value == null ? VALUE_NULL : value.toString();
private Function<Object,String> valueFormatter = defaultValueFormatter;
/**
* Allows to override the default formatter. Should be only done once during application startup
* from the main thread to avoid any concurrency issues
super.get().setLength(0);
return super.get();
}
FunctionalInterface t;
};
/**
/**
* Allows to pas in a lambda that will be invoked on every log entry to add additional mandatory
* key/value pairs (e.g. hostname, service name, etc). Saves the hassle of having to specify it explicitly
* on every log invocation
*
* @param mandatoryContextSupplier Lambda that will executed on every log entry.
*/
public void setMandatoryContextSupplier(IToLog mandatoryContextSupplier) {
StructLog4J.mandatoryContextSupplier = Optional.of(mandatoryContextSupplier);
}
/**
* ALlows to define a lambda that can perform custom formatting of any object that is passed in as a value
* to any key/value entry
*
* @param formatter Formatter lambda
*/
public void setValueFormatter(Function<Object,String> formatter) {
if (formatter != null) {
valueFormatter = formatter;
} else {
throw new RuntimeException("Value formatter cannot be null");
}
}
/**
* Gets current log formatter
*/
public IFormatter getFormatter() {
return formatter;
}
/**
* Gets optional mandatory context supplier
*/
public Optional<IToLog> getMandatoryContextSupplier() {
return mandatoryContextSupplier;
}
/**
* Clears the mandatory context supplier (usually for testing purposes only)
*/
public void clearMandatoryContextSupplier() {
mandatoryContextSupplier = Optional.empty();
}
/**
* Returns the value formatter
*/
public Function<Object,String> getValueFormatter() {
return valueFormatter;
}
/**
* Internal usage for formatting purposes
*/
public boolean isPrimitiveOrNumber(Class<?> valueType) {
return valueType.isPrimitive() ||
Boolean.class.equals(valueType) ||
Short.class.equals(valueType) ||
Integer.class.equals(valueType) ||
Long.class.equals(valueType) ||
Double.class.equals(valueType) ||
Float.class.equals(valueType);
}
}
<MSG> cleanup
<DFF> @@ -29,8 +29,6 @@ public class StructLog4J {
super.get().setLength(0);
return super.get();
}
-
- FunctionalInterface t;
};
/**
| 0 | cleanup | 2 | .java | java | mit | jacek99/structlog4j |
Subsets and Splits